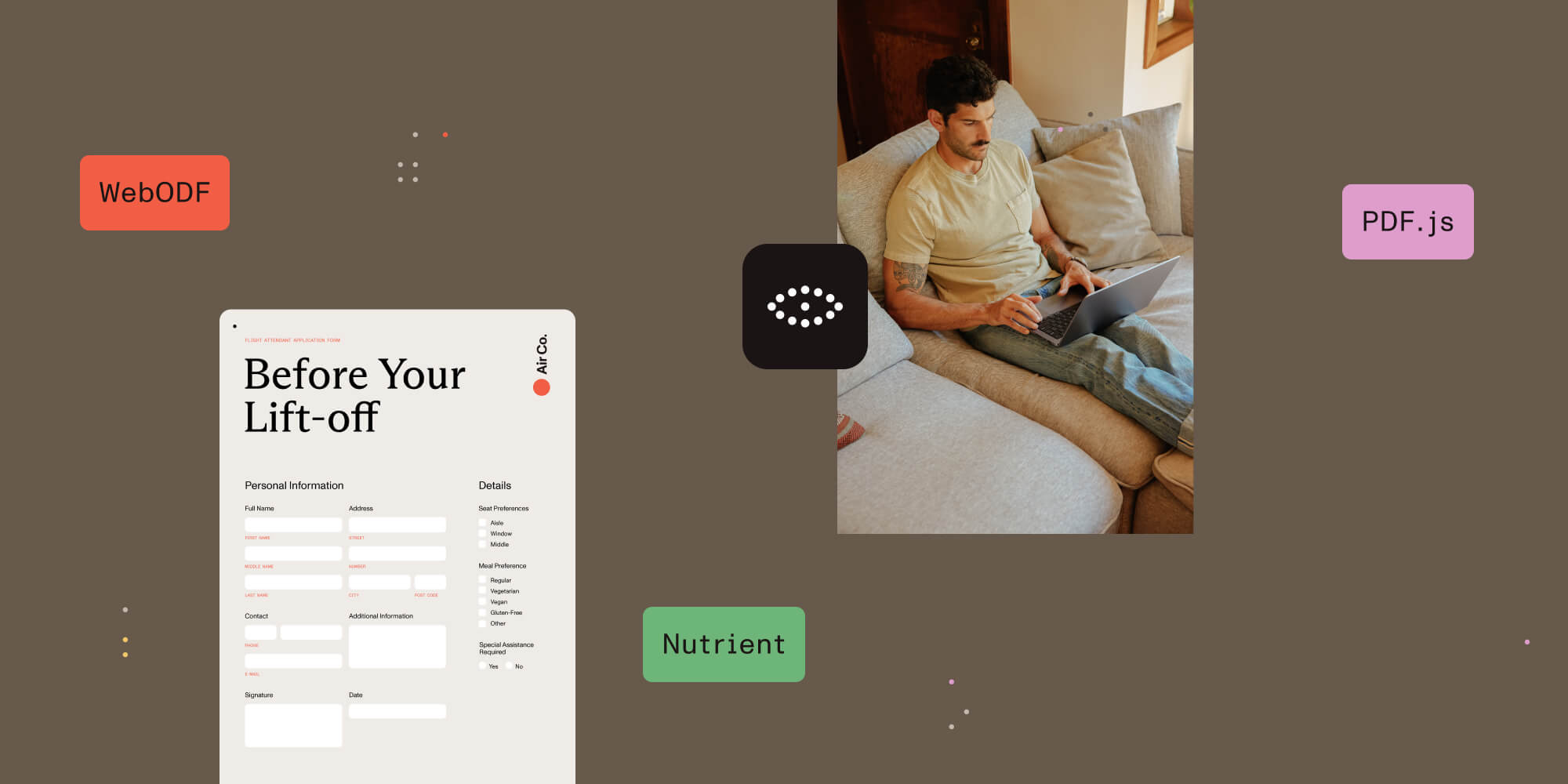
A document viewer (doc viewer) is a tool that allows users to open and display documents in various formats, such as DOCX or PDF, directly within a web or mobile application. These tools are invaluable for developers who want to offer document viewing capabilities without requiring users to download additional software like Microsoft Word.
This post will explore various document viewers that support DOCX and PDF files. It’ll provide an overview of each library, how to get started with it, and its technical features, advantages, and disadvantages.
1. WebODF
WebODF is an open source JavaScript library for rendering Open Document Format (ODF) files, such as text documents, spreadsheets, and presentations. While its primary focus is ODF, developers can extend WebODF to support DOCX.
Embedding WebODF in a webpage
To display an ODF file on your website, all you need is the webodf.js
file. Here’s a quick example of how to embed an ODF document into an HTML page:
<html> <head> <script src="webodf.js" type="text/javascript"></script> <script type="text/javascript"> function initialize() { var odfElement = document.getElementById('odf'), odfCanvas = new odf.OdfCanvas(odfElement); odfCanvas.load('document.odt'); } window.setTimeout(initialize, 0); </script> </head> <body> <div id="odf"></div> </body> </html>
Technical features
-
Natively supports ODF file formats.
-
Can be customized to support DOCX viewing.
-
Client-side rendering without server dependencies.
Advantages
-
Open source — Free to use and modify.
-
Lightweight — Simple and efficient for basic document viewing.
-
Cross-platform — Works on all modern browsers.
Disadvantages
-
Limited DOCX support — Requires custom development to support DOCX.
-
Focused on ODF — Ideal for projects requiring ODF compatibility, but less so for DOCX and PDF files.
2. PDF.js
PDF.js is a popular open source library maintained by Mozilla for rendering PDF files within web browsers. It provides a fully client-side solution for viewing and interacting with PDF documents.
How to get started
-
Installation:
Add PDF.js via a CDN or by downloading from GitHub:
<script src="https://cdnjs.cloudflare.com/ajax/libs/pdf.js/4.6.82/pdf.min.mjs"></script>
-
Basic usage:
const loadingTask = pdfjsLib.getDocument('path/to/document.pdf'); loadingTask.promise.then(function (pdf) { pdf.getPage(1).then(function (page) { const viewport = page.getViewport({ scale: 1.5 }); const canvas = document.getElementById('pdf-canvas'); const context = canvas.getContext('2d'); canvas.height = viewport.height; canvas.width = viewport.width; page.render({ canvasContext: context, viewport: viewport }); }); });
Technical features
-
Native PDF rendering — Renders PDF files directly in modern browsers without additional plugins.
-
Customizable rendering — Offers full control over how documents are rendered and how users interact with them.
-
Text extraction — Supports extracting text from PDF documents, enabling programmatic access to content.
Advantages
-
Open source — Free to use for any project, promoting community involvement.
-
Highly customizable — Provides developers with the ability to tailor the viewing experience and user interactions.
-
Active maintenance — Regularly updated and supported by Mozilla, ensuring reliability and new features.
Disadvantages
-
PDF format only — Limited to rendering PDF files; doesn’t support DOCX or other document formats.
-
Performance concerns — Rendering large or complex PDFs may lead to slower performance, especially on less powerful devices.
Check out the following blog posts to learn more about how to use PDF.js:
- How to build a React PDF viewer with PDF.js
- How to build a Vue.js PDF viewer with PDF.js
- How to build a jQuery PDF viewer with PDF.js
- How to build a Bootstrap 5 PDF viewer with PDF.js
- How to build an Electron PDF viewer with PDF.js
- How to build a TypeScript PDF viewer with PDF.js
- How to build a JavaScript PDF viewer with PDF.js
- How to build an Angular PDF viewer with PDF.js
- How to use PDF.js to highlight text programmatically
- Complete guide to PDF.js
- How to print PDFs using PDF.js
- Evaluating the render fidelity of PDF.js
- Creating a web component with PDF.js
- Implement a simple PDF viewer with PDF.js
- Rendering PDF files in the browser with PDF.js
3. Nutrient document viewer
Nutrient is a commercial solution offering comprehensive support for both DOCX and PDF viewing. It provides a robust and feature-rich viewer for developers who need enterprise-level functionality, including support for annotations, editing, and collaborative features.
How to get started
-
Installation:
Follow the installation guide to set up Nutrient. Download the library and start using it without needing a trial key.
-
Basic usage:
PSPDFKit.load({ document: '/path/to/document.docx', container: '#pspdfkit-container', });
Technical features
-
File type support — Nutrient supports multiple file types across different operational modes:
-
Standalone mode — Supports DOCX, PDF, PDF/A, XFDF, Instant JSON, PNG, JPEG, JPG, and TIFF.
-
Server-backed mode — In addition to the above, it also supports RTF, ODT, HTML, HEIC, GIF, WEBP, SVG, TGA, and EPS.
-
-
Advanced functionality — Includes features like annotations, editing, and cross-platform compatibility.
Advantages
-
Full DOCX and PDF support — Seamlessly handles both formats, alongside other common document and image formats.
-
Enterprise-grade — Reliable, fast, and customizable for large-scale projects.
-
Editing capabilities — Allows users to edit DOCX and PDF files and save changes in real time.
Disadvantages
-
Commercial license — Requires a paid license, which may make it less accessible for smaller projects, though it offers a free trial for evaluation.
To learn more about Nutrient’s document viewer, check out the following blog posts:
- How to build a TypeScript PDF viewer with Nutrient
- How to build a Nuxt.js PDF viewer with Nutrient
- How to display a PDF in React
- How to build a Tauri PDF viewer with Nutrient
- How to display PDFs using Angular
- How to build a PowerPoint (PPT/PPTX) viewer in JavaScript
- How to open Excel (XLS and XLSX) files in the browser with JavaScript
- How to build a React.js file viewer: PDF, image, MS Office
- How to open Word (DOC and DOCX) files in the browser with JavaScript
4. Nutrient Document Authoring
In addition to its viewing capabilities, Nutrient offers powerful Document Authoring, allowing developers to open, edit, and format DOCX documents. This feature integrates seamlessly with Nutrient’s viewer and offers comprehensive tools for document creation.
Explore the Nutrient Document Authoring demo
Key features
-
Open, edit, and export DOCX files, with support for rich text, tables, images, and more.
-
Export documents in both DOCX and PDF formats, ensuring perfect fidelity for print and digital use.
-
Flexible document handling with DocJSON, allowing custom workflows for document generation and modification.
-
Page-based editing for a familiar in-browser document experience.
-
Offline-first support: Edit documents entirely client-side without requiring a server.
Advantages
-
High-fidelity exports — Ensures perfect formatting when exporting to PDF or printing documents.
-
Extensive DOCX support — Directly edit DOCX files with powerful formatting and layout features.
-
Offline and client-side editing — Full offline support, allowing users to work without a server connection.
-
Easy integration — First-class TypeScript support for seamless integration into existing applications and workflows.
-
Compliance and data governance — Offers full control over data storage, simplifying the enforcement of compliance policies.
Disadvantages
-
Commercial solution — Requires a paid license. However, it offers a free trial for evaluation.
To learn more about Nutrient Document Authoring SDK, check out the following blog post:
5. ViewerJS
ViewerJS is an open source document viewer that enables users to view various document formats, including ODF and PDF, directly in their web browsers using an iframe
. It integrates the power of WebODF and PDF.js to provide robust support for these formats.
How to get started
-
Installation:
Begin by downloading ViewerJS from the official site.
-
Basic usage:
To embed a document, use the following HTML snippet:
<iframe src="/ViewerJS/#../path/to/document.odp" width="600" height="400" allowfullscreen webkitallowfullscreen ></iframe>
Technical features
-
Format support — Natively supports ODF and PDF file formats.
-
Easy embedding — Utilizes simple
iframe
tags for document display. -
Client-side operation — Requires no server-side processing, making it lightweight and efficient.
Advantages
-
Open source — Free to use and modify, promoting community collaboration.
-
Versatile format handling — Effectively manages both ODF and PDF formats.
-
Cross-browser functionality — Compatible with all major modern browsers.
Disadvantages
-
Limited functionality — Doesn’t include advanced features such as annotation or document editing.
-
DOCX support — Requires additional configuration for DOCX support, which may not be straightforward.
Conclusion
Open source libraries like PDF.js and ViewerJS provide flexible solutions for viewing PDFs and ODF documents in web applications. However, if you need robust DOCX support and enterprise-grade features, Nutrient is an excellent option for both document viewing and authoring. Its comprehensive suite of tools allows developers to create, edit, and view DOCX and PDF files seamlessly.
Choosing the right document viewer depends on the scale and needs of your project. Free options may suffice for basic document viewing, but commercial solutions like Nutrient offer superior performance, customization, and feature sets for large-scale applications.
To learn more about Nutrient’s document viewing and authoring capabilities, reach out to our Sales team. If you have any questions or need assistance, don’t hesitate to contact Support.