WEB PDF SDK
Accelerate web development with a powerful PDF SDK
Seamlessly integrate comprehensive document viewing, annotation, and editing capabilities into your web applications using any JavaScript framework. Enjoy fast setup and flexible deployment options tailored to your needs.



FEATURE-RICH VIEWER
Customizable for you, seamless for your users
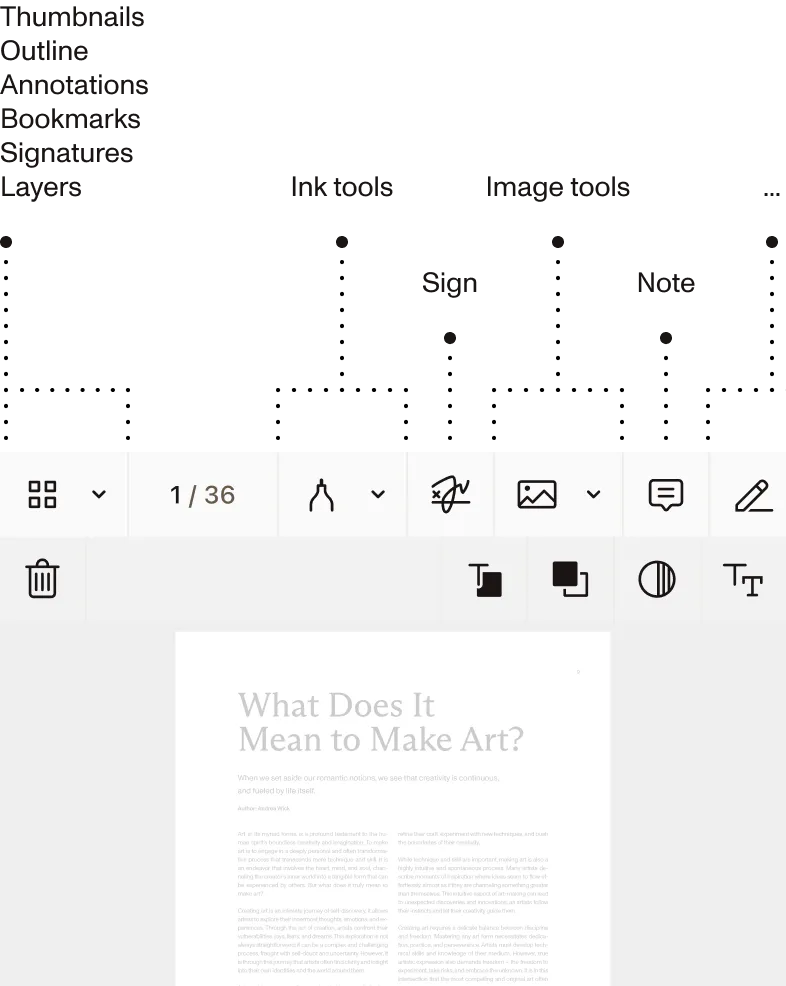
Benefits
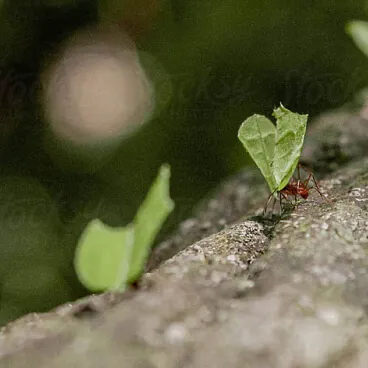
Build faster
Go to market faster than your competition with our straightforward setup process, extensive documentation, support resources, and ready-to-use code samples and templates.
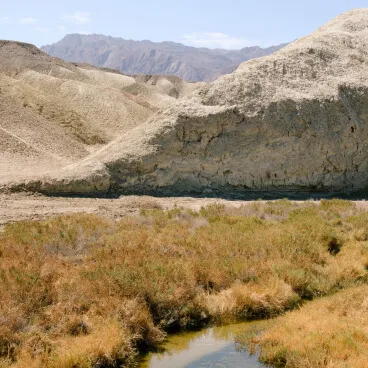
Improve ROI
Reduce the resources associated with building and maintaining robust document technology internally. Focus your efforts on creating more value for your users.

Customize more easily
Tailor the UI to match the look and feel of your app — your customers will never know you used a third-party vendor.

Scale with security
Rest easy knowing our SDKs are completely secure, reliable, and always up to date. Our dedicated support team is always on hand, giving you peace of mind, and your users a better experience.
Relied upon by industry leaders
EXAMPLES
Easy to integrate
1import PSPDFKit from "pspdfkit";
2
3const instance = await PSPDFKit.load({
4 container: "#pspdfkit",
5 document: "<pdf-file-path>",
6 licenseKey: "YOUR_LICENSE_KEY_GOES_HERE"
7});
8
9console.log("PSPDFKit for Web is ready!");
10console.log(instance);
1const PSPDFKit = require("pspdfkit");
2
3PSPDFKit.load({
4 container: "#pspdfkit",
5 document: "<pdf-file-path>",
6 licenseKey: "YOUR_LICENSE_KEY_GOES_HERE"
7}).then(function(instance) {
8 console.log("PSPDFKit for Web is ready!");
9 console.log(instance);
10})
1import PSPDFKit from "pspdfkit";
2
3const instance = await PSPDFKit.load({
4 container: "#pspdfkit",
5 document: "<pdf-file-path>",
6 licenseKey: "YOUR_LICENSE_KEY_GOES_HERE",
7 toolbarItems: [...PSPDFKit.defaultToolbarItems, {
8 type: "custom",
9 id: "my-button",
10 title: "My Button",
11 onPress: event => {
12 alert("hello from my button");
13 }
14 }]
15});
1const PSPDFKit = require("pspdfkit");
2
3PSPDFKit.load({
4 container: "#pspdfkit",
5 document: "<pdf-file-path>",
6 licenseKey: "YOUR_LICENSE_KEY_GOES_HERE",
7 toolbarItems: PSPDFKit.defaultToolbarItems.concat([{
8 type: "custom",
9 id: "my-button",
10 title: "My Button",
11 onPress: function(event) {
12 alert("hello from my button");
13 }
14 }])
15});
1import PSPDFKit from "pspdfkit";
2
3const instance = await PSPDFKit.load({
4container: "#pspdfkit",
5document: "<pdf-file-path>",
6licenseKey: "YOUR_LICENSE_KEY_GOES_HERE"
7});
8
9const annotation = new PSPDFKit.Annotations.LineAnnotation({
10 pageIndex: 0,
11 startPoint: new PSPDFKit.Geometry.Point({ x: 95, y: 95}),
12 endPoint: new PSPDFKit.Geometry.Point({ x: 195, y: 195}),
13 boundingBox: new PSPDFKit.Geometry.Rect({
14 left: 90,
15 top: 90,
16 width: 200,
17 height: 200,
18 }),
19});
20
21instance.createAnnotation(annotation);
1const PSPDFKit = require("pspdfkit");
2
3PSPDFKit.load({
4 container: "#pspdfkit",
5 document: "<pdf-file-path>",
6 licenseKey: "YOUR_LICENSE_KEY_GOES_HERE"
7}).then(instance => {
8 const annotation = new PSPDFKit.Annotations.LineAnnotation({
9 pageIndex: 0,
10 startPoint: new PSPDFKit.Geometry.Point({ x: 95, y: 95}),
11 endPoint: new PSPDFKit.Geometry.Point({ x: 195, y: 195}),
12 boundingBox: new PSPDFKit.Geometry.Rect({
13 left: 90,
14 top: 90,
15 width: 200,
16 height: 200,
17 }),
18 });
19
20 instance.createAnnotation(annotation);
21})
Knowledge center
Deployment Options
Choose the best deployment option for your business
The Nutrient Web SDK operates fully client-side in the browser, or it can be combined with Document Engine for server-supported document streaming, real-time collaboration, and improved performance.
Web SDK | Web SDK + Document Engine | |
---|---|---|
User interface | HTML5-based | HTML5-based |
Deployment | Viewer: In-browser via WebAssembly | Viewer: In-browser via WebAssembly Rendering: Self-hosted (with Docker), or managed by Nutrient |
Rendering | Client-side | Server-side |
Key notes |
|
|
Documentation | Get started | Get started |
Client-side or server-backed
The choice is yours
WebAssembly
Our standalone Web SDK runs 100 percent in the browser using robust WebAssembly technology

Document Engine
Pair Document Engine with our Web SDK, either self-hosted (with Docker) or managed by Nutrient

Pick your perfect solution
Our Web SDK is a suite of tools made to grow with your web app. You can start with what you need now and add more tools later.
Viewing
Open all documents with high fidelity in a well-designed viewer.
Open all documents with high fidelity in a well-designed viewer.
Markup
Improve the review process with a suite of annotation tools.
Improve the review process with a suite of annotation tools.
Collaboration
Bring real-time collaboration to your documents.
Bring real-time collaboration to your documents.
Editing
Modify documents and easily edit PDF text directly in your app.
Modify documents and easily edit PDF text directly in your app.
Forms
Easy for users to fill forms. Easy for you to create them programmatically.
Easy for users to fill forms. Easy for you to create them programmatically.
Signing
Streamline contract execution and approval workflows by enabling eSignatures and PDF digital signatures.
Streamline contract execution and approval workflows by enabling eSignatures and PDF digital signatures.
Generation
Effortlessly generate PDFs from HTML and DOCX.
Effortlessly generate PDFs from HTML and DOCX.
OCR and Data Extraction
Give new life to scanned documents by easily converting them into text that's selectable and searchable.
Give new life to scanned documents by easily converting them into text that's selectable and searchable.
Conversion
Easily convert any Office document, image, email, or webpage into a high-quality PDF.
Easily convert any Office document, image, email, or webpage into a high-quality PDF.
Scanning and Barcodes
Capture and process information from physical documents and products.
Capture and process information from physical documents and products.
Security and Compliance
Protect sensitive information and meet regulatory requirements within your application.
Protect sensitive information and meet regulatory requirements within your application.
Latest from the blog
Blog