How to Edit a PDF Programmatically with C#
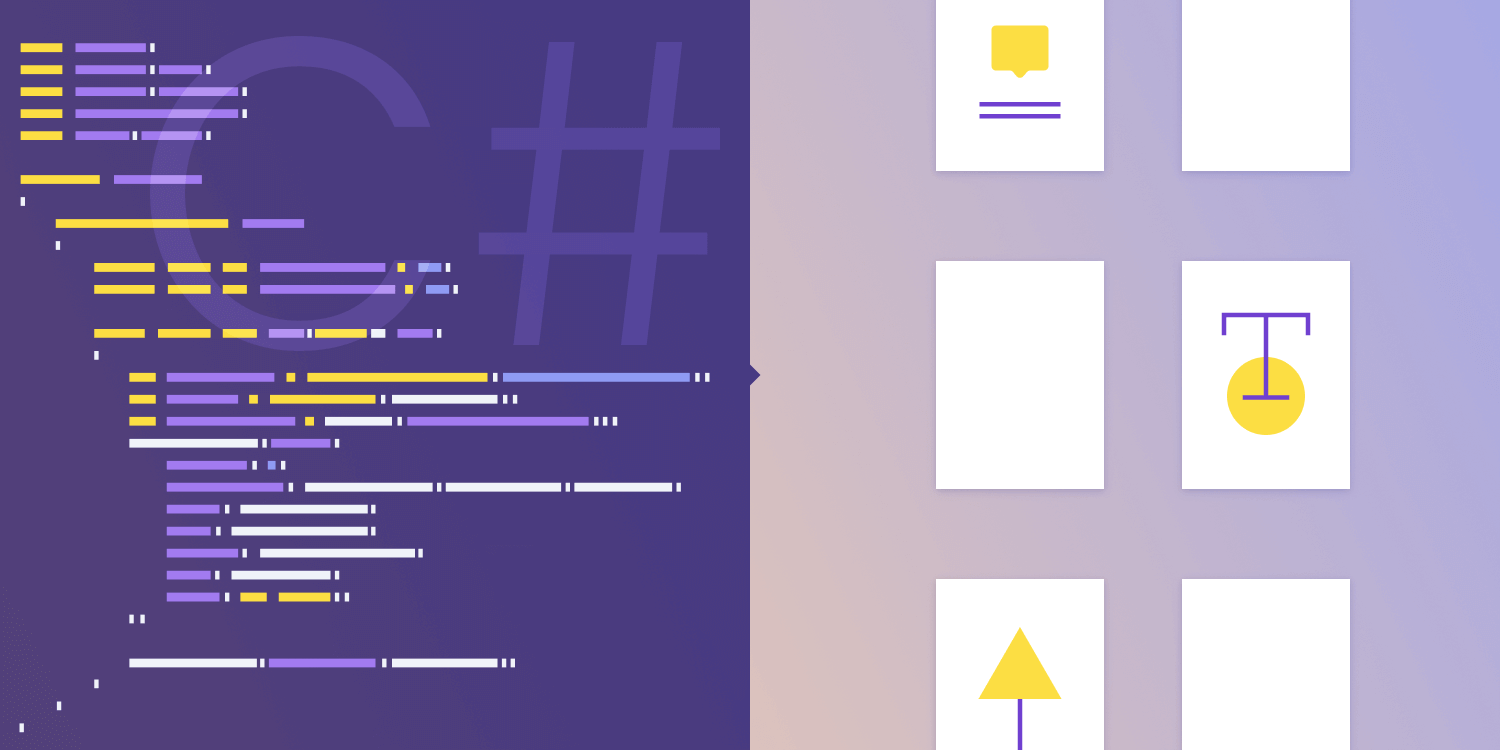
In this blog post, we’ll demonstrate how to edit a PDF document programmatically using C#. After reading it, you’ll know how to add annotations, add new pages, and change the pages of a PDF.
Setting Up
For this tutorial, we’ll use the PSPDFKit Library for .NET in a .NET 5 project. If your project is using a different version of .NET, don’t worry; the PSPDFKit Library for .NET is a .NET Standard 2.0 library, which means it can be used in a wide variety of different .NET project types.
Once you have your project in place, you need to add a reference to the PSPDFKit NuGet package. You can do so by using the Visual Studio package manager UI, or by using the .NET CLI to run the following command:
dotnet add package PSPDFKit.NET --version 1.4.0
💡 Tip: If you need more information about how to integrate PSPDFKit into your specific flow, please refer to our integration guide.
It’s also important to note that before you call any PSPDFKit APIs, you need to initialize the SDK. If you don’t have a license and are currently evaluating the product, you can call Sdk.InitializeTrial
without the need for a license key. If you already have a license, call Sdk.Initialize
instead, providing your license key as an argument.
Loading Documents
To load a document, the PSPDFKit library uses the concept of data providers. For this tutorial, we’ll focus on the data provider responsible for reading files on disk — the FileDataProvider
— but the API is extensible should you have a more specific need!
Here’s an example of how to load a document from a file:
using PSPDFKit; using PSPDFKit.Providers; namespace PdfEditing { public sealed class Program { public static void Main(string[] args) { Sdk.InitializeTrial(); var fileProvider = new FileDataProvider("Assets/document.pdf"); var document = new Document(fileProvider); // After loading the document, you can start editing it. } } }
open PSPDFKit open PSPDFKit.Providers [<EntryPoint>] let main argv = let fileProvider = new FileDataProvider("Assets/document.pdf") let document = new Document(fileProvider) // TODO: Edit the document! 0
Adding Annotations
PSPDFKit for .NET provides a JSON-based API for describing PDF annotations. This allows for a lot of flexibility when dealing with annotations, since it means you can have handcrafted annotations in your code, or you can have a JSON file you load when needed.
The example below shows how to add a text annotation to the PDF we opened in the previous section:
using Newtonsoft.Json.Linq; using PSPDFKit; using PSPDFKit.Providers; namespace PdfEditing { public sealed class Program { public static void Main(string[] args) { var fileProvider = new FileDataProvider("Assets/document.pdf"); var document = new Document(fileProvider); var annotationProvider = document.GetAnnotationProvider(); var textAnnotationJson = new JObject { { "text", "Hello from PSPDFKit" }, { "bbox", new JArray(10, 10, 400, 400) }, { "creatorName", "Will" }, { "type", "pspdfkit/text" }, { "updatedAt", "2021-01-01T00:00:00Z" }, { "v", 1 } }; annotationProvider.AddAnnotationJson(textAnnotationJson); document.Save(new DocumentSaveOptions()) } } }
open Newtonsoft.Json.Linq open PSPDFKit open PSPDFKit.Providers [<EntryPoint>] let main argv = let fileProvider = new FileDataProvider("Assets/document.pdf") let document = new Document(fileProvider) let annotationProvider = document.GetAnnotationProvider() let textAnnotationJson = new JObject() textAnnotationJson.Add("text", JObject("Hello from PSPDFKit")) textAnnotationJson.Add("bbox", JObject(new JArray(10, 10, 400, 400))) textAnnotationJson.Add("creatorName", JObject("Will")) textAnnotationJson.Add("type", JObject("pspdfkit/text")) textAnnotationJson.Add("updatedAt", JObject("2021-01-01T00:00:00Z")) textAnnotationJson.Add("v", JObject(1)) annotationProvider.AddAnnotationJson(textAnnotationJson) document.Save(new DocumentSaveOptions()) 0
Note that we’re also calling the Save
method. This method ensures that the changes made will be saved to the data provider used to load the document. In other words, it ensures that our file will be saved with all the up-to-date changes!
💡 Tip: For more details on the types of annotations and all valid properties of annotations, refer to the annotations guide.
Manipulating Pages
Next, we’ll add a page to the beginning of our document. This can be useful for reports that need a dynamically generated cover page.
To achieve this, we’ll use the DocumentEditor
API, a powerful API that allows us to manipulate documents by adding/removing/modifying pages. In the example below, we’re adding a new page at the beginning of the document:
using PSPDFKit; using PSPDFKit.Basic; using PSPDFKit.Providers; using PSPDFKitFoundation; using System.Drawing; namespace PdfEditing { public sealed class Program { private const int CoverPageWidth = 200; private const int CoverPageHeight = 200; public static void Main(string[] args) { var fileProvider = new FileDataProvider("Assets/document.pdf"); var document = new Document(fileProvider); var documentEditor = document.CreateDocumentEditor(); documentEditor.AddPage( pageIndex: 0, indexPosition: DocumentEditor.IndexPosition.BeforeIndex, height: CoverPageWidth, width: CoverPageHeight, rotation: Rotation.Degrees0, color: Color.White, insets: new Insets() ); documentEditor.SaveDocument(fileProvider); } } }
open PSPDFKit open PSPDFKit.Basic open PSPDFKit.Providers open PSPDFKitFoundation open System.Drawing let coverPageWidth = 200 let coverPageHeight = 200 [<EntryPoint>] let main argv = let fileProvider = FileDataProvider("Assets/document.pdf") let document = Document(fileProvider) let documentEditor = document.CreateDocumentEditor() documentEditor.AddPage( pageIndex = 0, indexPosition = DocumentEditor.IndexPosition.BeforeIndex, height = coverPageWidth, width = coverPageHeight, rotation = Rotation.Degrees0, color = Color.White, insets = Insets() ) documentEditor.SaveDocument(fileProvider); 0
💡 Tip: I’m using the
SaveDocument
method by passing it the samefileProvider
we used to load the document. This effectively means we’re saving over the existing document, but you can use a different provider to save the modified document in a different file instead.
With the same DocumentEditor
, we can also change the order of pages using the MovePages
method, and we can remove pages we no longer need using the RemovePages
method.
Conclusion
The nuggets of knowledge found in this tutorial are a foundation for what you can achieve when working with PDFs. By combining annotation editing with page manipulation, you can create gorgeous custom reports with different cover pages and customized content based on whatever logic your program possesses. If you’re looking for an example using Java, you can check out Programmatically Editing a PDF Using Java.
The PSPDFKit Library for .NET offers an easy-to-use yet very powerful API for manipulating PDFs. Getting started with it is simple, and what we show here is just a small taste of all the features the SDK contains!
FAQ
What is PSPDFKit used for?
PSPDFKit is a powerful library for working with PDF documents, allowing you to view, edit, and annotate PDFs programmatically.
How can I load a PDF document in C#?
You can load a PDF document using the FileDataProvider
class provided by the PSPDFKit library.
What types of annotations can I add to a PDF?
You can add various types of annotations, such as text, highlights, and shapes, using JSON-based APIs in PSPDFKit.
Is it possible to manipulate pages in a PDF?
Yes, PSPDFKit allows you to add, remove, and rearrange pages in a PDF document easily.
Do I need a license to use PSPDFKit?
You can evaluate PSPDFKit using a trial version, but a license is required for production use.