What are configuration changes in Android?
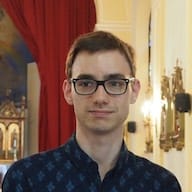
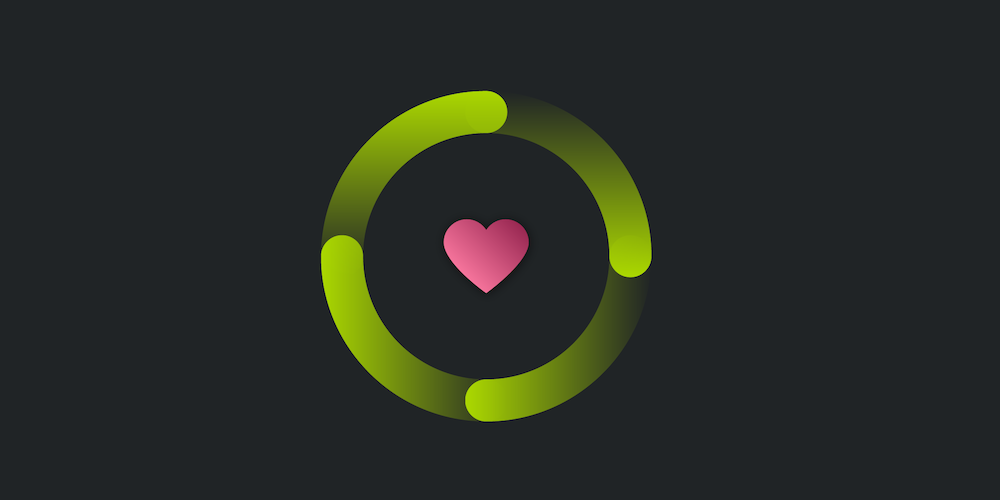
![]()
This article was first published in March 2019 and was updated in September 2024.
Configuration changes can sometimes be hard to understand. This blog post aims to shed some light on them and help you understand how to handle them effectively in modern Android development.
One of the most common causes of your app experiencing a configuration change is the user rotating their device. So let’s start by looking at how rotating the device impacts your app, followed by an overview of best practices for handling configuration changes.
How rotation on Android works
When a user rotates their device, Android handles orientation changes by destroying and recreating your Activity
. This means your Activity
will go through all the lifecycle events associated with being closed and then restarted, which can lead to the loss of any unpersisted data.
To prevent losing data, it’s essential to manage how your app responds to these configuration changes.
Handling configuration changes
Android provides a way for your application to opt out of being restarted when the orientation changes by defining android:configChanges
for your Activity
in the manifest. This tells the system that you’ll handle the specified configuration changes manually instead of relying on the system.
You can specify which changes to handle, such as orientation
and screenSize
. By doing this, when the user rotates their device, the system will call onConfigurationChanged()
with the new configuration, and your Activity
will remain alive.
Best practices
While using android:configChanges
can save time, it’s not the best practice for handling configuration changes in modern Android development. Instead, consider these approaches:
-
ViewModels — Use
ViewModels
from the Android Architecture Components. They’re designed to store and manage UI-related data in a lifecycle-conscious way, ensuring data survives configuration changes without needing to manually manage state. -
Jetpack Compose — If you’re using Jetpack Compose, it automatically handles configuration changes more gracefully. Composable functions are recomposed when configuration changes occur, making it easier to manage UI state without explicit lifecycle management.
-
Persistent storage — For critical data that must survive configuration changes or process death, consider using
SharedPreferences
, Room database, or any other form of persistent storage.
Advantages of handling configuration changes
Using android:configChanges
might seem beneficial because it allows your Activity
to maintain its state through rotation, resulting in a smoother user experience on slower devices. However, relying on this method can lead to potential issues down the line.
Disadvantages of handling configuration changes
The biggest disadvantage of using android:configChanges
is that you still need to implement onSaveInstanceState()
to save your app’s state when going into the background. This means you won’t save any work, since implementing onSaveInstanceState()
is still necessary for proper state management.
Moreover, when using android:configChanges
, you lose the automatic resource switching that Android provides. If your app needs different resources based on screen size or orientation, you must update your views in the onConfigurationChanged()
method, adding unnecessary complexity to your code.
// Activity.java @Override public void onConfigurationChanged(Configuration newConfig) { super.onConfigurationChanged(newConfig); // This is called after the device was rotated. if (newConfig.orientation == Configuration.ORIENTATION_LANDSCAPE) { // Do something landscape specific. } else { // Do something portrait specific. } }
Additionally, some parts of your application might rely on lifecycle events triggered during orientation changes, which won’t function correctly when using android:configChanges
.
Conclusion
While specifying android:configChanges
in the manifest may seem like a quick fix to handle orientation changes, it’s not the best solution for modern Android development. Leveraging ViewModels
and embracing Jetpack Compose can provide a more robust way to handle configuration changes while keeping your code clean and maintainable.
Ultimately, you cannot bypass the need for implementing onSaveInstanceState()
, regardless of whether or not you opt for android:configChanges
. It’s crucial to ensure your app maintains a seamless user experience, especially when faced with configuration changes.