FLUTTER PDF SDK
Create a better document experience in your Flutter app
Build cross-platform apps across iOS, Android, and the web using a Flutter PDF SDK designed to offer the best user and developer experience. Your apps need a powerful, flexible, and seamless PDF editing experience, and the Nutrient Flutter PDF library delivers advanced PDF editing, document security, and high-performance rendering in a package that’s easy to integrate and customize.
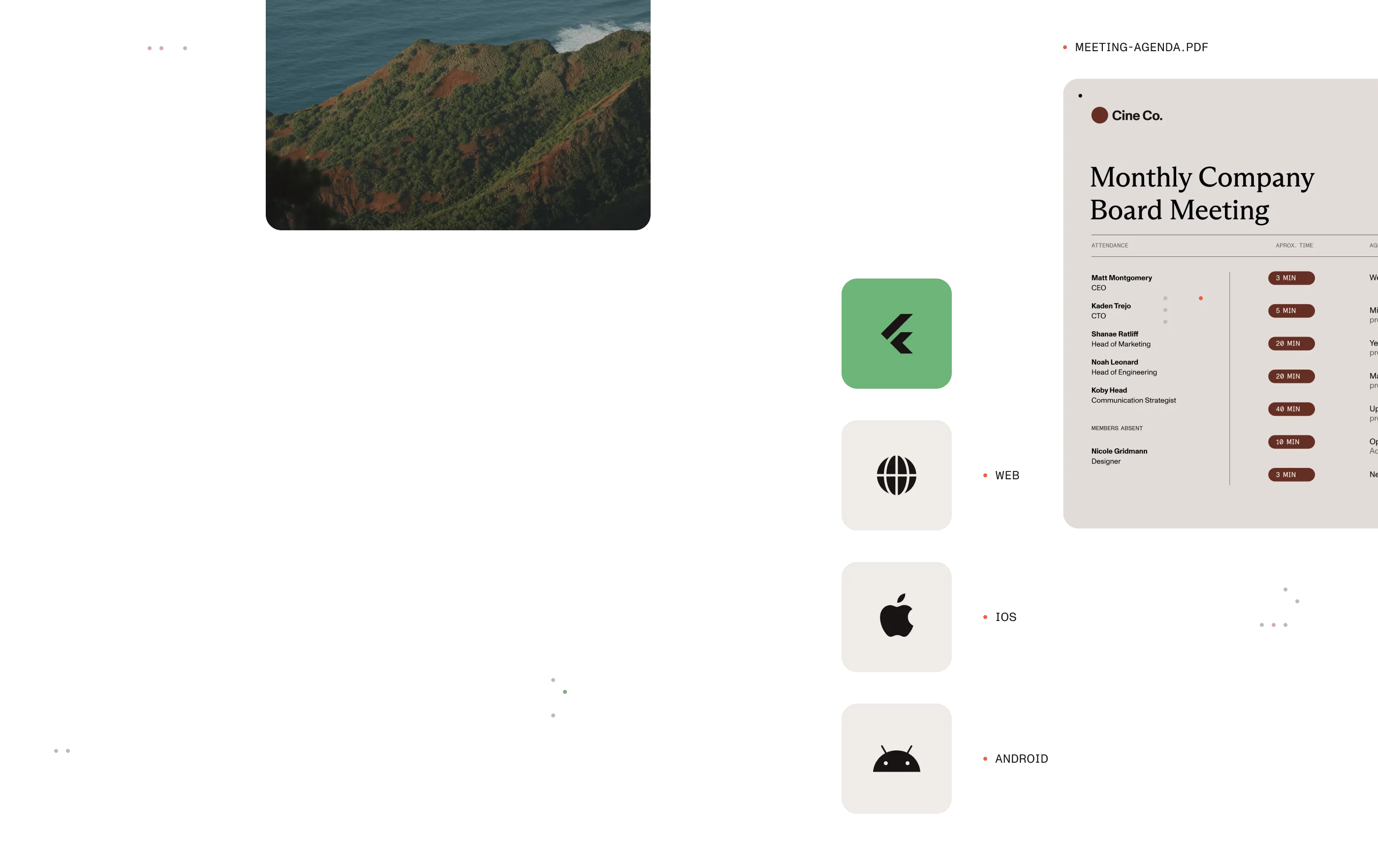
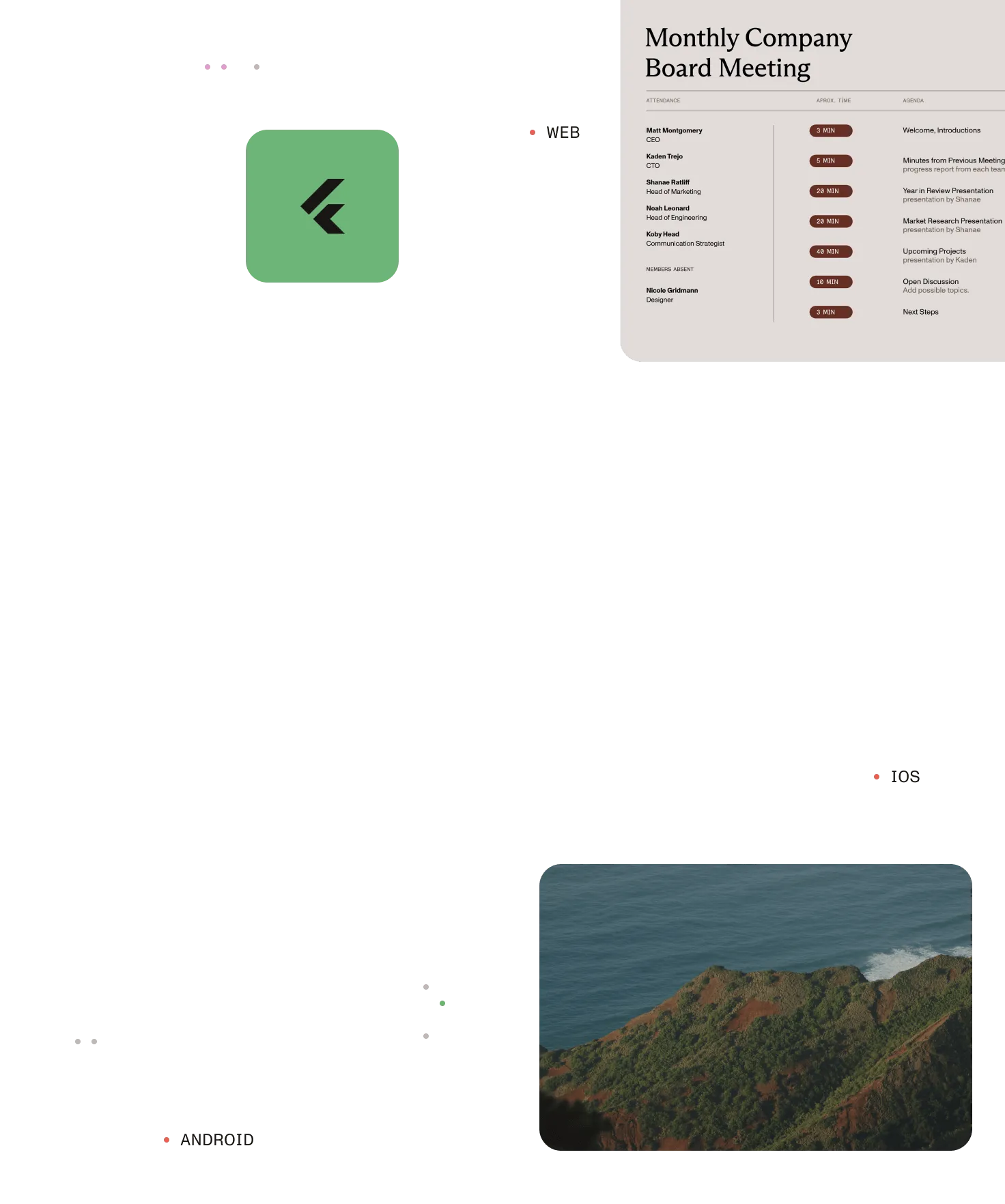

Cross-platform capabilities
Add document functionality to mobile and web apps from a single codebase
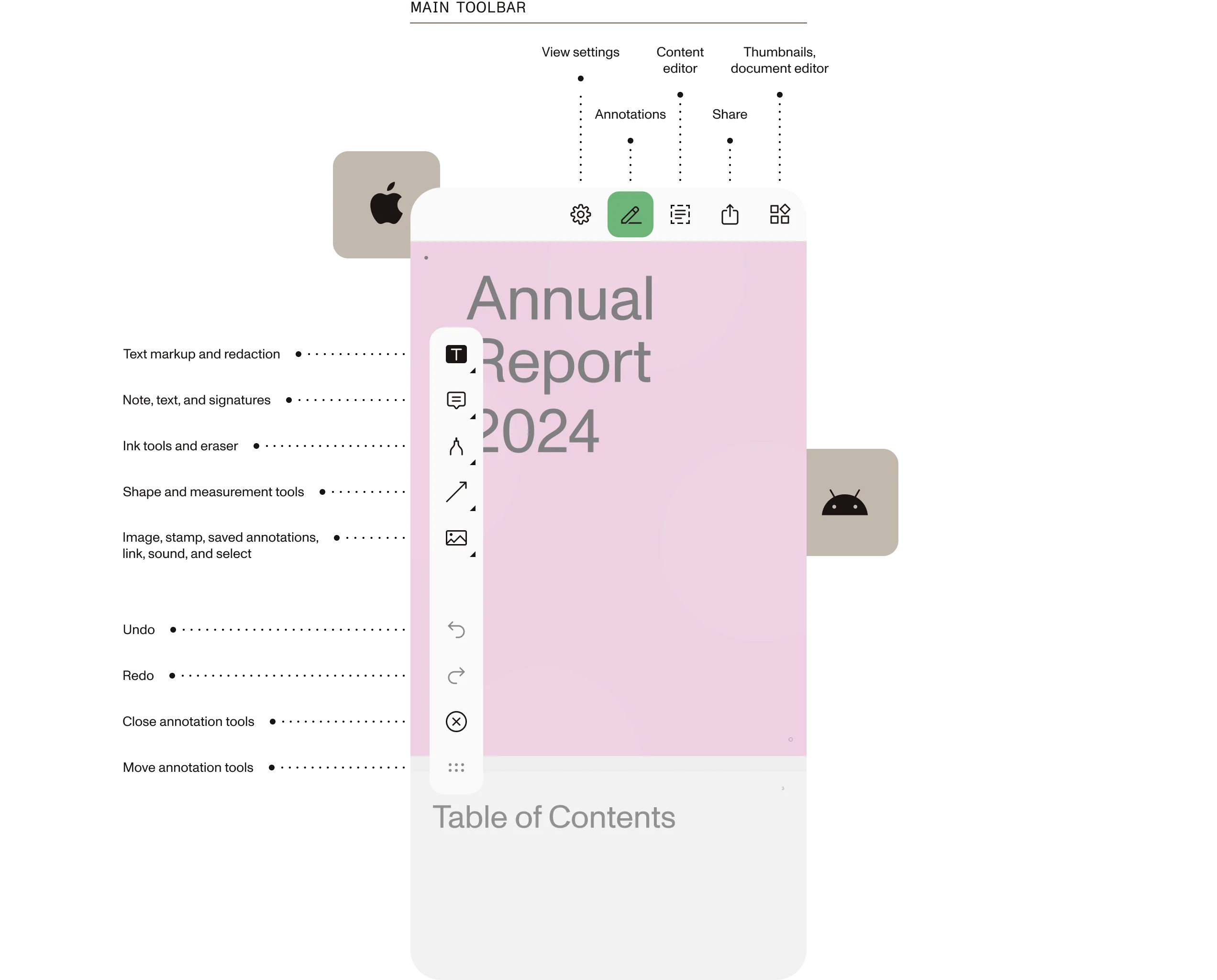
Benefits

Build faster
Go to market sooner with our easy setup process, detailed documentation, support resources, and ready-to-use code samples and templates. Developers can load, edit, and save PDFs with minimal setup using our Flutter plugin.

Save resources
Reduce the overhead costs associated with building and maintaining document technology internally across multiple applications. WIth our Flutter PDF editor, businesses can integrate PDF file management into their enterprise apps, allowing employees to sign and store documents efficiently.

Create consistency
Build comprehensive document functionality and a seamless user experience across iOS, Android, and the web. Our Flutter PDF SDK delivers high-performance rendering in a package that’s easy to integrate and customize.

Scale with security
Innovate with confidence knowing our SDKs are safe, reliable, and backed by a global support team that’s always ready to help. Secure and manage any PDF documents containing confidential medical records, financial statements, or compliance reports.
Relied upon by industry leaders
Example
Easy code integration
1import 'dart:io';
2
3import 'package:flutter/material.dart';
4import 'package:path_provider/path_provider.dart';
5import 'package:pspdfkit_flutter/pspdfkit.dart';
6
7const String DOCUMENT_PATH = 'PDFs/Document.pdf';
8
9void main() => runApp(MyApp());
10
11class MyApp extends StatefulWidget {
12 @override
13 _MyAppState createState() => _MyAppState();
14}
15
16class _MyAppState extends State<MyApp> {
17 void showDocument(BuildContext context) async {
18 final bytes = await DefaultAssetBundle.of(context).load(DOCUMENT_PATH);
19 final list = bytes.buffer.asUint8List();
20
21 final tempDir = await getTemporaryDirectory();
22 final tempDocumentPath = '${tempDir.path}/$DOCUMENT_PATH';
23
24 final file = await File(tempDocumentPath).create(recursive: true);
25 file.writeAsBytesSync(list);
26
27 await Pspdfkit.present(tempDocumentPath);
28 }
29
30 @override
31 Widget build(BuildContext context) {
32 final themeData = Theme.of(context);
33 return MaterialApp(
34 home: Scaffold(
35 body: Builder(
36 builder: (BuildContext context) {
37 return Center(
38 child: Column(
39 mainAxisAlignment: MainAxisAlignment.spaceEvenly,
40 children: [
41 ElevatedButton(
42 child: Text(
43 'Tap to Open Document',
44 style: themeData.textTheme.headline4?.copyWith(fontSize: 21.0),
45 ),
46 onPressed: () => showDocument(context),
47 ),
48 ],
49 ),
50 );
51 },
52 ),
53 ),
54 );
55 }
56}
File type support
PDF documents
image documents
jpeg
jpg
tiff
tif
Knowledge center
Pick your perfect solution
Nutrient is made to grow with your app. You can start with what you need now and add more products later. The Nutrient Flutter PDF editor is designed for developers who need robust security and compliance features beyond basic PDF viewing.
Viewing
Open all documents with high fidelity in a well-designed viewer.
Open all documents with high fidelity in a well-designed viewer.
Markup
Improve the review process with a suite of annotation tools.
Improve the review process with a suite of annotation tools.
Collaboration
Bring real-time collaboration to your documents.
Bring real-time collaboration to your documents.
Editing
Modify documents and easily edit PDF text directly in your app.
Modify documents and easily edit PDF text directly in your app.
Forms
Easy for users to fill forms. Easy for you to create them programmatically.
Easy for users to fill forms. Easy for you to create them programmatically.
Signing
Streamline contract execution and approval workflows by enabling eSignatures and PDF digital signatures.
Streamline contract execution and approval workflows by enabling eSignatures and PDF digital signatures.
Generation
Effortlessly generate PDFs from HTML and DOCX.
Effortlessly generate PDFs from HTML and DOCX.
OCR and Data Extraction
Give new life to scanned documents by easily converting them into text that's selectable and searchable.
Give new life to scanned documents by easily converting them into text that's selectable and searchable.
Document Conversion
Easily convert any Office document, image, email, or webpage into a high-quality PDF.
Easily convert any Office document, image, email, or webpage into a high-quality PDF.
Security and Compliance
Protect sensitive information and meet regulatory requirements within your application.
Protect sensitive information and meet regulatory requirements within your application.
Frequently asked questions
What is a Flutter PDF SDK?
A Flutter PDF SDK is a software development kit that enables developers to integrate PDF viewing, editing, and management capabilities into Flutter applications. It allows for the creation, modification, and rendering of PDF documents within both iOS and Android platforms from a single codebase.
Is the SDK compatible with both iOS and Android platforms?
Yes. The Nutrient Flutter PDF SDK is designed for cross-platform compatibility, allowing developers to deploy PDF functionality seamlessly on both iOS and Android devices from a single Flutter codebase.
Does the SDK support offline access to PDF documents?
Absolutely. The SDK enables offline access to PDF documents, allowing users to view and interact with PDFs without an active internet connection.
Can I customize the user interface of the PDF viewer?
Yes. The SDK provides extensive customization options for the PDF viewer’s user interface, enabling developers to tailor the appearance and functionality to align with their application’s design and user experience requirements.
How does the SDK handle large PDF files?
The Nutrient Flutter PDF SDK is optimized for performance, ensuring smooth loading and navigation, even with large PDF files. Features like lazy loading and efficient memory management contribute to handling extensive documents effectively.
Is there support for real-time collaboration on PDF documents?
While the SDK offers robust annotation and editing tools, implementing real-time collaboration requires additional backend support to manage concurrent user interactions. Developers can integrate this functionality depending upon their application’s architecture and requirements.
Latest from the blog
Blog