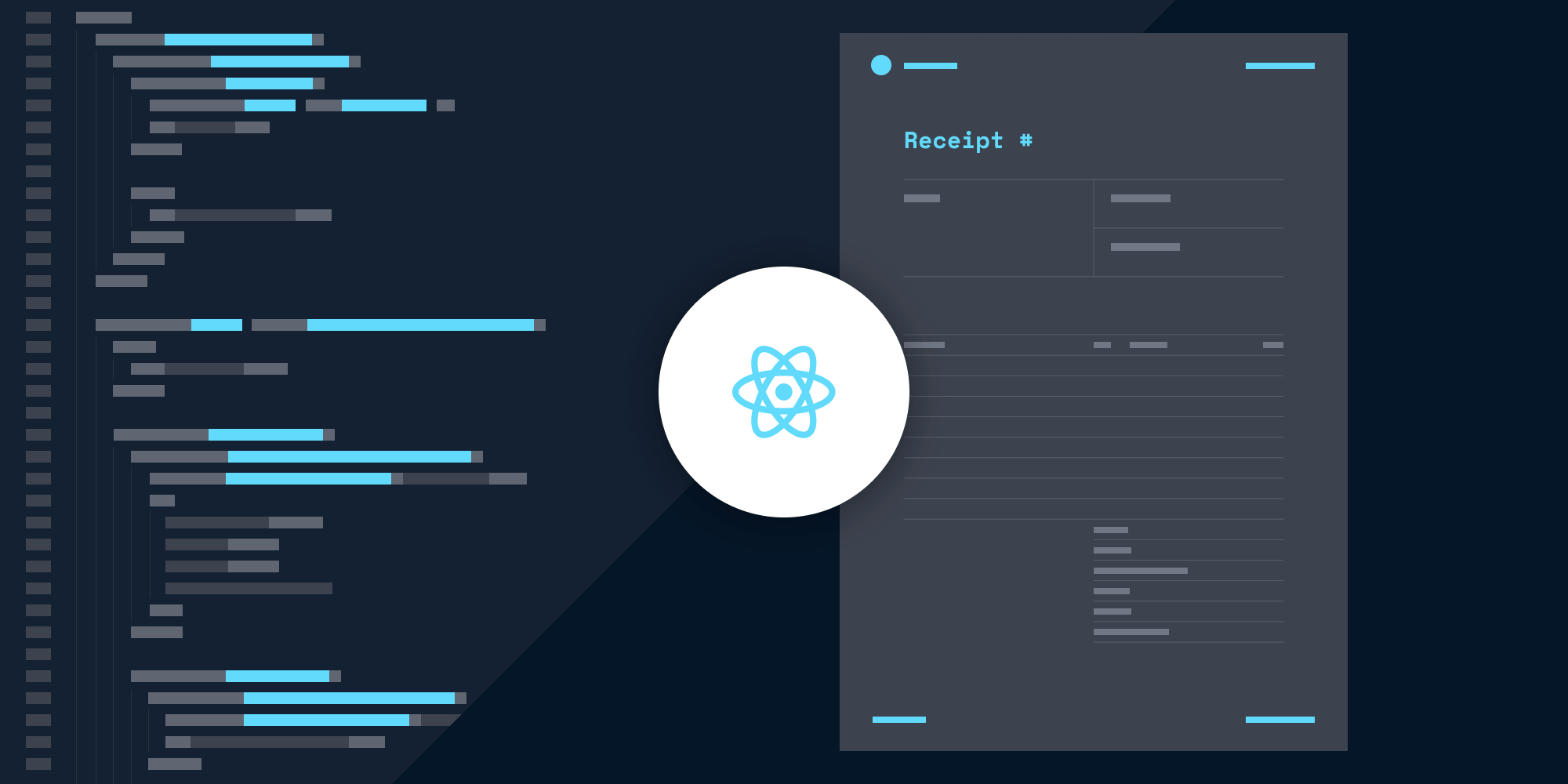
TL;DR
This tutorial demonstrates how to generate PDFs directly from React components using the react-to-pdf
package with Vite. The implementation uses the usePDF
hook to capture and convert JSX content into downloadable PDFs, ideal for creating invoices, reports, and certificates. While easy to implement, the library has limitations: PDFs are generated from screenshots rather than vector graphics (causing potential text blur when zoomed), large content may get clipped, and complex CSS may not render properly. For advanced PDF functionality like annotations or form handling, commercial solutions like Nutrient Web SDK offer more robust capabilities.
In this blog post, you’ll learn how to generate a PDF from a React component. You’ll use the React to PDF package and integrate it with a project scaffolded by Vite, a fast and modern build tool that’s an alternative to Create React App. The React to PDF package allows you to generate PDFs directly from the client side, without needing a server.
This post will walk you through installing the library, building a simple UI, and adding a button to generate PDFs. Additionally, it’ll cover potential issues and how to address them when generating PDFs in React.
What is react-to-pdf?
react-to-pdf
is a React component that allows you to generate PDF files from JSX content. It wraps the content you want to export and provides an easy-to-use button to download the resulting PDF.
Why use react-to-pdf?
There are several use cases where generating a PDF directly from a web page can be very useful:
-
Invoices or receipts — You can export invoice data filled out by a user in a web form into a downloadable PDF file.
-
Reports — Users may want to save dynamically generated data (like charts, graphs, or reports) as a PDF.
-
Certificates — If your application generates certificates, React to PDF allows you to provide users with downloadable PDFs based on their interactions.
However, a key point to remember is that React to PDF generates PDFs using screenshots of your components, which means the PDFs are not vector-based. As a result, the text may blur upon zooming in, and the captured area may not include the entire web page, depending on scroll position.
Setting up the project with Vite
Before diving into the code, you’ll first set up a React project using Vite.
Step 1 — Installing Vite and creating a new project
To create a new React project using Vite, run the following commands in your terminal:
npm create vite@latest my-pdf-app --template react cd my-pdf-app npm install
This sets up a fresh Vite project with React.
Step 2 — Installing react-to-pdf
Next, install the react-to-pdf
library to enable PDF generation functionality:
npm install react-to-pdf
Now that the project is set up, you’re ready to start building the PDF generation feature!
Step 3 — Creating the PDF generator component
Create a simple component that lets users download content as a PDF:
// App.jsx import { usePDF } from 'react-to-pdf'; const App = () => { const { toPDF, targetRef } = usePDF({ filename: 'simple-receipt.pdf', }); return ( <div className="App"> {/* Button to trigger the PDF generation */} <button onClick={() => toPDF()}>Download PDF</button> {/* The content to be exported into the PDF */} <div ref={targetRef} style={{ padding: '20px', border: '1px solid black', marginTop: '20px', }} > <h2>Receipt</h2> <p> <strong>Date:</strong> October 16, 2024 </p> <p> <strong>Total:</strong> $120.00 </p> <p>Thank you for shopping with us!</p> </div> </div> ); }; export default App;
The code uses the usePDF
hook from react-to-pdf
to generate a PDF from a specific section of a React component. Clicking the Download PDF button triggers the toPDF()
function, exporting the content inside the referenced div
as a PDF.
Step 4: Running the app with Vite
Once you’ve set up the component and installed the necessary dependencies, it’s time to run the app. With Vite, the process is simple:
npm run dev
This will start a local development server and open the app in your browser. You’ll see a Download PDF button. When clicked, a PDF file named simple-receipt.pdf
will be generated with the content of the receipt.
Customizing the PDF content
You can easily customize the content inside the div
to include any type of information you want in your PDF. The react-to-pdf
library works well with both simple and complex JSX content, so you can create invoices, reports, or even design-rich PDFs with images and styles.
Here’s an example of a customized receipt:
<div ref={targetRef} style={{ padding: '20px', border: '1px solid black', marginTop: '20px', }} > <h2>Receipt</h2> <p> <strong>Company:</strong> My Awesome Company </p> <p> <strong>Address:</strong> 123 Main Street, Springfield </p> <p> <strong>Date:</strong> October 16, 2024 </p> <h3>Items:</h3> <ul> <li>Item 1 - $50.00</li> <li>Item 2 - $30.00</li> <li>Item 3 - $40.00</li> </ul> <p> <strong>Total:</strong> $120.00 </p> <p>Thank you for shopping with us!</p> </div>
Known limitations and issues
While React to PDF is a great tool for client-side PDF generation, there are a few limitations to be aware of:
-
Text quality — Since the PDF is created using a screenshot, the text may not be as sharp as vector-based PDFs. This means that zooming in on the PDF may result in blurry text.
-
Page size — React to PDF captures the visible part of the component. If the component content exceeds the viewport height, it might get clipped.
-
CSS styling — Some CSS styles, particularly those involving complex layout and animations, may not be well-supported in the generated PDF.
For more advanced PDF functionality, like annotations or form handling, consider integrating a more robust library, like Nutrient Web SDK.
Conclusion
With just a few lines of code and the powerful combination of Vite and react-to-pdf
, you can easily add PDF export functionality to your React app. Whether you’re generating simple receipts or detailed reports, this setup allows you to provide users with the option to download important content as a PDF.
If you’re looking to add more robust PDF capabilities, we offer a commercial React PDF library that can easily be integrated into your web application. It comes with 30+ features that let you view, annotate, edit, and sign documents directly in your browser. Out of the box, it has a polished and flexible UI that you can extend or simplify based on your unique use case.
You can also deploy our vanilla JavaScript PDF viewer or use one of our many web framework deployment options like React.js, Angular, and Vue.js. To see a list of all web frameworks, start your free trial. Or, launch our demo to see our viewer in action.
FAQ
How can I create PDFs in a React application using react-to-pdf?
To create PDFs, install thereact-to-pdf
package, use the Pdf
component to wrap the content you want to export, and trigger the PDF generation through a button click or event.
What are the advantages of using react-to-pdf for PDF generation in React?
react-to-pdf
is easy to integrate and allows you to convert React components into PDFs with minimal configuration, making it ideal for exporting reports, invoices, or any web content.
How do I customize the PDF layout in a React app with react-to-pdf?
Customize the layout by applying CSS styles to your React components before rendering them as PDFs. Ensure the final document matches your design specifications.Can react-to-pdf handle dynamic content in React applications?
Yes,react-to-pdf
can handle dynamic content, allowing you to generate PDFs that include real-time data and user inputs directly from your React components.