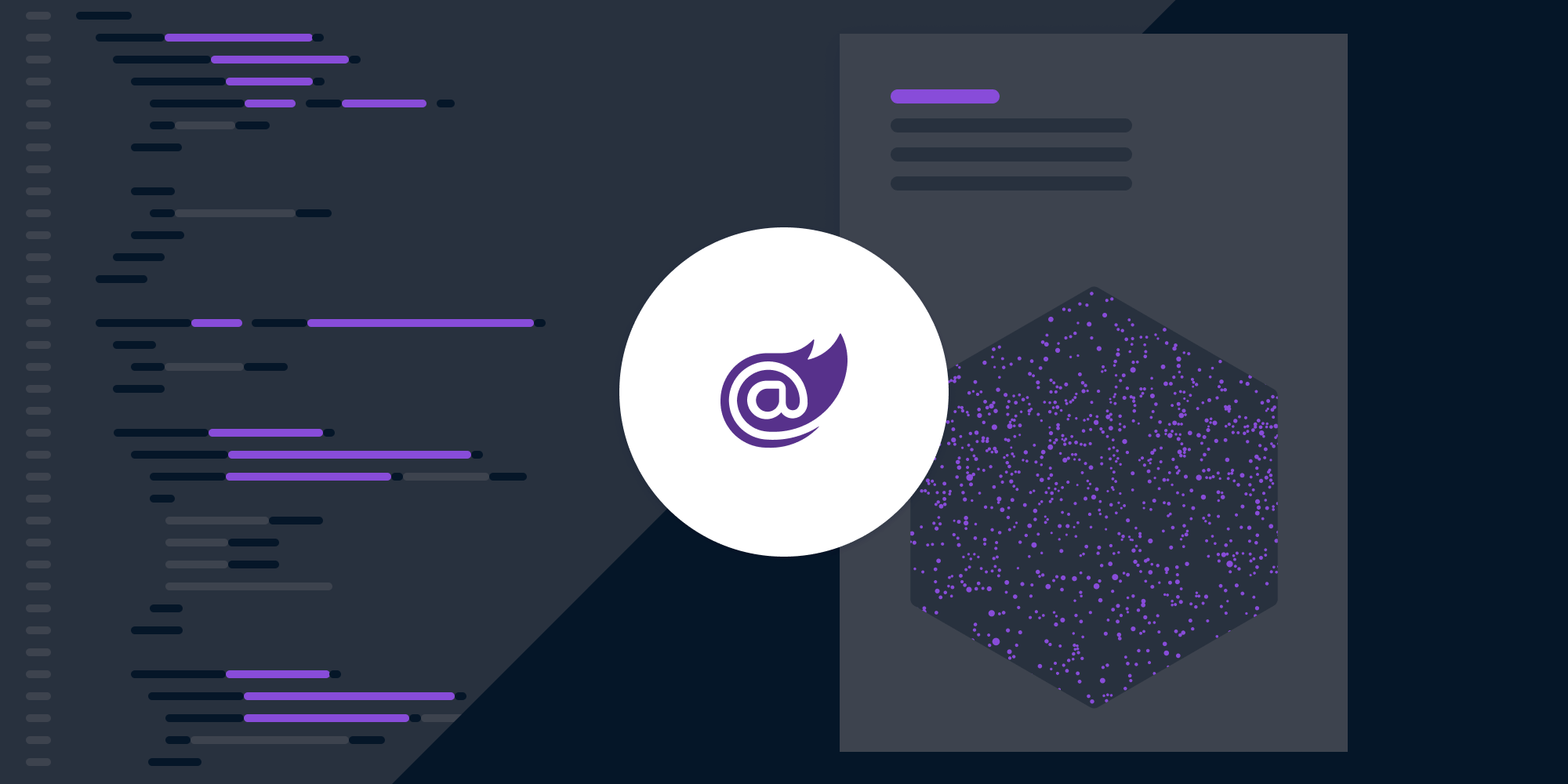
Blazor Server applications offer a dynamic way to work with web technologies using C# and .NET. In this blog post, we’ll demonstrate how to generate a simple PDF in a Blazor Server application using the PDFsharp library. Additionally, you’ll learn about Nutrient’s .NET PDF library, which offers more advanced features for complex .NET projects.
PDFsharp is a .NET library for processing PDF files, and it can be integrated into a Blazor project for this purpose.
Prerequisites
Step 1 — Setting up the Blazor Server project
Open Visual Studio Code and create a new directory for your project. In your terminal, navigate to the project directory and run the following command to create a Blazor Server project:
dotnet new blazorserver -n GeneratePDFBlazor
After the project is created, open the project folder in Visual Studio Code:
code GeneratePDFBlazor
Next, restore the project dependencies:
dotnet restore
Your project must be .NET 6 or higher, as the .NET Framework is no longer supported.
Step 2 — Installing the PDFsharp package
Now, install the PDFsharp package using the .NET CLI:
dotnet add package PDFsharp --version 6.0.0
Alternatively, you can add PDFsharp by modifying the GeneratePDFBlazor.csproj
file. Add the following under the <ItemGroup>
section:
<PackageReference Include="PDFsharp" Version="6.0.0" />
Then run:
dotnet restore
Step 3 — Setting up the PDF generator
Now, create a new class named PdfGenerator.cs
in the GeneratePDFBlazor
project folder. This class will handle the creation of the PDF document using PDFsharp. In Visual Studio Code, create a new file, PdfGenerator.cs
, and add the following code:
using PdfSharp; using PdfSharp.Pdf; using PdfSharp.Drawing; using PdfSharp.Fonts; using PdfSharp.Snippets.Font; public class PdfGenerator { public static byte[] CreatePdf() { using (var memoryStream = new MemoryStream()) { if (Capabilities.Build.IsCoreBuild) GlobalFontSettings.FontResolver = new FailsafeFontResolver(); PdfDocument document = new PdfDocument(); document.Info.Title = "Generated with PDFsharp"; PdfPage page = document.AddPage(); XGraphics gfx = XGraphics.FromPdfPage(page); var width = page.Width; var height = page.Height; gfx.DrawLine(XPens.Red, 0, 0, width, height); gfx.DrawLine(XPens.Red, width, 0, 0, height); var radius = width / 5; gfx.DrawEllipse(new XPen(XColors.Red, 1.5), XBrushes.White, new XRect(width / 2 - radius, height / 2 - radius, 2 * radius, 2 * radius)); XFont font = new XFont("Times New Roman", 20, XFontStyleEx.BoldItalic); gfx.DrawString("Hello, PDFsharp!", font, XBrushes.Black, new XRect(0, 0, page.Width, page.Height), XStringFormats.Center); document.Save(memoryStream); return memoryStream.ToArray(); } } }
This class generates a simple PDF with text and graphics using PDFsharp and returns it as a byte array.
Step 4 — Creating the Blazor interface
Next, you’ll create a user interface in Blazor to generate and download the PDF. Open the Pages/Index.razor
file and update it as follows:
@page "/" @inject IJSRuntime JSRuntime <h3>PDF Generator</h3> <button class="btn btn-primary" @onclick="DownloadPdf">Download PDF</button> @code { private async Task DownloadPdf() { byte[] pdfBytes = PdfGenerator.CreatePdf(); var fileName = "GeneratedPdf.pdf"; await JSRuntime.InvokeVoidAsync("saveAsFile", fileName, Convert.ToBase64String(pdfBytes)); } }
The DownloadPdf
method calls the PdfGenerator.CreatePdf
method. It then uses JavaScript interop to trigger a download in the browser.
JavaScript interop in _Host.cshtml
Finally, add the JavaScript required for downloading the PDF in the Pages/_Host.cshtml
file. Add the following script before the closing body
tag:
<script> window.saveAsFile = (filename, bytesBase64) => { var link = document.createElement('a'); link.href = 'data:application/octet-stream;base64,' + bytesBase64; link.download = filename; document.body.appendChild(link); link.click(); document.body.removeChild(link); }; </script>
This script creates a temporary link element, sets its href
to the base64
-encoded PDF data, and triggers a click to start the download.
Step 5 — Running the application
In Visual Studio Code, open the terminal and run the following command:
dotnet run
By default, the application may run on a different port (e.g. http://localhost:5138). You can access the application by going to http://localhost:5138 in your browser.
You’ll see the interface you created, and clicking the Download PDF button will generate and download the PDF.
Step 6 — Interacting with the application
Interact with your application in the browser. Click the button (or perform the action you set up) to trigger the PDF generation. The PDF will be generated and then downloaded by the browser.
The PDFsharp library offers a wide range of capabilities for creating complex PDFs, while Blazor and JavaScript interop make it easy to deliver these documents to your users. This basic setup can be expanded and customized to meet the specific needs of your application.
![]()
You can find the full source code for this tutorial in our GitHub repository.
Nutrient .NET SDK
While both PDFsharp and the Nutrient .NET SDK offer PDF generation capabilities for .NET applications, Nutrient .NET SDK stands out as a more comprehensive and feature-rich solution. Here are some reasons why Nutrient .NET SDK can be considered better:
-
Extensive capabilities — Nutrient .NET SDK provides a wide range of functionalities, including OCR, barcode processing, PDF editing, document conversion, scanning, and image processing. It outperforms many other .NET document imaging technologies available on the market.
-
OCR (optical character recognition) — Nutrient .NET SDK supports OCR, which enables the extraction of text from scanned documents and images. This feature is essential for applications that require text recognition and data extraction from PDFs.
-
Advanced PDF editing — Nutrient .NET SDK offers more advanced PDF editing capabilities, such as editing text, images, and annotations, as well as adding watermarks, digital signatures, and form fields to PDF documents.
-
Comprehensive support — Nutrient has a reputation for providing excellent customer support and regular updates to address any issues or introduce new features. This ensures that your application remains up to date and well-maintained.
-
Integration-friendly — Nutrient .NET SDK is designed to seamlessly integrate into your existing .NET applications, making it easier to adopt and implement in your projects.
-
Documentation and resources — Nutrient offers comprehensive documentation, tutorials, and sample code, making it easier for developers to get started and leverage the library’s full potential.
To get started with the Nutrient .NET SDK, follow our getting started guide.
Conclusion
This tutorial demonstrated how to use the PDFsharp library for basic PDF generation in Blazor Server applications. PDFsharp is an excellent open source tool for creating and manipulating PDFs in .NET, ideal for straightforward tasks like drawing text and graphics in documents.
For more advanced features, however, consider the Nutrient .NET SDK library. It extends capabilities to include OCR, PDF editing, barcode processing, and document conversion. This makes it a comprehensive solution for complex Blazor projects that require robust document processing functionalities. Whether you need simplicity with PDFsharp or advanced features with GdPicture.NET, both libraries offer powerful ways to handle PDFs in Blazor applications.
To see a list of all frameworks, you can contact our Sales team. Or, launch our demo to see our viewer in action.