Analyze usage with the Document Converter Services API
This guide explains how to use the instrumentation features in the Document Converter Services API to retrieve and report on usage data.
Using instrumentation operations
This code uses the OpenService
and CloseService
methods from the PDF Converter API DocumentConverterServiceClient
sample code:
/// <summary> /// Get a usage report from Document Converter Services. /// </summary> /// <param name="url">Service URL</param> /// <param name="targetFile">File to save the report data (optional)</param> /// <returns></returns> static string GetReport(string url, string targetFile = null) { DocumentConverterServiceClient client = null; string reportText = null; try { client = OpenService(url); ReportRequest reportRequest = new ReportRequest(); reportRequest.ByOperation = BooleanEnum.True; reportRequest.ByDate = BooleanEnum.True; reportRequest.ProductID = 4;
reportText = client.GetReport(reportRequest); if (!string.IsNullOrEmpty(targetFile)) { File.WriteAllText(targetFile, reportText); }
} catch (FaultException<WebServiceFaultException> ex) { Console.WriteLine($"FaultException occurred: ExceptionType: {ex.Detail.ExceptionType.ToString()}"); } catch (Exception ex) { Console.WriteLine(ex.ToString()); } finally { if (client != null) { CloseService(client);
} } return reportText; }
Instrumentation reporting properties
The Document Converter API includes a set of properties to filter and group usage data. You can define a report’s date range, select specific operations or product IDs, and group the results by date, month, or operation.
Property | Description |
---|---|
ByYear | Group usage data by year |
ByMonth | Group usage data by year and month |
ByDate | Group usage data by year, month, and day |
ByOperation | Group usage data by operation |
ByProductID | Group usage data by product ID |
StartDate | Return only operations that start on or after this date |
EndDate | Return only operations that start on or before this date |
Operation | Return only the specified operation |
ProductID | Return only usage data for the specified product ID (for Document Converter Services, the ProductID is 4) |
The underlying database structure is being rolled out across all Nutrient products to help you better understand your usage. Each product is assigned a unique ProductID.
Output format
The operation returns usage data as a CSV string:
date,Operation,files,filessize20250108,0,483,49691944020250108,1,26,1872470620250108,7,36,548065020250108,8,1,3332620250108,9,1,3332620250108,10,1,3332620250108,12,160,329180207
Date | Operation | Files | Size of files |
---|---|---|---|
20250108 | 0 | 483 | 496919440 |
20250108 | 1 | 26 | 18724706 |
20250108 | 7 | 36 | 5480650 |
20250108 | 8 | 1 | 33326 |
20250108 | 9 | 1 | 33326 |
20250108 | 10 | 1 | 33326 |
20250108 | 12 | 160 | 329180207 |
Operation types
The Operation column uses numeric values that map to the following operation types, with numbering beginning at 0:
- 0 — Convert
- 1 — Merge
- 2 — OCR
- 3 — Watermark
- 4 — Secure
- 5 — Split
- 6 — ExtractText
- 7 — KeyValuePairs
- 8 — SmartRedaction
- 9 — PatternRedaction
- 10 — PatternHighlight
- 11 — PDFToOffice
- 12 — DirectCall
- 13 — ConvertAny
- 14 — Compression
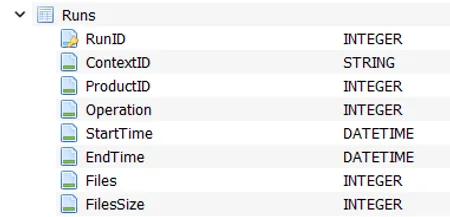