react native pdf sdk
Add document support to your React Native apps
Work with a React Native PDF viewer SDK engineered for the best possible user and developer experience. Whether you need high-fidelity PDF rendering, annotation tools, digital signatures, or form filling, our React Native PDF SDK gives you complete control over PDF viewing and editing within your app.
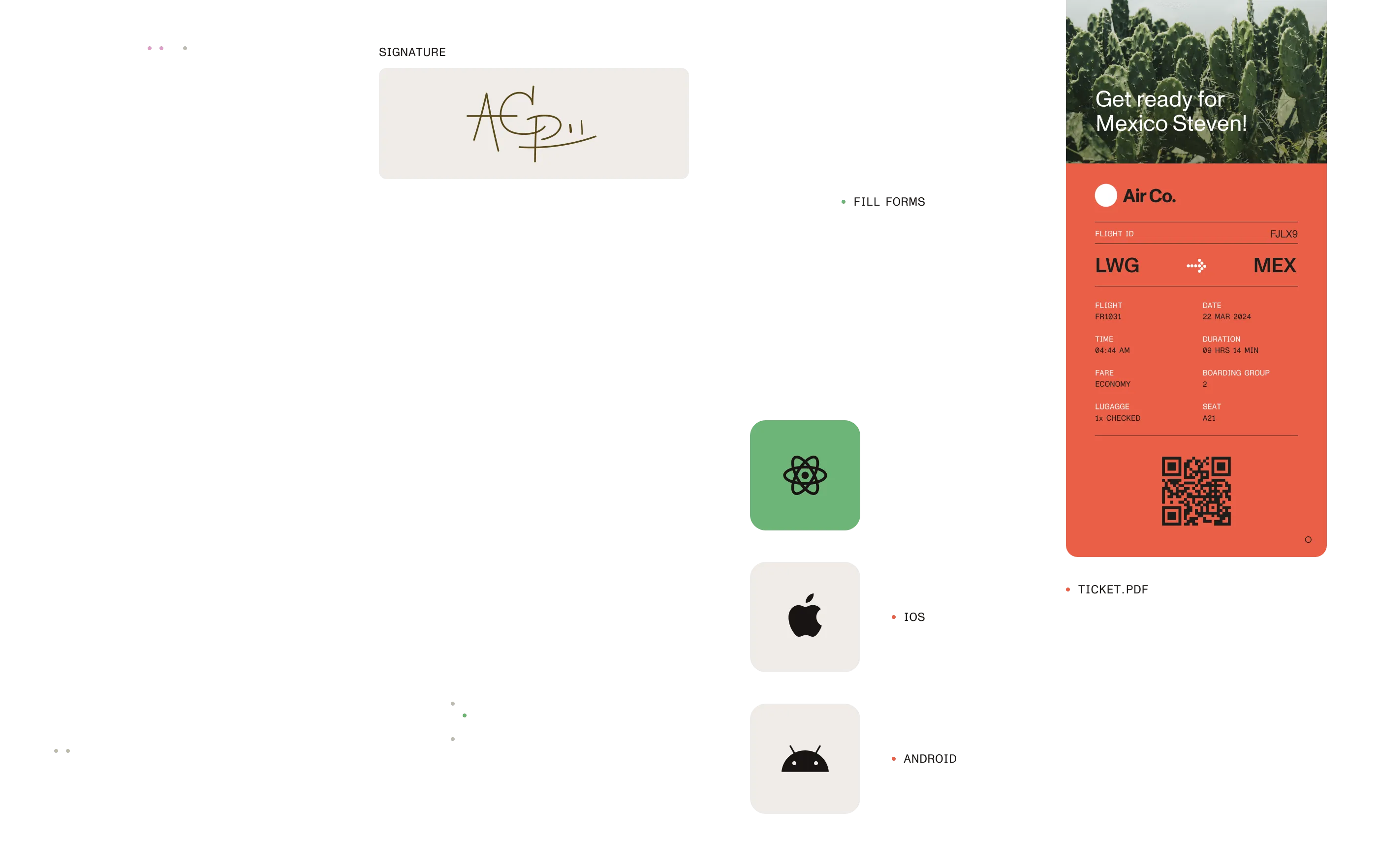
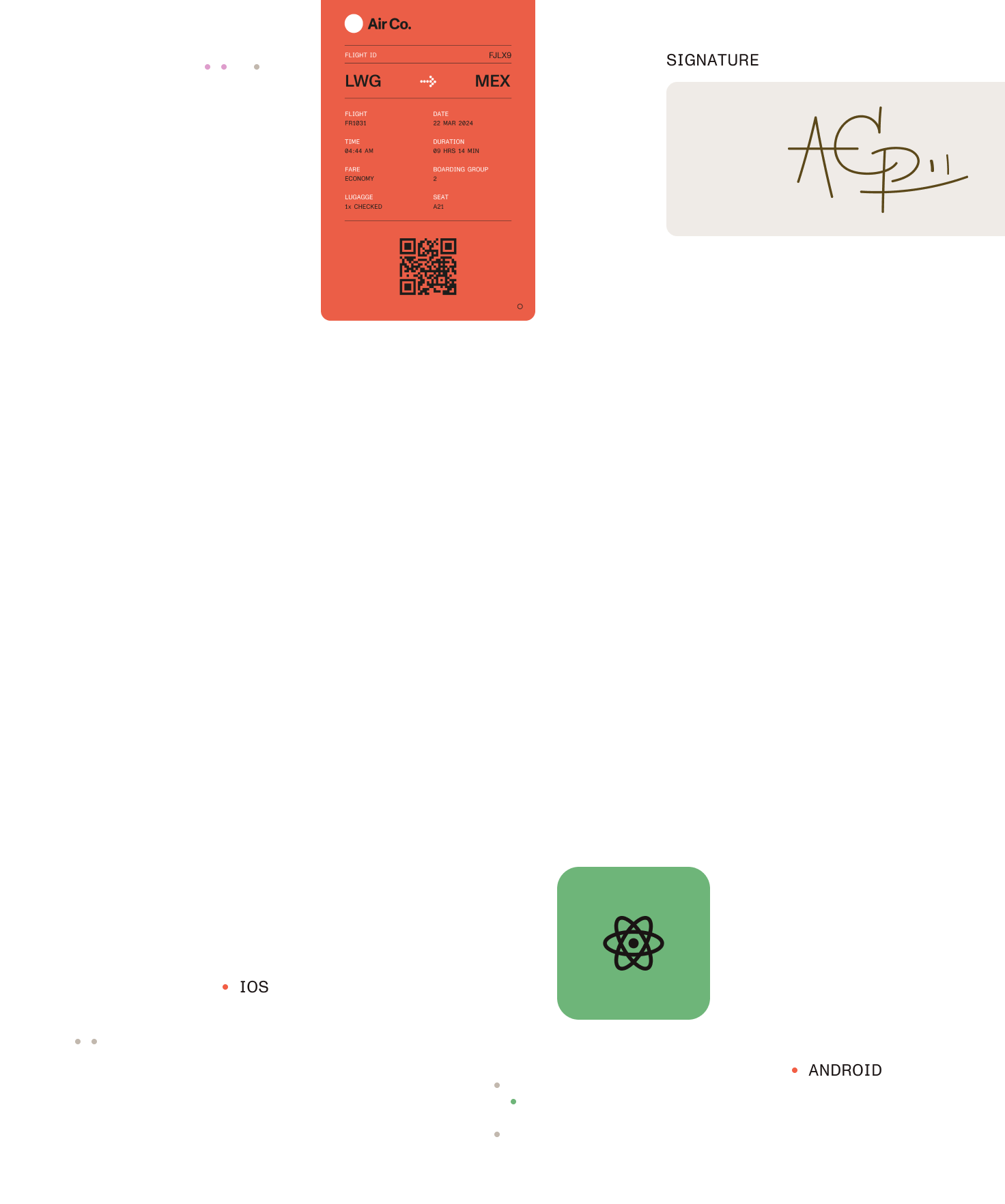
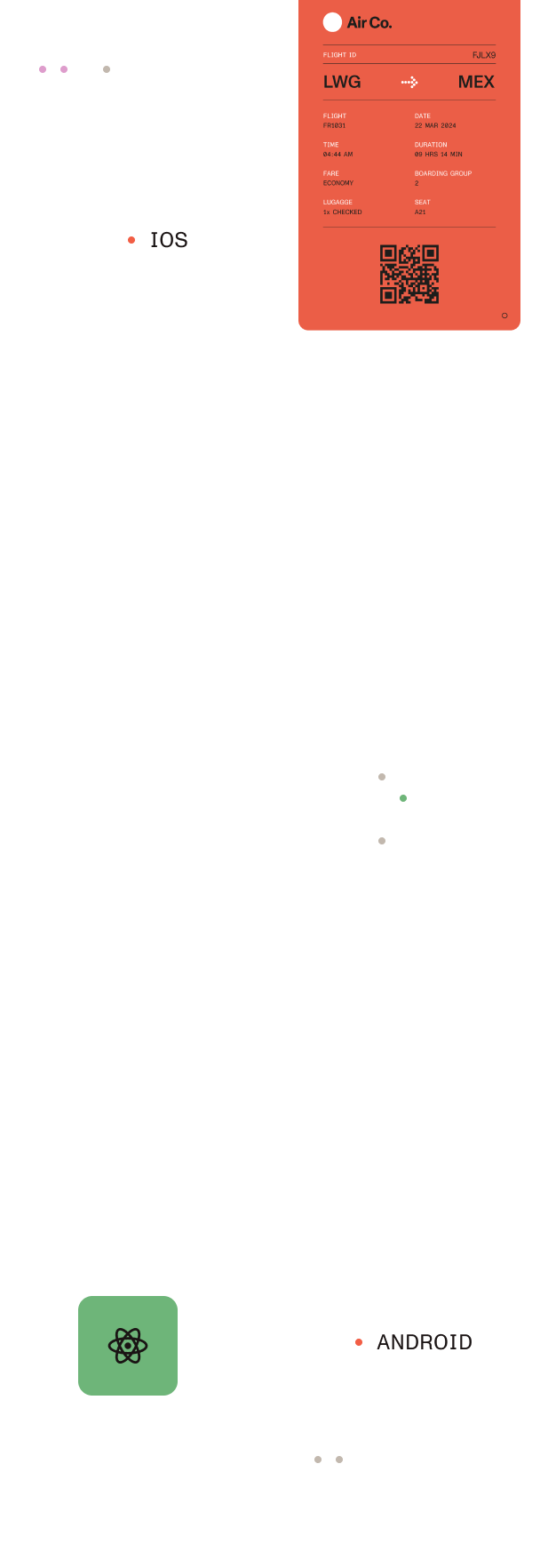
Benefits

Build faster
Go to market sooner with our easy setup process, detailed documentation, support resources, and ready-to-use code samples and templates. Unlike basic libraries, which often rely on WebView-based rendering, Nutrient’s React Native PDF SDK uses a fully native rendering engine, ensuring smooth scrolling, zooming, and text search.

Save resources
Reduce the overhead costs associated with building, customizing, and maintaining document technology internally across multiple applications. With a lightweight package, our React Native SDK allows developers to start working with PDFs immediately without dealing with unnecessary complexity.

Create consistency
Build a smooth experience across platforms, ensuring the user interface and functionality are the same across each touchpoint. For businesses that require enterprise-grade document management, our React Native PDF SDK supports real-time collaboration, allowing multiple users to annotate, comment on, and review documents simultaneously.

Scale with security
Innovate with confidence knowing that our SDKs are safe, reliable, and supported by a global, proven support team that’s always ready to help. When working with sensitive documents, developers can even lock down access to PDFs, restrict editing permissions, and prevent unauthorized modification with our React Native library.
trusted by industry leaders
Example
Easy code integration
1import React, {Component} from 'react';
2import {Platform} from 'react-native';
3import PSPDFKitView from 'react-native-pspdfkit';
4import { NativeModules } from 'react-native';
5
6const PSPDFKit = NativeModules.PSPDFKit;
7PSPDFKit.setLicenseKey(null);
8
9const DOCUMENT =
10 Platform.OS === 'ios'
11 ? 'Document.pdf'
12 : 'file:///android_asset/Document.pdf';
13export default class PSPDFKitDemo extends Component<{}> {
14 render() {
15 var pdfRef: React.RefObject<PSPDFKitView> = React.createRef();
16 return (
17 <PSPDFKitView
18 document={DOCUMENT}
19 ref={pdfRef}
20 fragmentTag="PDF1"
21 style={{flex: 1}}
22 />
23 );
24 }
25}
Knowledge center
Pick your perfect solution
Nutrient SDKs are made to grow with your app. You can start with what you need now and add more tools later. With full support for Android, iOS, and web, the Nutrient developer library is the best choice for those who require a powerful, scalable, and easy-to-integrate React Native PDF SDK.
Viewing
Open all documents with high fidelity in a well-designed viewer.
Open all documents with high fidelity in a well-designed viewer.
Markup
Improve the review process with a suite of annotation tools.
Improve the review process with a suite of annotation tools.
Collaboration
Bring real-time collaboration to your documents.
Bring real-time collaboration to your documents.
Editing
Modify documents and easily edit PDF text directly in your app.
Modify documents and easily edit PDF text directly in your app.
Forms
Easy for users to fill forms. Easy for you to create them programmatically.
Easy for users to fill forms. Easy for you to create them programmatically.
Signing
Streamline contract execution and approval workflows by enabling eSignatures and PDF digital signatures.
Streamline contract execution and approval workflows by enabling eSignatures and PDF digital signatures.
Generation
Effortlessly generate PDFs from HTML and DOCX.
Effortlessly generate PDFs from HTML and DOCX.
OCR and Data Extraction
Give new life to scanned documents by easily converting them into text that's selectable and searchable.
Give new life to scanned documents by easily converting them into text that's selectable and searchable.
Document Conversion
Easily convert any Office document, image, email, or webpage into a high-quality PDF.
Easily convert any Office document, image, email, or webpage into a high-quality PDF.
Security and Compliance
Protect sensitive information and meet regulatory requirements within your application.
Protect sensitive information and meet regulatory requirements within your application.
Frequently asked questions
What is the Nutrient React Native PDF SDK?
The Nutrient React Native PDF SDK is a comprehensive toolkit that enables developers to seamlessly integrate advanced PDF viewing and editing capabilities into React Native applications. It supports features such as high-fidelity rendering, annotation tools, digital signatures, and form filling, providing a rich user experience.
How do I integrate the Nutrient PDF SDK into my React Native project?
Integration involves installing the SDK via npm or yarn, linking native dependencies, and configuring the SDK within your React Native project. Detailed setup guides and documentation are available to assist developers through the process.
What platforms does the SDK support?
The Nutrient React Native PDF SDK supports both iOS and Android platforms, ensuring consistent PDF functionality across devices.
Can the SDK handle PDF annotations?
Yes. The SDK provides robust annotation capabilities, allowing users to add, edit, and remove annotations such as highlights, notes, and drawings within PDF documents.
Does the SDK support form filling in PDFs?
Absolutely. Users can fill out interactive PDF forms, extract form data, and programmatically manipulate form fields using the SDK.
How does the SDK handle document rendering?
The SDK ensures high-fidelity rendering of PDF documents, providing a smooth and accurate viewing experience across supported platforms.
Are there customization options for the viewer UI?
Yes. Developers can customize the user interface of the PDF viewer to align with their app’s design and user experience requirements.
Can the SDK be used in offline scenarios?
Yes. The SDK supports offline usage, allowing users to access and interact with PDF documents without an active internet connection.
React Native PDF SDK
What is a React Native PDF SDK?
A React Native PDF SDK allows developers to easily add powerful PDF viewing and editing features directly into React Native applications. Rather than building functionality from scratch, developers leverage the SDK to quickly implement essential tools like viewing PDFs, annotating documents, digitally signing forms, and securely managing document workflows.
- Easily integrates PDF capabilities into mobile apps.
- Includes high-quality rendering and smooth user experiences.
- Enables annotation, form filling, digital signatures, and more.
- Simplifies complex document-handling tasks.
How to choose the right React Native PDF SDK
Selecting the best React Native PDF SDK involves considering several key factors that ensure the solution meets your project’s unique needs. Pay special attention to:
- Performance — Choose an SDK with native rendering capabilities for smooth, lag-free user experiences.
- Feature sets — Prioritize comprehensive features like annotations, signatures, form handling, and collaboration tools.
- Integration and documentation — Look for extensive documentation, easy setup, and robust developer support.
- Security and compliance — Verify that the SDK has strong security measures and compliance with industry standards for handling sensitive documents.
What are the best solutions to solve my React Native PDF problem?
Several solutions exist, but the optimal React Native PDF SDK solutions typically offer:
- Fully native rendering engines rather than WebView-based rendering
- Rich, built-in annotation and editing tools
- Robust support for digital signatures and form filling
- Real-time collaboration capabilities for document reviews
- Comprehensive security features for sensitive information handling
What are the benefits of a React Native PDF SDK with Nutrient?
Choosing Nutrient's React Native PDF SDK means getting a product crafted for developers who demand reliability, simplicity, and scalability:
- High performance — Nutrient uses a native rendering engine, ensuring high-fidelity document views and seamless navigation.
- Rapid integration — Accelerate your app development with extensive guides, ready-to-use code samples, and detailed documentation.
- Cost-efficient — Reduce development and maintenance overhead with a lightweight SDK designed for immediate deployment.
- Enterprise-ready — Built-in features such as annotation synchronization, document security, and compliance controls make it ideal for enterprise-level use.
- Expert support — Global, responsive support to resolve issues quickly and keep your projects running smoothly.
How easy is it to integrate Nutrient’s React Native PDF SDK?
Nutrient ensures integration into your React Native projects is straightforward and hassle-free, with:
- Clearly documented setup processes
- Simple, intuitive APIs
- Comprehensive guides for a quick start
- Prebuilt examples and templates ready for immediate use
Latest from the blog
Blog