SDK Products
Accelerate innovation with intelligent PDF SDKs
Build with best-in-class PDF SDKs that seamlessly integrate into your web, mobile, and server apps — now with native AI capabilities for redaction, extraction, summarization, and more. Nutrient SDKs help you accelerate time to market, boost product differentiation, and stay compliant at scale.
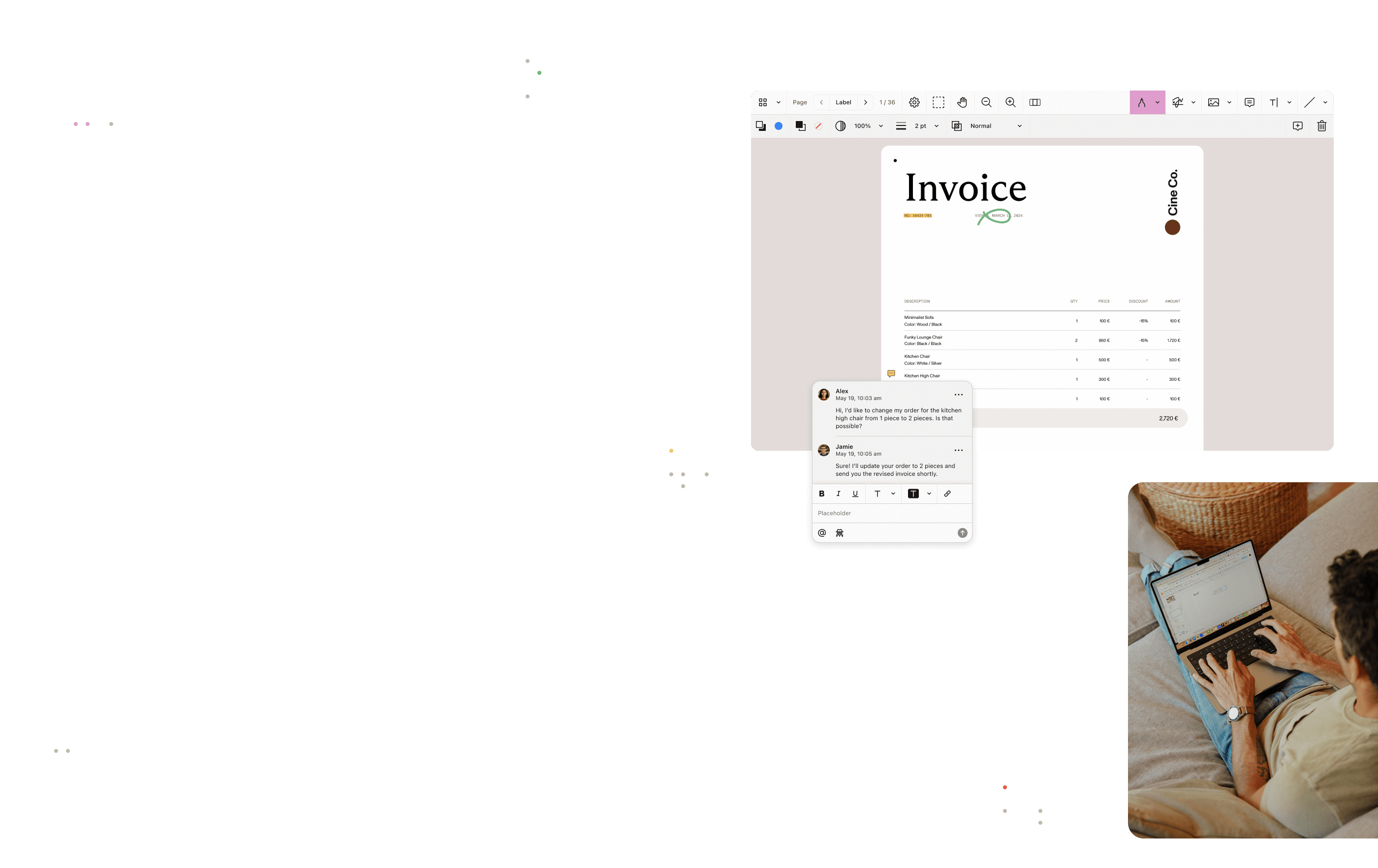
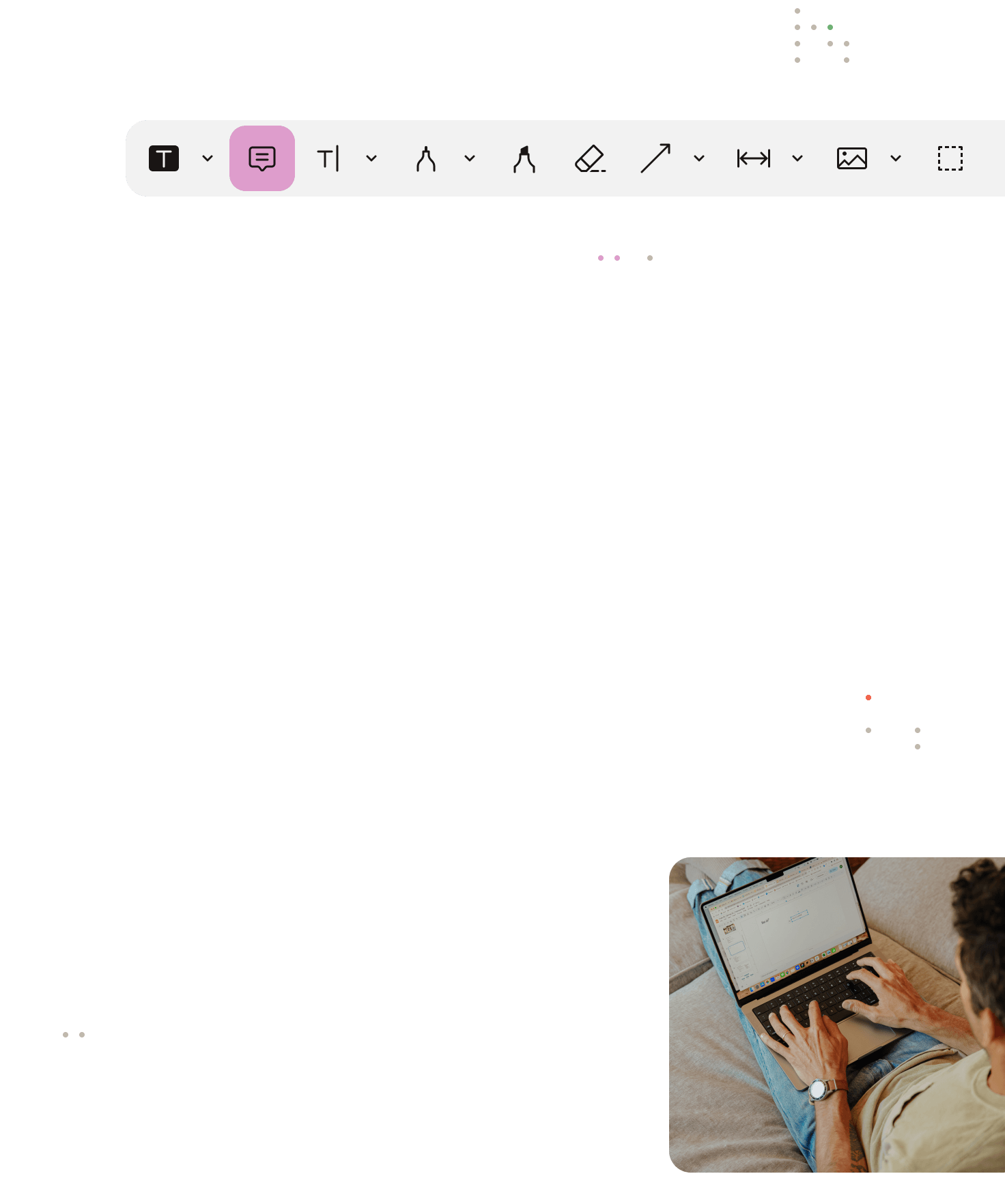
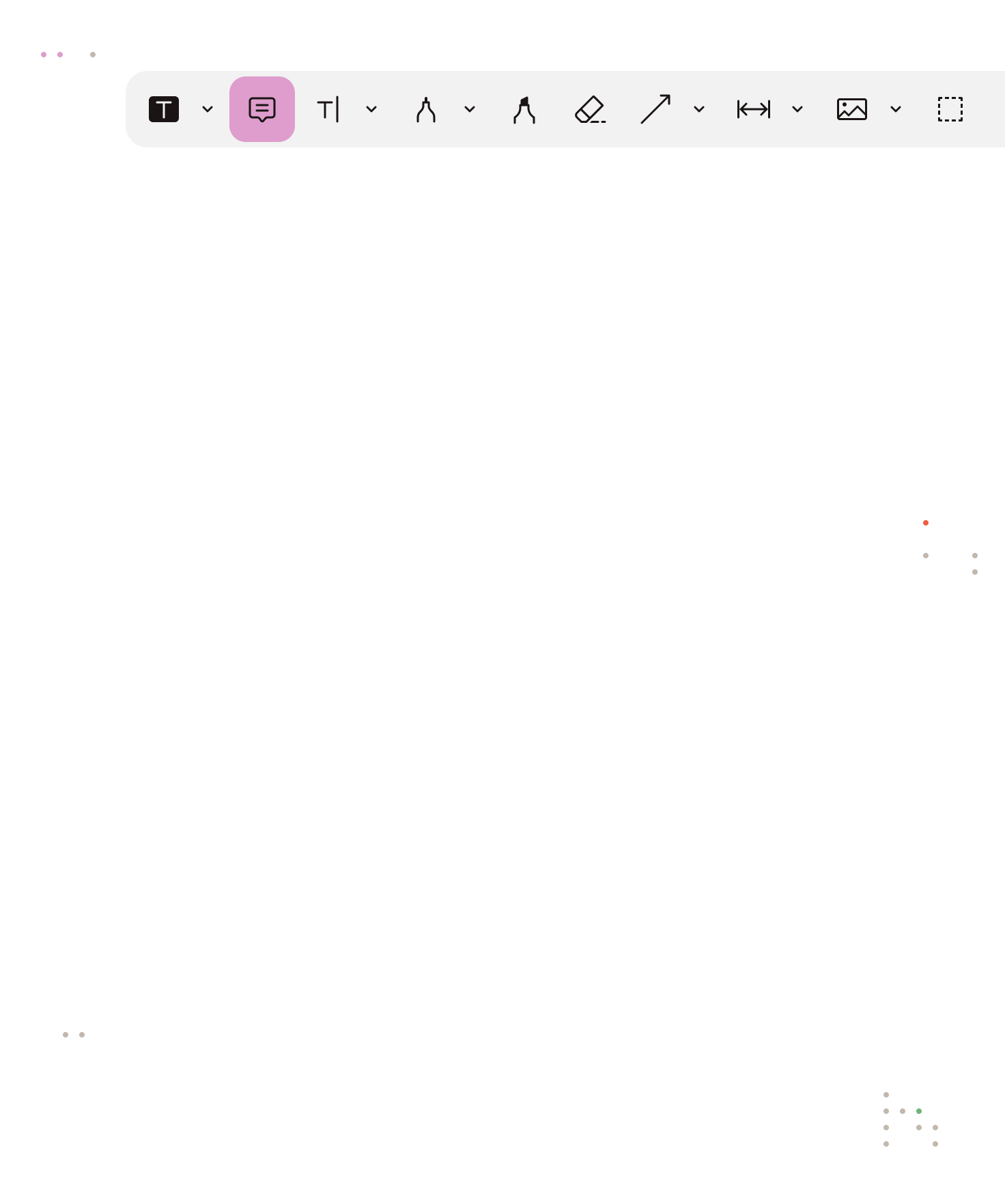
trusted by industry leaders
Build with the most intuitive and flexible PDF APIs
Integrate document generation, editing, signing, and more into your app with cross-platform SDKs built by developers for developers.
1import PSPDFKit from "pspdfkit";
2
3// Obtain a PSPDFKit document instance.
4const instance = await PSPDFKit.load({
5 container: "#pspdfkit",
6 document: "<document-file-path>",
7 licenseKey: "<license-key>"
8});
9
10console.log("PSPDFKit for Web is ready!");
11console.log(instance);
1import DocAuth from '@pspdfkit/document-authoring';
2
3const docAuthSystem = await DocAuth.createDocAuthSystem({
4 licenseKey: '<license-key>',
5});
6
7const editor = await docAuthSystem.createEditor(
8 document.getElementById('editor'),
9 {
10 document: await docAuthSystem.createDocumentFromPlaintext('Hi there!'),
11 }
12);
1import PSPDFKit
2import PSPDFKitUI
3import SwiftUI
4
5// A \`Document\` is the container for your PDF file.
6let document = Document(url: documentURL)
7
8var body: some View {
9 // A \`PDFView\` will present and manage the PSPDFKit UI.
10 PDFView(document: document)
11 .scrollDirection(.vertical)
12 .pageTransition(.scrollContinuous)
13 .pageMode(.single)
14}
1- (instancetype)initWithFrame:(CGRect)frame {
2 if ((self = [super initWithFrame:frame])) {
3 // Set configuration to use the custom annotation toolbar when initializing the \`PSPDFViewController\`.
4 // For more details, see \`PSCCustomizeAnnotationToolbarExample.m\` from PSPDFCatalog and our documentation here: https://pspdfkit.com/guides/ios/customizing-the-interface/customize-the-annotation-toolbar/
5 _pdfController = [[PSPDFViewController alloc] initWithDocument:nil configuration:[PSPDFConfiguration configurationWithBuilder:^(PSPDFConfigurationBuilder *builder) {
6 [builder overrideClass:PSPDFAnnotationToolbar.class withClass:CustomButtonAnnotationToolbar.class];
7 }]];
8
9 _pdfController.delegate = self;
10 _pdfController.annotationToolbarController.delegate = self;
11 _closeButton = [[UIBarButtonItem alloc] initWithImage:[PSPDFKitGlobal imageNamed:@"x"] style:UIBarButtonItemStylePlain target:self action:@selector(closeButtonPressed:)];
12
13 [NSNotificationCenter.defaultCenter addObserver:self selector:@selector(annotationChangedNotification:) name:PSPDFAnnotationChangedNotification object:nil];
14 [NSNotificationCenter.defaultCenter addObserver:self selector:@selector(annotationChangedNotification:) name:PSPDFAnnotationsAddedNotification object:nil];
15 [NSNotificationCenter.defaultCenter addObserver:self selector:@selector(annotationChangedNotification:) name:PSPDFAnnotationsRemovedNotification object:nil];
16 }
17
18 return self;
19}
1package com.your.package
2
3import android.net.Uri
4import android.os.Bundle
5import androidx.appcompat.app.AppCompatActivity
6import com.pspdfkit.ui.PdfActivityIntentBuilder
7
8// We need to use a Compat activity for the PdfFragment.
9class MainActivity : AppCompatActivity() {
10 override fun onCreate(savedInstanceState: Bundle?) {
11 super.onCreate(savedInstanceState)
12
13 // Get the document path from the application assets.
14 val documentUri = Uri.parse("file:///android_asset/document.pdf")
15
16 // Build the \`Intent\` for launching the \`PdfActivity\`.
17 val intent = PdfActivityIntentBuilder.fromUri(this, documentUri).build()
18
19 // Launch the activity.
20 startActivity(intent)
21 }
22}
1package com.your.package;
2
3import android.content.Intent;
4import android.net.Uri;
5import android.os.Bundle;
6import androidx.appcompat.app.AppCompatActivity;
7import com.pspdfkit.ui.PdfActivityIntentBuilder;
8
9// We need to use a Compat activity for the PdfFragment.
10public class MainActivity extends AppCompatActivity {
11 @Override
12 protected void onCreate(Bundle savedInstanceState) {
13 super.onCreate(savedInstanceState);
14
15 // Get the document path from the application assets.
16 final Uri documentUri = Uri.parse("file:///android_asset/document.pdf");
17
18 // Build the \`Intent\` for launching the \`PdfActivity\`.
19 final Intent intent = PdfActivityIntentBuilder.fromUri(this, documentUri).build();
20
21 // Launch the activity.
22 startActivity(intent);
23 }
24}
1import 'dart:io';
2
3import 'package:flutter/material.dart';
4import 'package:path_provider/path_provider.dart';
5import 'package:pspdfkit_flutter/pspdfkit.dart';
6
7const String DOCUMENT_PATH = 'PDFs/Document.pdf';
8
9void main() => runApp(MyApp());
10
11class MyApp extends StatefulWidget {
12@override
13_MyAppState createState() => _MyAppState();
14}
15
16class _MyAppState extends State<MyApp> {
17void showDocument(BuildContext context) async {
18 final bytes = await DefaultAssetBundle.of(context).load(DOCUMENT_PATH);
19 final list = bytes.buffer.asUint8List();
20
21 final tempDir = await getTemporaryDirectory();
22 final tempDocumentPath = '$\{tempDir.path\}/$DOCUMENT_PATH';
23
24 final file = await File(tempDocumentPath).create(recursive: true);
25 file.writeAsBytesSync(list);
26
27 await Pspdfkit.present(tempDocumentPath);
28}
29
30@override
31Widget build(BuildContext context) {
32 final themeData = Theme.of(context);
33 return MaterialApp(
34 home: Scaffold(
35 body: Builder(
36 builder: (BuildContext context) {
37 return Center(
38 child: Column(
39 mainAxisAlignment: MainAxisAlignment.spaceEvenly,
40 children: [
41 ElevatedButton(
42 child: Text('Tap to Open Document',
43 style: themeData.textTheme.headline4?.copyWith(fontSize: 21.0)),
44 onPressed: () => showDocument(context))
45 ]));
46 },
47 )),
48 );
49}
50}
1import React, {Component} from 'react';
2import {Platform} from 'react-native';
3import PSPDFKitView from 'react-native-pspdfkit';
4
5const DOCUMENT =
6 Platform.OS === 'ios' ? 'Document.pdf' : 'file:///android_asset/Document.pdf';
7export default class PSPDFKitDemo extends Component<{}> {
8 render() {
9 return (
10 <PSPDFKitView
11 document={DOCUMENT}
12 configuration={{
13 showThumbnailBar: 'scrollable',
14 pageTransition: 'scrollContinuous',
15 scrollDirection: 'vertical',
16 }}
17 ref={this.pdfRef}
18 fragmentTag="PDF1"
19 style={{flex: 1}}
20 />
21 );
22 }
23}
1var app = {
2 // Application Constructor
3 initialize: function() {
4 document.addEventListener('deviceready', this.onDeviceReady.bind(this), false);
5 },
6
7 // deviceready Event Handler
8 //
9 // Bind any cordova events here. Common events are:
10 // 'pause', 'resume', etc.
11 onDeviceReady: function() {
12 this.receivedEvent('deviceready');
13 const DOCUMENT = (window.cordova.platformId === "ios") ? "Document.pdf" : "file:///android_asset/Document.pdf";
14 PSPDFKit.present(DOCUMENT);
15 },
16
17 // Update DOM on a Received Event
18 receivedEvent: function(id) {
19 var parentElement = document.getElementById(id);
20 var listeningElement = parentElement.querySelector('.listening');
21 var receivedElement = parentElement.querySelector('.received');
22
23 listeningElement.setAttribute('style', 'display:none;');
24 receivedElement.setAttribute('style', 'display:block;');
25
26 console.log('Received Event: ' + id);
27 }
28};
29
30app.initialize();
1using PSPDFKit.Model;
2using PSPDFKit.UI;
3using PSPDFKit.Instant;
4
5var configuration = PSPDFConfiguration.FromConfigurationBuilder ((builder) => {
6 builder.PageMode = PSPDFPageMode.Single;
7 builder.PageTransition = PSPDFPageTransition.ScrollContinuous;
8 builder.ScrollDirection = PSPDFScrollDirection.Vertical;
9}));
10
11var document = new PSPDFDocument (NSUrl.FromFilename ("document.pdf"));
12var pdfViewController = new PSPDFViewController (document, configuration);
1import { Component } from "@angular/core";
2import { Platform } from "@ionic/angular";
3
4@Component({
5 selector: "app-root",
6 templateUrl: "app.component.html",
7 styleUrls: ["app.component.scss"],
8})
9export class AppComponent {
10 constructor(private platform: Platform) {
11 this.platform.ready().then(() => {
12 const DOCUMENT = this.platform.is("ios")
13 ? "Document.pdf"
14 : "file:///android_asset/Document.pdf";
15 PSPDFKit.present(DOCUMENT);
16 });
17 }
18}
1using (GdPictureDocumentConverter oConverter = new GdPictureDocumentConverter())
2{
3 // Select the source document and its file format (DOCX, DOC, XLSX, XLS, PPTX, PPT).
4 oConverter.LoadFromFile("input.docx", GdPicture14.DocumentFormat.DocumentFormatDOCX);
5 // Convert the source document to PDF.
6 oConverter.SaveAsPDF("output.pdf", PdfConformance.PDF);
7}
1Using oConverter As GdPictureDocumentConverter = New GdPictureDocumentConverter()
2 'Select the source document and its file format (DOCX, DOC, XLSX, XLS, PPTX, PPT).
3 oConverter.LoadFromFile("input.docx", GdPicture14.DocumentFormat.DocumentFormatDOCX)
4 'Convert the source document to PDF.
5 Converter.SaveAsPDF("output.pdf", PdfConformance.PDF)
6End Using
1# You simply supply a list of document operations to apply.
2curl -F [email protected] \\
3 -F operations='{"operations":[{"type": "flattenAnnotations"}]}' \\
4 http://localhost:5000/process \\
5 --output result.pdf
1// Initialize PSPDFKit with your activation key.
2PSPDFKit.initialize("YOUR_LICENSE_KEY_GOES_HERE");
3
4// Open a document to work on.
5File file = new File("assets/default.pdf");
6PdfDocument document = new PdfDocument(new FileDataProvider(file));
7
8// Add a new stamp annotation.
9JSONObject jsonObject = new JSONObject();
10jsonObject.put("bbox", new float[]{0, 0, 100, 50});
11jsonObject.put("pageIndex", 0);
12jsonObject.put("type", "pspdfkit/stamp");
13jsonObject.put("stampType", "Approved");
14jsonObject.put("opacity", 1);
15jsonObject.put("v", 1);
16document.getAnnotationProvider().addAnnotationJson(jsonObject);
17
18// Export the changes to Instant Document JSON.
19File jsonFile = new File("out/instantOutput.json");
20if (jsonFile.createNewFile()) {
21 document.exportDocumentJson(new FileDataProvider(jsonFile));
22}
23
24// Render the first page and save to a PNG.
25BufferedImage image = document.getPage(0).renderPage();
26File pngfile = new File("out/test.png");
27boolean success = ImageIO.write(image, "png", pngfile);
1# You simply supply a list of document operations to apply.
2curl -F [email protected] \\
3 -F operations='{"operations":[{"type": "flattenAnnotations"}]}' \\
4 http://localhost:5000/process \\
5 --output result.pdf
1// This loads a PDF from \`Assets\` as soon as the \`PdfView\` is ready.
2private async void PdfViewInitializationCompletedHandler(PdfView sender, Document args)
3{
4 try
5 {
6 var file = await StorageFile.GetFileFromApplicationUriAsync(new Uri("ms-appx:///Assets/document.pdf"));
7 if (file == null) return;
8
9 await sender.OpenStorageFileAsync(file);
10 }
11 catch (Exception e)
12 {
13 var messageDialog = new MessageDialog(e.Message);
14 await messageDialog.ShowAsync();
15 }
16}
17
18// This loads a PDF from a file picked by the user in the UI.
19private async void Button_OpenPDF_Click(object sender, RoutedEventArgs e)
20{
21 // Open a \`Picker\` so the user can choose a PDF.
22 var picker = new FileOpenPicker
23 {
24 ViewMode = PickerViewMode.Thumbnail,
25 SuggestedStartLocation = PickerLocationId.DocumentsLibrary
26 };
27 picker.FileTypeFilter.Add(".pdf");
28
29 var file = await picker.PickSingleFileAsync();
30 if (file == null) return;
31
32 // Open and display it in the PSPDFKit \`PdfView\`.
33 var documentSource = DocumentSource.CreateFromStorageFile(file);
34 await PdfView.Controller.ShowDocumentAsync(documentSource);
35}
1Imports Windows.Storage
2Imports Windows.Storage.Pickers
3Imports PSPDFKit.Document
4Imports PSPDFKit.Pdf
5Imports PSPDFKit.UI
6
7Public NotInheritable Class MainPage
8Inherits Page
9
10Private Async Sub PdfViewInitializationCompletedHandler(sender As PdfView, args As Document)
11Dim file As StorageFile
12file = Await StorageFile.GetFileFromApplicationUriAsync(New Uri("ms-appx:///Assets/document.pdf"))
13
14If file IsNot Nothing Then
15Await sender.OpenStorageFileAsync(file)
16End If
17End Sub
18
19Private Async Sub Button_OpenPDF_Click(sender As Object, e As RoutedEventArgs)
20Dim picker As New FileOpenPicker
21picker.FileTypeFilter.Add(".pdf")
22
23Dim file = Await picker.PickSingleFileAsync
24If file IsNot Nothing Then
25Dim documentSource As DocumentSource
26documentSource = DocumentSource.CreateFromStorageFile(file)
27Await PdfView.Controller.ShowDocumentAsync(documentSource)
28End If
29End Sub
30End Class
1// This loads a PDF from \`Assets\` as soon as the \`PdfView\` is ready.
2void MainPage::PdfViewInitializationCompletedHandler(UI::PdfView^ sender, Pdf::Document^ args)
3{
4 const auto path = ref new Uri("ms-appx:///Assets/document.pdf");
5
6 create_task(StorageFile::GetFileFromApplicationUriAsync(path))
7 .then([this](StorageFile^ file)
8 {
9 if (file == nullptr) return;
10
11 PdfView->OpenStorageFileAsync(file);
12 });
13}
14
15// This loads a PDF from a file picked by the user in the UI.
16void MainPage::Button_OpenPDF_Click(Platform::Object^ sender, RoutedEventArgs^ e)
17{
18 // Open a \`Picker\` so the user can choose a PDF.
19 FileOpenPicker^ openPicker = ref new FileOpenPicker();
20 openPicker->ViewMode = PickerViewMode::Thumbnail;
21 openPicker->SuggestedStartLocation = PickerLocationId::PicturesLibrary;
22 openPicker->FileTypeFilter->Append(".pdf");
23
24 create_task(openPicker->PickSingleFileAsync())
25 .then([this](StorageFile^ file)
26 {
27 if (file == nullptr) return;
28
29 // Open and display it in the PSPDFKit \`PdfView\`.
30 const auto documentSource = DocumentSource::CreateFromStorageFile(file);
31 PdfView->Controller->ShowDocumentAsync(documentSource);
32 });
33}
1<Page
2x:Class="BasicExample.MainPage"
3xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
4xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
5xmlns:local="using:BasicExample"
6xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
7xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
8xmlns:ui="using:PSPDFKit.UI"
9mc:Ignorable="d"
10Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
11 <Page.Resources>
12 <x:String x:Key="license">YOUR LICENSE GOES HERE</x:String>
13 </Page.Resources>
14
15 <Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
16 <Grid.RowDefinitions>
17 <RowDefinition Height="*"/>
18 <RowDefinition Height="52"/>
19 </Grid.RowDefinitions>
20 <ui:PdfView Grid.Row="0" Name="PdfView" License="{StaticResource license}" InitializationCompletedHandler="PdfViewInitializationCompletedHandler"/>
21 <Button Content="Open PDF" HorizontalAlignment="Left" Margin="10" Grid.Row="1" Name="Button_OpenPDF" Click="Button_OpenPDF_Click"/>
22 </Grid>
23</Page>
1import PSPDFKit
2import PSPDFKitUI
3import SwiftUI
4
5// A \`Document\` is the container for your PDF file.
6let document = Document(url: documentURL)
7
8var body: some View {
9 // A \`PDFView\` will present and manage the PSPDFKit UI.
10 PDFView(document: document)
11 .scrollDirection(.vertical)
12 .pageTransition(.scrollContinuous)
13 .pageMode(.single)
14}
1- (instancetype)initWithFrame:(CGRect)frame {
2 if ((self = [super initWithFrame:frame])) {
3 // Set configuration to use the custom annotation toolbar when initializing the \`PSPDFViewController\`.
4 // For more details, see \`PSCCustomizeAnnotationToolbarExample.m\` from PSPDFCatalog and our documentation here: https://pspdfkit.com/guides/ios/customizing-the-interface/customize-the-annotation-toolbar/
5 _pdfController = [[PSPDFViewController alloc] initWithDocument:nil configuration:[PSPDFConfiguration configurationWithBuilder:^(PSPDFConfigurationBuilder *builder) {
6 [builder overrideClass:PSPDFAnnotationToolbar.class withClass:CustomButtonAnnotationToolbar.class];
7 }]];
8
9 _pdfController.delegate = self;
10 _pdfController.annotationToolbarController.delegate = self;
11 _closeButton = [[UIBarButtonItem alloc] initWithImage:[PSPDFKitGlobal imageNamed:@"x"] style:UIBarButtonItemStylePlain target:self action:@selector(closeButtonPressed:)];
12
13 [NSNotificationCenter.defaultCenter addObserver:self selector:@selector(annotationChangedNotification:) name:PSPDFAnnotationChangedNotification object:nil];
14 [NSNotificationCenter.defaultCenter addObserver:self selector:@selector(annotationChangedNotification:) name:PSPDFAnnotationsAddedNotification object:nil];
15 [NSNotificationCenter.defaultCenter addObserver:self selector:@selector(annotationChangedNotification:) name:PSPDFAnnotationsRemovedNotification object:nil];
16 }
17
18 return self;
19}
1import PSPDFKit from "pspdfkit";
2
3// Obtain a PSPDFKit document instance.
4const instance = await PSPDFKit.load({
5 container: "#pspdfkit",
6 document: "<document-file-path>",
7 licenseKey: "<license-key>"
8});
9
10console.log("PSPDFKit for Web is ready!");
11console.log(instance);
1curl -X POST https://api.pspdfkit.com/build
2 -H "Authorization: Bearer your_api_key_here"
3 -o result.pdf
4 -F [email protected]
5 -F instructions='{
6 "parts": [
7 { "html": "index.html" }
8 ]
9 }'
1curl -X POST https://api.pspdfkit.com/build
2 -H "Authorization: Bearer your_api_key_here"
3 -o converted.pdf
4 -F [email protected]
5 -F instructions='{
6 "parts": [
7 { "file": "document" }
8 ]
9 }'
1curl -X POST https://api.pspdfkit.com/build
2 -H "Authorization: Bearer your_api_key_here"
3 -o merged.pdf
4 -F [email protected]
5 -F [email protected]
6 -F instructions='{
7 "parts": [
8 { "file": "part1" },
9 { "file": "part2" }
10 ]
11 }'
1curl -X POST https://api.pspdfkit.com/build
2 -H "Authorization: Bearer your_api_key_here"
3 -o result.pdf
4 -F [email protected]
5 -F instructions='{
6 "parts": [
7 { "file": "document" }
8 ],
9 "actions": [{
10 "type": "ocr",
11 "language": "english"
12 }]
13 }'
Choose your SDK
Our document SDKs offer flexible deployment options for web, server, and mobile applications.
Web viewer
Integrate advanced document viewing and user-driven processing functionality directly into your web app. Build with customizable SDKs that support leading JavaScript frameworks and all major browsers.
Learn MoreMobile viewer
Add advanced document viewing into your iOS, Android, and cross-platform development apps. Support native or leverage cross-platform frameworks to build phenomenal user experiences faster.
Learn MoreServer processing
Leverage advanced document processing in your server apps and backend services. Quickly enable performant document generation, editing, conversion, compression, data extraction, and more.
Learn MorePurpose-built AI for document-driven work
The same document-tuned AI engine powers every Nutrient product — from SDKs to low-code tools to end-to-end workflows. Redact, extract, summarize, and automate with drop-in intelligence that works across web, mobile, and server environments.


Understand
Summaries, smart diffs, and chat answers in seconds.
Protect
Confidence‑scored redaction with full audit trails.
Extract
Prompt‑based data capture, no templates required.
Automate
No-code workflows trigger downstream actions.
Deploy
In-browser, in the cloud, or self-hosted — same AI engine, same results
What you get building with Nutrient SDKs
When you build with our document SDKs, you’ll accelerate development speed, save on wasted engineering cycles, and easily leverage cutting-edge AI functionality.
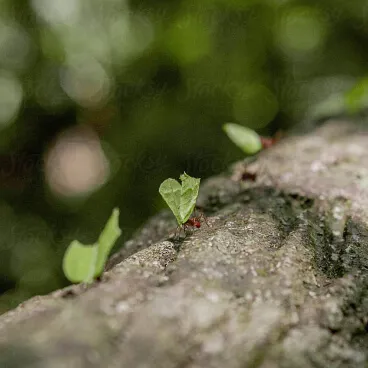
Accelerate development
Slash the time to develop and maintain with our fully documented SDKs. Leverage years of research and development from working with thousands of customers, enterprise-level support, and our robust catalog of examples to ship document functionality faster.

Save time and money
Save on engineering time spent adapting to platform changes, bug fixing, and managing support requests around documents. Focus more time on the core value of your application by removing the headache of integrating and maintaining buggy open source or homegrown document solutions.
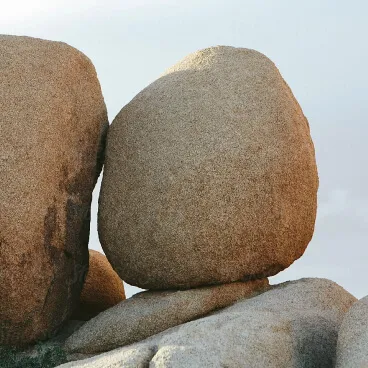
AI at your fingertips
Deliver AI functionality easily with our AI-assisted document SDKs. Whether you’re looking to add AI-assisted summarization, translation, redaction, and data extraction, or build MCP agentic-driven workflows, our SDKs are the simplest way to ship AI in your document apps and workflows.
A Great User Experience
Easy to use — easier to customize
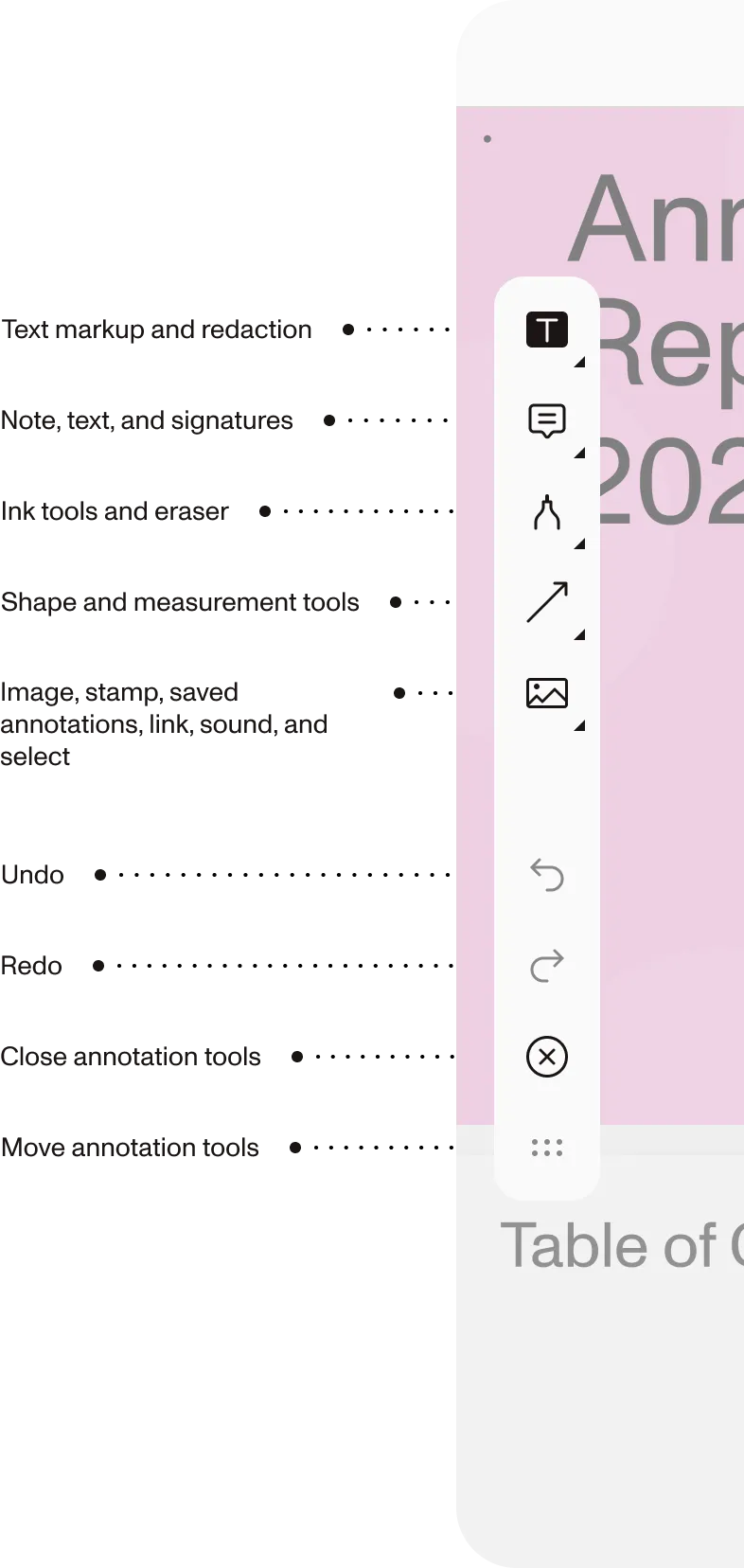
Discover why developers love Nutrient SDKs
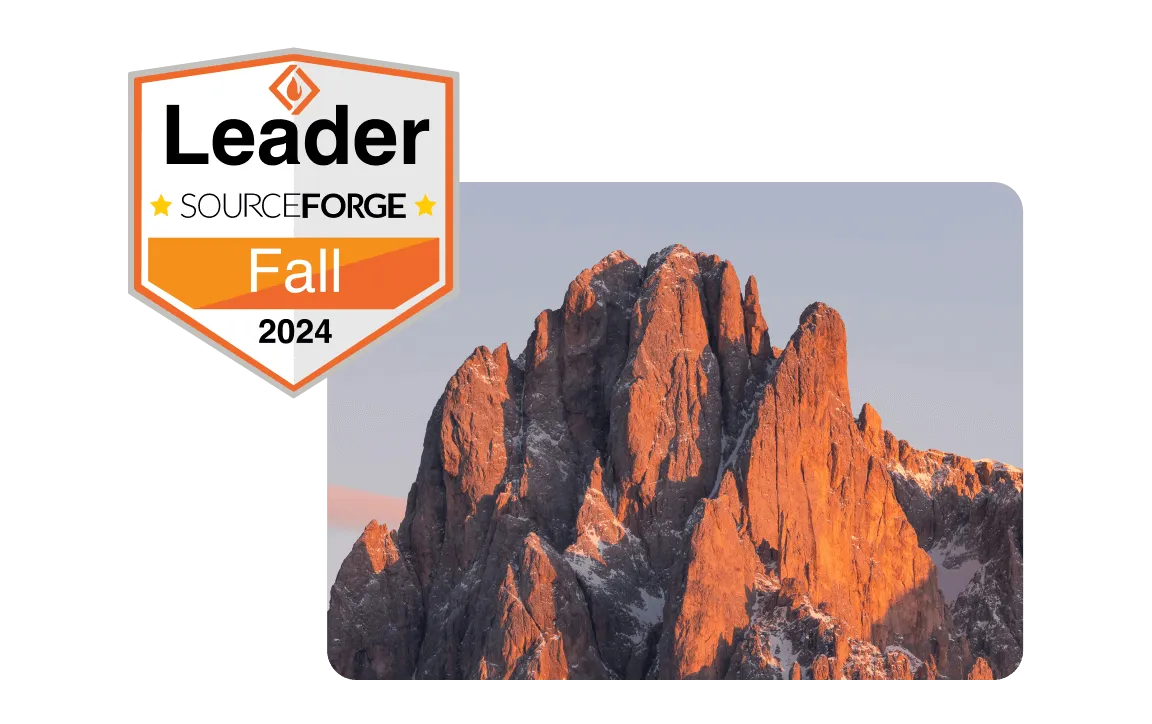
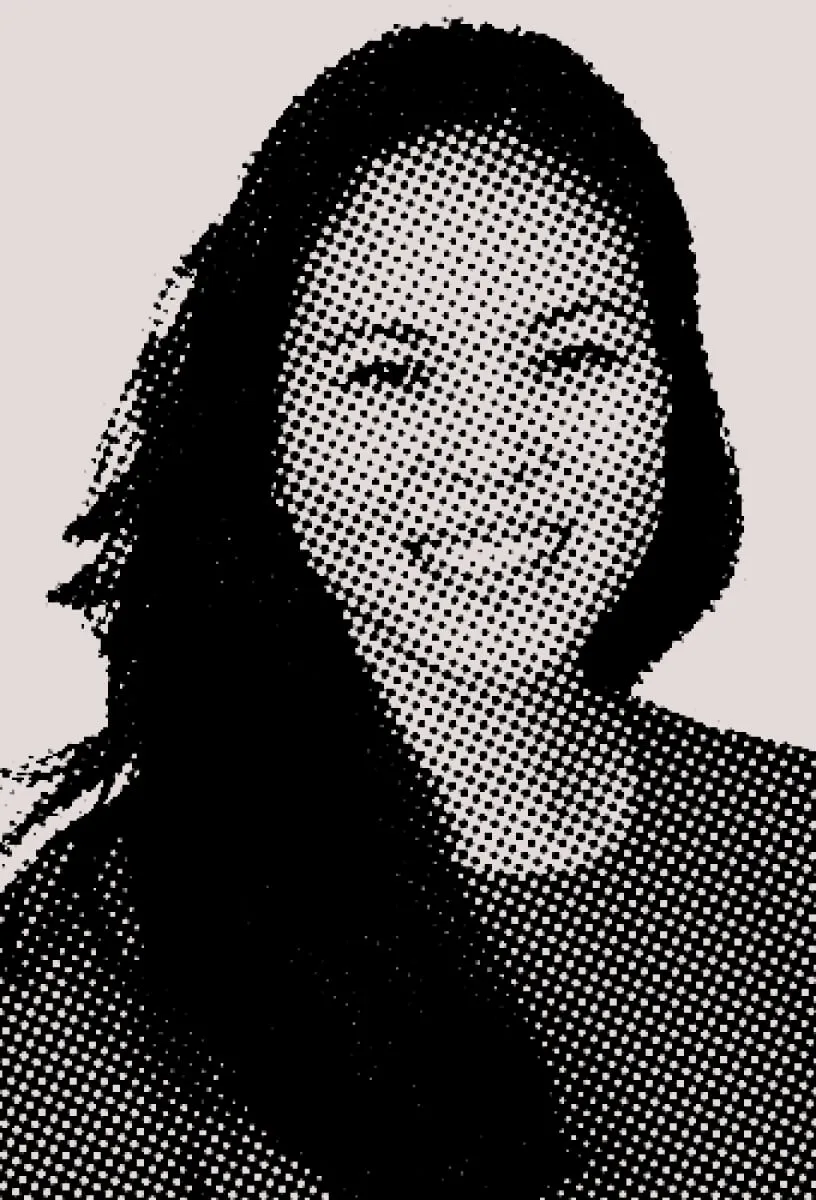
Angelica Nierras
Chief Growth Officer
“Nutrient helps us significantly accelerate our time to market, provide key services to our clients, and reliably deliver solutions that we can easily integrate into our portfolio.”
Faria Education Group
Shift your development into overdrive
reduction in development costs
of customers report faster time to market
Frequently asked questions
What is a PDF SDK?
A PDF SDK (software development kit) provides developers with tools to integrate robust PDF capabilities into their applications — like viewing, editing, signing, generating, converting, and more. Nutrient’s PDF SDK is a complete solution that works across web, mobile, and server platforms, helping you build secure, scalable, and interactive document support in your application without relying on external tools.
- View, edit, annotate, and sign PDFs directly in your app.
- Generate documents from Word templates or HTML.
- Convert between PDF and Word, Excel, PowerPoint, and PDF/A.
- Redact, extract data, and perform advanced OCR on scanned documents.
- Integrate seamlessly with JavaScript, .NET, Java, Node.js, iOS, Android, and more.
Can I use Nutrient PDF SDKs on multiple platforms?
Yes! Nutrient PDF SDKs are optimized for web, native mobile, cross-platform, and server platforms and languages, ensuring high performance across all platforms.
What file types and frameworks are supported by Nutrient’s PDF SDKs?
Our comprehensive developer toolkits provide performant, secure and reliable support for all major file formats (including PDF, DOCX, PPTX, PNG, JPG, and more) and all major developer frameworks. Learn more in our documentation.
Are Nutrient’s PDF SDKs customizable to match a brand’s UI/UX design?
Nutrient SDKs make it easy to customize every part of the user interface in your PDF documents. Our robust API for configuring behavior and appearance lets you hide or add buttons, change the theme to match your look and feel, trigger workflows, create overlays, and much more. See our user interface customization guide on Web Viewer or switch to other platforms for more details.
Are Nutrient’s PDF SDKs secure?
Absolutely. Nutrient’s PDF SDKs are based on an extensive fork of PDFium, the most trusted platform for PDF rendering. PDFium is the same PDF engine used in Chromium, Android, and countless other applications. The PDFium project is backed and actively maintained by Google, Microsoft, Amazon, Dropbox, and ourselves. We’ve been building and optimizing our fork of PDFium for more than 10 years now.
Encrypted PDFs are supported, and they can’t be accessed without the matching password. PDF passwords are never persisted. Additionally, we’re SOC 2 Type 2 audited, and all our code commits undergo peer review and extensive testing before being merged to production. For more details, check out our Trust Center.
How do Nutrient PDF SDKs ensure high-fidelity PDF rendering across different browsers and devices?
Nutrient PDF SDKs have built-in mobile support for displaying your PDFs. They come with a responsive UI that works on all screen sizes and device sizes, ensuring the UI adapts to the screen size automatically. Nutrient PDF SDKs provide a similar feature set across desktop, tablet, and mobile viewers.
What resources are available for developers?
Our comprehensive guides and API reference outline simple step-by-step instructions to add document functionality to any of your apps. We also have a knowledge base and extensive code samples available across all platforms. Technical support is provided via our online portal during both integration and after you’ve come aboard as a customer.
Why choose a PDF SDK over a third-party general document solution?
PDF SDKs specialize in precise PDF processing and allow you as a developer to integrate them specifically to serve the needs of your use case. PDF SDKs also offer advanced capabilities like OCR, smart redaction, AI functionality, and text comparison. These capabilities enable businesses to handle sensitive information, extract data securely, and execute digital signatures with ease. They’re also versatile across many different industries — like legal, finance, and education — offering time savings and scalability for apps and enterprise platforms.
How do I get started with Nutrient’s PDF SDK?
To get started now, grab a license key for the platform you’re building on by clicking here. Next, visit our documentation page to access everything you need — from guides with sample code to API references. If you need help, our Support team is ready to assist you!
PDF SDK
What is a PDF SDK?
A PDF SDK (software development kit) provides developers with tools to integrate robust PDF capabilities into their applications — like viewing, editing, signing, generating, converting, and more. Nutrient’s PDF SDK is a complete solution that works across web, mobile, and server platforms, helping you build secure, scalable, and interactive document support in your application, without relying on external tools.
- View, edit, annotate, and sign PDFs directly in your app.
- Generate documents from Word templates or HTML.
- Convert between PDF and Word, Excel, PowerPoint, and PDF/A.
- Redact, extract data, and perform advanced OCR on scanned documents.
- Integrate seamlessly with JavaScript, .NET, Java, Node.js, iOS, Android, and more.
How to choose the right PDF SDK?
Choosing a PDF SDK comes down to flexibility, performance, and platform coverage. Consider these key questions:
- Cross-platform support — Does it work on web, mobile (iOS/Android), and backend systems?
- Modular architecture — Can you start with core features and expand to advanced features like OCR, redaction, or signing later?
- Performance and rendering — How does it handle large or complex PDFs across devices?
- Developer experience — Is the SDK well-documented, actively maintained, and easy to integrate?
- Security and compliance — Can it run on-premises or in secure environments to meet data privacy requirements?
What are the best PDF SDKs to solve my PDF integration needs?
The best PDF SDK depends on your use case — whether it’s lightweight client-side only deployment, secure signing, or the ability to fully automate your document workflows. Nutrient’s PDF SDK has a full-featured PDF engine with AI built into it, giving you best-in-class performance, cross-platform support, and modular APIs that developers love. It’s trusted by thousands of companies —from startups, to multinational enterprises across the globe — and used by billions of end users.
What are the benefits of using Nutrient’s PDF SDK?
Nutrient’s PDF SDK is the core engine behind all types of use cases — contract management, form filling, document workflows, compliance, and more — all within your app’s native environment.
- Unified API across platforms — Consistent, phenomenal developer experience across Web, iOS, Android, and Server.
- Modular feature set — Start with viewing and annotations, and then scale into signing, OCR, redaction, and collaboration.
- Faster time to market — Built-in UI components and code examples accelerate your development.
- Secure by design — Keep documents in your environment — no risky third-party uploads.
- Enterprise-ready — Deploy on-premises, in the cloud, or in hybrid environments with robust compliance, accessibility, and enterprise support.
How does Nutrient’s PDF SDK compare to others?
Nutrient stands out by focusing on developer-first tools with enterprise-level performance, scalability, and support. It’s ideal for engineering and product teams that need flexibility, performance, and real ROI.
- Broader platform support — Web, mobile, desktop, and server — all from one vendor.
- Easier to embed — Clean APIs and UI components save weeks of custom work building in house or on top of open source.
- Lower cost and vendor risk — Replace multiple tools from multiple vendors with a single, battle-tested, secure, unified PDF engine.
- Vendor partner — We view our relationship with customers as a partnership, and work individually with them to help them achieve their goals.
- Backed by developer-first support — Quickstart guides, extensive code examples, comprehensive documentation, and support from the developers that build the product.