Zoom Options in Our JavaScript PDF Viewer
PSPDFKit for Web features different UI elements and API helpers to both manage zooming in and out of a document and handle zooming automatically.
Zooming with the UI
Documents opened in PSPDFKit for Web’s viewer can be zoomed in on and out of using the corresponding toolbar buttons, zoom-in
and zoom-out
:
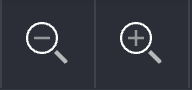
Zooming can also be controlled using keyboard shortcuts:
-
Control/Command + + — zoom in
-
Control/Command + - — zoom out
-
Control/Command + 0 — zoom to auto
-
Control + Scroll wheel — zoom in or out at mouse position
The zoom-mode
button can be used to toggle the current zoom so that the page fits either the viewport width or the viewport height.

The marquee-zoom
button, hidden by default, can be used to zoom to the area of a rectangle drawn by the user.

The marquee-zoom
button can be enabled in the PSPDFKit.Configuration
object passed to PSPDFKit.load()
:
PSPDFKit.load({
toolbarItems: PSPDFKit.defaultToolbarItems.concat([
{ type: "marquee-zoom" }
])
});
Or, it can be enabled using the instance.setToolbarItems()
API method:
instance.setToolbarItems((items) =>
items.concat([{ type: "marquee-zoom" }])
);
Zooming at Mouse Position
PSPDFKit supports zooming with the scroll wheel with this shortcut:
-
Control-Scroll wheel — zoom in or out at mouse position
When the Control key isn’t pressed, using the scroll wheel will scroll through the pages. But when Control is pressed, using the scroll wheel will zoom the page either in or out, depending on the direction of the scroll.
If you want to always zoom using the scroll wheel without having to press Control, you can use PSPDFKit.ViewState#enableAlwaysScrollToZoom
. The default value of enableAlwaysScrollToZoom
is false
:
instance.setViewState((viewState) => viewState.set("enableAlwaysScrollToZoom", true) );
Zooming with the API
Different settings related to zooming can be set using the API.
Changing Zoom Values
Zoom values can be passed in PSPDFKit.Configuration#initialViewState
when loading a document.
This example sets the initial value to PSPDFKit.ZoomMode.AUTO
(the default):
PSPDFKit.load({
initialViewState: new PSPDFKit.ViewState({
zoom: PSPDFKit.ZoomMode.AUTO
})
});
It’s also possible to set it to a specific zoom value:
PSPDFKit.load({ initialViewState: new PSPDFKit.ViewState({ zoom: 2 }) });
This value can be changed at any time after loading via instance.setViewState()
:
instance.setViewState((viewState) => viewState.set("zoom", 1.5));
Available values for PSPDFKit.ViewState#zoom
can be looked up in the API reference on PSPDFKit.ZoomMode
.
Changing Zoom Scale Increments
By default, each click on the zoom toolbar buttons increases or decreases the zooming scale by increments of 25 percent. To change this default zoom step, change the zoomStep
property of the viewState
object. Use a number greater than 1.
The example below changes the zoom step to 10 percent:
instance.setViewState((viewState) => viewState.set("zoomStep", 1.1));
Querying Current Zoom Values
The current zoom value can be queried with instance.currentZoomLevel
at any time.
Other dynamic values related to zooming available in the current instance are:
-
instance.minimumZoomLevel
— minimum zoom level attainable using the UI or the API -
instance.maximumZoomLevel
— maximum zoom level attainable using the UI or the API
API Zooming Helpers
The API provides some helpers to make programmatic zoom handling easier.
This example zooms in to the document by a single step via PSPDFKit.ViewState#zoomIn()
:
instance.setViewState((viewState) => viewState.zoomIn());
It has its counterpart, PSPDFKit.ViewState#zoomOut()
:
instance.setViewState((viewState) => viewState.zoomOut());
Finally, instance.jumpAndZoomToRect()
can zoom to any part of the document.
This example navigates to page 10 of the document and zooms in to the bounding box of an annotation, making it take the whole viewport size:
instance.jumpAndZoomToRect(10, annotation.boundingBox);
![]()
When the zoom value changes, the page background may be rendered again in order to keep showing a sharp image.
Preventing Zooming for Annotations
By default, each annotation’s appearance scales to match the page zoom. To change this behavior, the noZoom
flag can be set to true
to prevent the annotation from being magnified when zooming in. This is currently only enabled for StampAnnotation
and TextAnnotation
(with the exception of TextAnnotation
s with the callout
property set).
The example below creates a TextAnnotation
with the noZoom
flag set to true
:
await instance.create([ new PSPDFKit.Annotations.TextAnnotation({ pageIndex: 0, boundingBox: new PSPDFKit.Geometry.Rect({ left: 50, top: 150, width: 180, height: 25 }), text: { format: "plain", value: "Text with noZoom flag" }, noZoom: true }) ]);