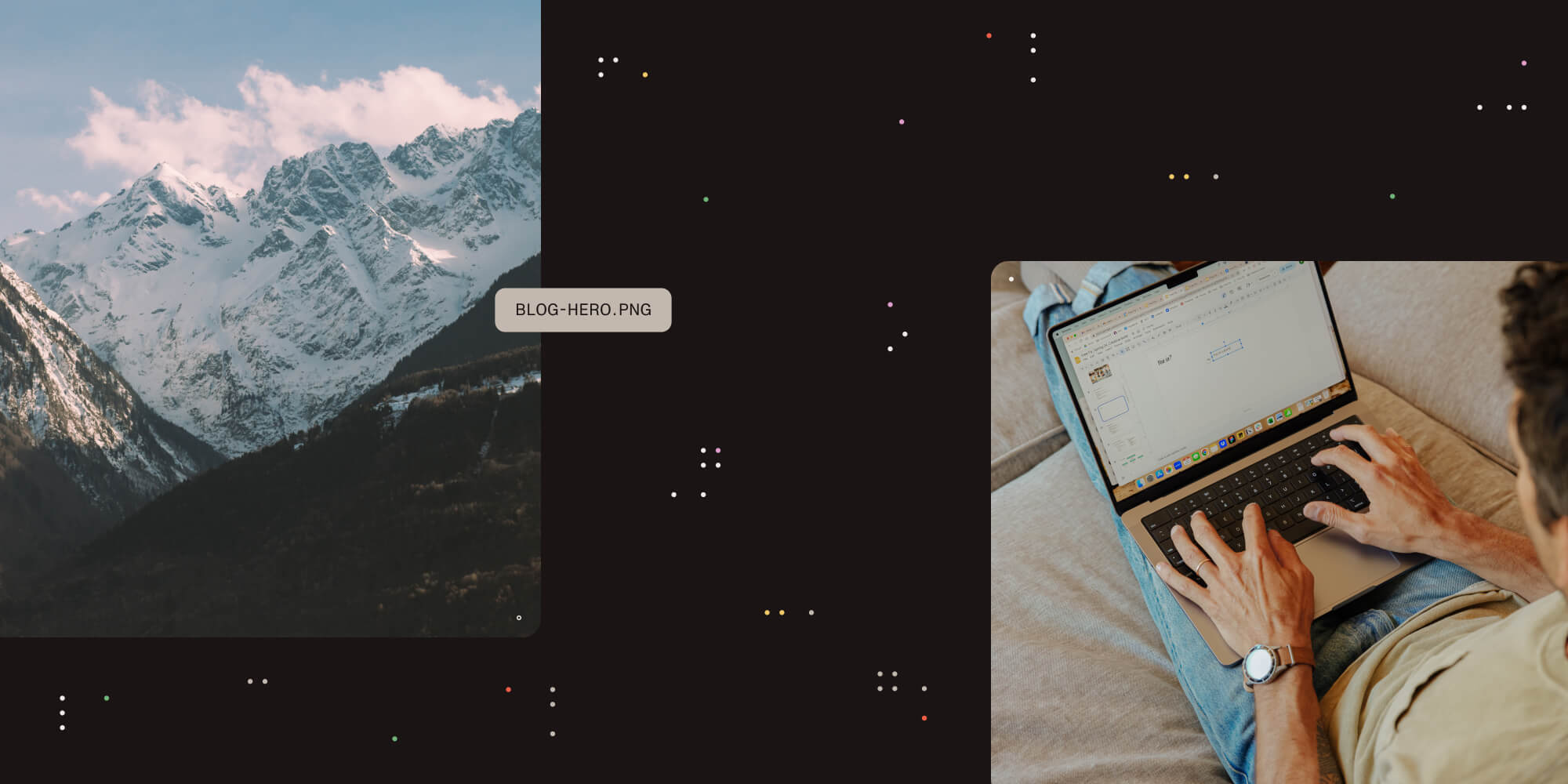
In this article we explain how to use Java and server based Optical Character Recognition (OCR) to convert image based files such as TIFF, PNG and scanned PDFs into fully searchable and indexable PDF files. Read on to learn about how - in addition to Document Conversion, Merging, Watermarking, Splitting and Securing of documents - the latest version of the Muhimbi PDF Converter Services also comes with a comprehensive OCR engine.
A .NET (C#) version of this article is available here.
For those not familiar with the product, the Muhimbi PDF Converter Services is a server based SDK that allows software developers to convert and manipulate typical Office files, including MS-Word, Excel, PowerPoint, Visio, Publisher, AutoCAD, HTML, emails and InfoPath, to PDF and other formats using a robust, scalable but friendly Web Services interface from Java, PHP, Ruby and .NET based solutions. If you have any questions or need more information then please let us know.
Please read this post for a high level overview of our new OCR facilities.
Sample code
The following sample illustrates how to use OCR to convert a file (preferably a scan) into a fully searchable PDF. In this example we use wsimport to generate web service proxy classes, but other web service frameworks are supported as well. We actually prefer Apache Axis2 ( See this sample) as it generates cleaner and more flexible code.
The example described below assumes the following:
-
The JDK has been installed and configured.
-
The Conversion Service and all prerequisites have been installed in line with the Administration Guide.
-
The Conversion Service is running in the default anonymous mode. This is not an absolute requirement, but it makes initial experimentation much easier.
As of version 7.1 this sample code is automatically installed alongside the product. The source code, including pre-generated proxy classes for the web service and a sample file, can be downloaded here. If you choose not to download the sample code then please carry out the steps described below.
The first step is to generate proxy classes for the web service by executing the following command:
wsimport https://localhost:41734/Muhimbi.DocumentConverter.WebService/?wsdl
-d src -Xnocompile -p com.muhimbi.ws
Feel free to change the package name and destination directory to something more suitable for your organisation. If you are generating proxies from a remote system (a system that doesn’t run the Muhimbi Conversion Service) then please see this Knowledge Base Article.
Wsimport automatically generates the Java class names. Unfortunately some of the generated names are rather long and ugly so you may want to consider renaming some, particularly the Exception classes, to something friendlier. This, however, means that if you ever run wsimport again you will need to re-apply those changes. For more information have a look at the high level overview of the Object Model exposed by the web service.
Once the proxy classes have been created add the following sample code to your project. Run the code and make sure the file to OCR is specified on the command line.
`package com.muhimbi.app; import com.muhimbi.ws.*; import java.io.*; import java.net.URL; import java.util.List; import javax.xml.bind.JAXBElement; import javax.xml.namespace.QName; public class WsClient { private final static String DOCUMENTCONVERTERSERVICE_WSDL_LOCATION = "https://localhost:41734/Muhimbi.DocumentConverter.WebService/?wsdl"; private static ObjectFactory _objectFactory = new ObjectFactory(); public static void main(String[] args) { try { if (args.length != 1) { System.out.println("Please specify a single file name on the command line."); } else { // ** Process command line parameters String sourceDocumentPath = args[0]; File file = new File(sourceDocumentPath); String fileName = getFileName(file); String fileExt = getFileExtension(file); System.out.println("Processing file " + sourceDocumentPath); // ** Initialise Web Service DocumentConverterService_Service dcss = new DocumentConverterService_Service( new URL(DOCUMENTCONVERTERSERVICE_WSDL_LOCATION), new QName("https://tempuri.org/", "DocumentConverterService")); DocumentConverterService dcs = dcss.getBasicHttpBindingDocumentConverterService(); // ** Only call conversion if file extension is supported if (isFileExtensionSupported(fileExt, dcs)) { // ** Read source file from disk byte[] fileContent = readFile(sourceDocumentPath); // ** Converting the file OpenOptions openOptions = getOpenOptions(fileName, fileExt); ConversionSettings conversionSettings = getConversionSettings(); byte[] convertedFile = dcs.convert(fileContent, openOptions, conversionSettings); // ** Writing converted file to file system String destinationDocumentPath = getPDFDocumentPath(file); writeFile(convertedFile, destinationDocumentPath); System.out.println("File converted successfully to " + destinationDocumentPath); } else { System.out.println("The file extension is not supported."); } } } catch (IOException e) { System.out.println(e.getMessage()); } catch (DocumentConverterServiceGetConfigurationWebServiceFaultExceptionFaultFaultMessage e) { printException(e.getFaultInfo()); } catch (DocumentConverterServiceConvertWebServiceFaultExceptionFaultFaultMessage e) { printException(e.getFaultInfo()); } } public static OpenOptions getOpenOptions(String fileName, String fileExtension) { OpenOptions openOptions = new OpenOptions(); // ** Set the minimum required open options. Additional options are available openOptions.setOriginalFileName(_objectFactory.createOpenOptionsOriginalFileName(fileName)); openOptions.setFileExtension(_objectFactory.createOpenOptionsFileExtension(fileExtension)); return openOptions; } public static ConversionSettings getConversionSettings() { ConversionSettings conversionSettings = new ConversionSettings(); // ** Set the minimum required conversion settings. Additional settings are available conversionSettings.setQuality(ConversionQuality.OPTIMIZE_FOR_PRINT); conversionSettings.setRange(ConversionRange.ALL_DOCUMENTS); conversionSettings.getFidelity().add("Full"); conversionSettings.setFormat(OutputFormat.PDF); conversionSettings.setOCRSettings(_objectFactory.createConversionSettingsOCRSettings(getOCRSettings())); return conversionSettings; } public static OCRSettings getOCRSettings() { OCRSettings ocrSettings = new OCRSettings(); ocrSettings.setLanguage(_objectFactory.createOCRSettingsLanguage("eng")); ocrSettings.setPerformance(OCRPerformance.SLOW); ocrSettings.setWhiteList(_objectFactory.createOCRSettingsWhiteList("")); ocrSettings.setBlackList(_objectFactory.createOCRSettingsBlackList("")); return ocrSettings; } public static String getFileName(File file) { String fileName = file.getName(); return fileName.substring(0, fileName.lastIndexOf('.')); } public static String getFileExtension(File file) { String fileName = file.getName(); return fileName.substring(fileName.lastIndexOf('.') + 1, fileName.length()); } public static String getPDFDocumentPath(File file) { String fileName = getFileName(file); String folder = file.getParent(); if (folder == null) { folder = new File(file.getAbsolutePath()).getParent(); } return folder + File.separatorChar + fileName + "_ocr." + OutputFormat.PDF.value(); } public static byte[] readFile(String filepath) throws IOException { File file = new File(filepath); InputStream is = new FileInputStream(file); long length = file.length(); byte[] bytes = new byte[(int) length]; int offset = 0; int numRead; while (offset < bytes.length && (numRead = is.read(bytes, offset, bytes.length - offset)) >= 0) { offset += numRead; } if (offset < bytes.length) { throw new IOException("Could not completely read file " + file.getName()); } is.close(); return bytes; } public static void writeFile(byte[] fileContent, String filepath) throws IOException { OutputStream os = new FileOutputStream(filepath); os.write(fileContent); os.close(); } public static boolean isFileExtensionSupported(String extension, DocumentConverterService dcs) throws DocumentConverterServiceGetConfigurationWebServiceFaultExceptionFaultFaultMessage { Configuration configuration = dcs.getConfiguration(); final JAXBElement<ArrayOfConverterConfiguration> converters = configuration.getConverters(); final ArrayOfConverterConfiguration ofConverterConfiguration = converters.getValue(); final List<ConverterConfiguration> cList = ofConverterConfiguration.getConverterConfiguration(); for (ConverterConfiguration cc : cList) { final List<String> supportedExtension = cc.getSupportedFileExtensions() .getValue().getString(); if (supportedExtension.contains(extension)) { return true; } } return false; } public static void printException(WebServiceFaultException serviceFaultException) { System.out.println(serviceFaultException.getExceptionType()); JAXBElement<ArrayOfstring> element = serviceFaultException.getExceptionDetails(); ArrayOfstring value = element.getValue(); for (String msg : value.getString()) { System.out.println(msg); } } }`
As all this functionality is exposed via a Web Services interface, it works equally well from .NET, PHP, Ruby and other web services enabled environments. Please note that you need the OCR and PDF/A Archiving add-on license in addition to a valid PDF Converter Services or PDF Converter for SharePoint License in order to use this functionality.
This code is merely an example of what is possible, feel free to adapt it to you own needs. The possibilities are endless.
Any questions or remarks? Leave a message in the comments below or contact us.
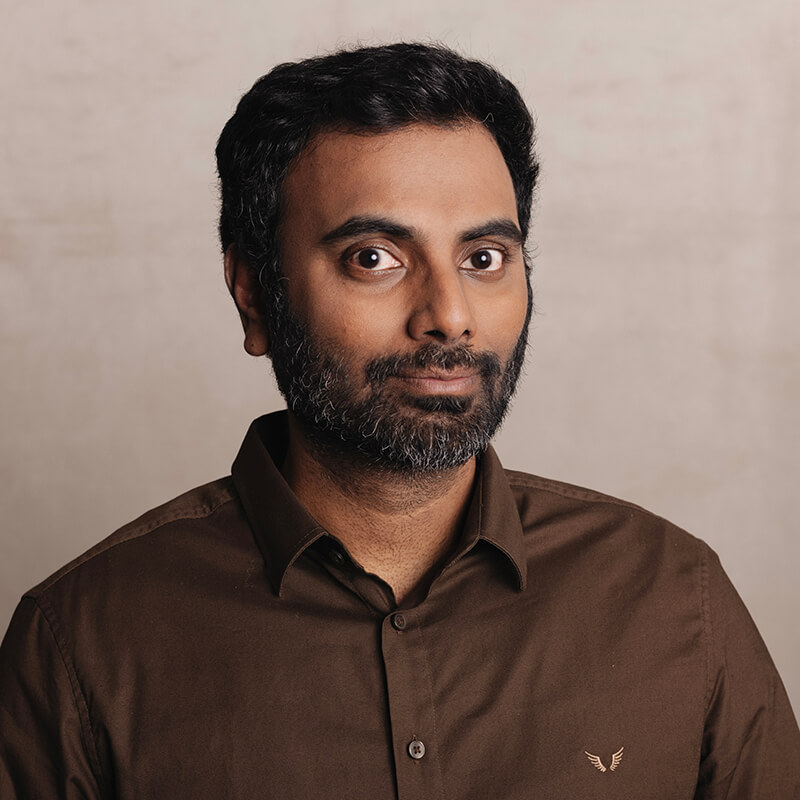
Clavin is a Microsoft Business Applications MVP who supports 1,000+ high-level enterprise customers with challenges related to PDF conversion in combination with SharePoint on-premises Office 365, Azure, Nintex, K2, and Power Platform mostly no-code solutions.