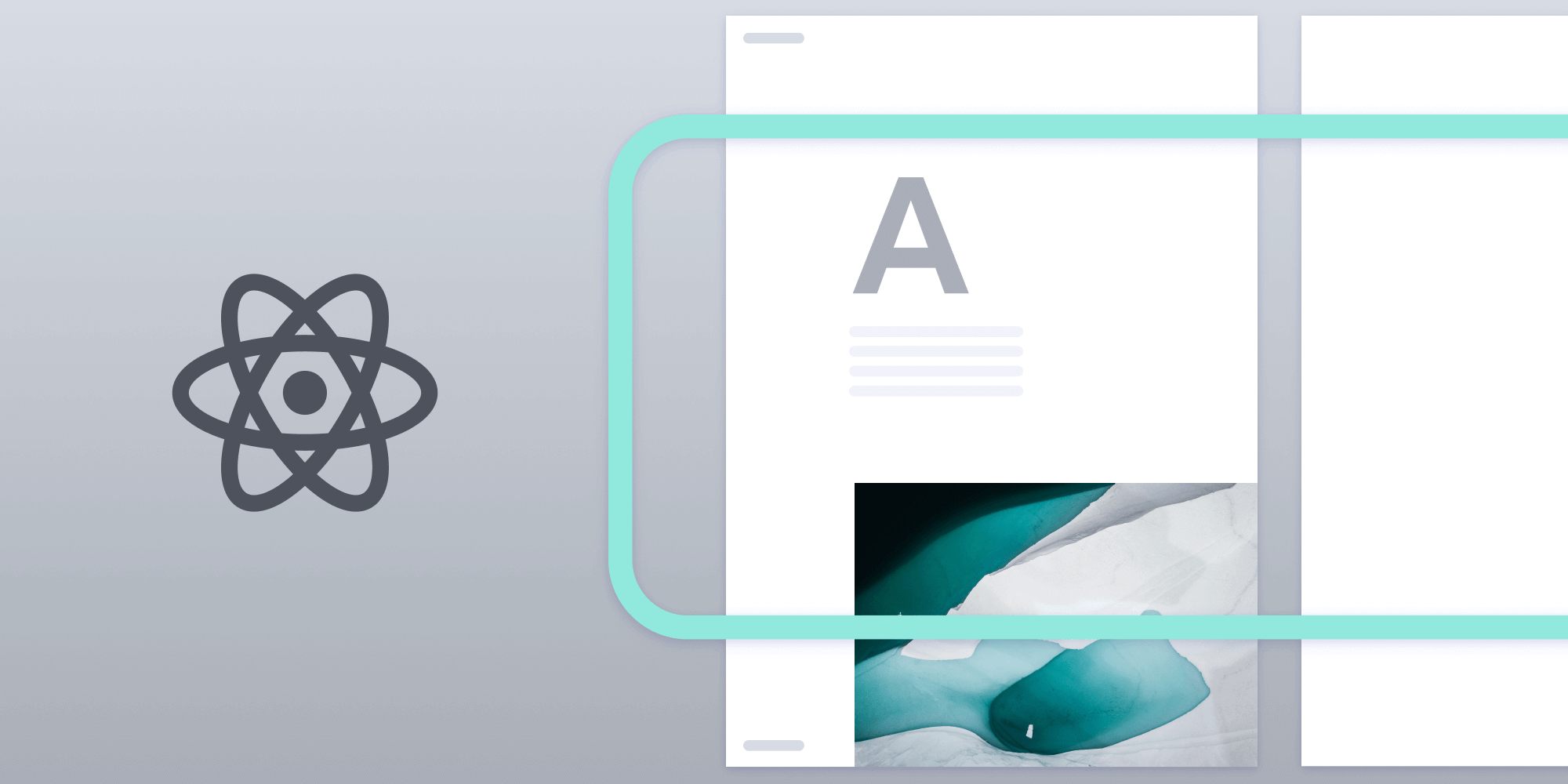
Integrating PDF functionality into mobile applications is crucial for enhancing user experience and providing essential document management features. PDFs are widely used across various industries such as education, finance, healthcare, and legal services. With the increasing demand for mobile-friendly document access, offering PDF viewing capabilities can significantly boost an app’s value proposition.
Common use cases include:
-
ebooks and digital publishing — Providing seamless access to digital content with interactive features.
-
Business reports and invoices —Allowing professionals to view and share reports on the go.
-
Document management systems — Facilitating access to important contracts, forms, and records.
By implementing PDF functionality, developers can cater to a broad audience, ensuring document accessibility and enhancing productivity.
This article will show you how to set up a PDF viewer using two different libraries: the open source react-native-pdf
, and the commercial Nutrient React Native PDF SDK .
You’ll learn how to create a new project, add the necessary dependencies, configure platform-specific settings, and display a PDF document. By the end of this tutorial, you’ll have a fully functional PDF viewer in your React Native app.
Introduction to React Native PDF viewers
React Native PDF viewers are essential components for mobile applications that require displaying PDF files. These viewers enable users to interact with PDF documents directly within the app, providing a seamless user experience. With features like zooming, scrolling, and page navigation, React Native PDF viewers make it easy for users to navigate through PDF files. They support various PDF formats, including PDF 1.7 and later versions, ensuring compatibility with a wide range of documents. By integrating a native PDF viewer into your React Native project, you can enhance your app’s functionality and offer users a more comprehensive document handling experience.
Why add PDF support to your app?
Adding PDF support to your app enables it to handle various document needs, from basic viewing, to advanced functionalities like annotations, form filling, and digital signatures. Choosing the right library can help you implement features that are essential for your app’s success.
React Native PDF viewer use case
This post assumes you’re familiar with React Native and that you’ve previously developed apps using React Native. If that isn’t the case, you can use our getting started guides to create a new React Native project that uses Nutrient. Or, if you’re in a hurry, you can download our ready-to-run example project and follow the instructions from its README.
In the video below, you can see how we open a PDF file using react-native-pdf
and Nutrient React Native SDK.
Now, let’s take a look at the steps involved!
Prerequisites
Before getting started, ensure you have the following:
-
A development environment for React Native using the React Native CLI (not the Expo CLI)
-
The latest stable versions of Android Studio, the Android NDK, and an Android Virtual Device or hardware device
Platform-specific configuration
Android configuration
-
Ensure compatibility with different Android SDK versions.
-
Common issues and solutions:
-
Gradle build failures — Ensure proper dependency versions in
build.gradle
. -
Permissions — Add required permissions in
AndroidManifest.xml
for file access.
-
iOS configuration
-
Setting up permissions:
-
Add necessary permissions in
Info.plist
for document access.
-
-
Handling device orientations:
-
Ensure the app supports all orientations by modifying the
UISupportedInterfaceOrientations
key.
-
-
Performance optimizations:
-
Enable caching for faster load times.
-
Step 1 — Creating a new React Native project
Open your terminal and navigate to the directory where you want to create your project. For this example, you’ll use the ~/Documents/
directory:
cd ~/Documents
Create a new React Native project:
npx react-native@latest init ReactNativePDFViewer
Next, you’ll add the required dependencies.
Step 2 — Installing the Nutrient dependency
-
Navigate to your project directory:
cd ReactNativePDFViewer
-
Then, add the Nutrient dependency:
yarn add react-native-pspdfkit@github:PSPDFKit/react-native
-
Install all the dependencies for the project:
yarn install
Step 3 — Configuring the Android platform
-
Open
android/build.gradle
and add the Nutrient repository:
allprojects {
repositories {
mavenCentral()
+ maven { url 'https://my.nutrient.io/maven/' }
}
}
-
Open
android/app/build.gradle
and update the SDK versions:
android { - compileSdkVersion rootProject.ext.compileSdkVersion + compileSdkVersion 34 defaultConfig { applicationId "com.reactnativepdfviewer" - minSdkVersion rootProject.ext.minSdkVersion + minSdkVersion 21 targetSdkVersion rootProject.ext.targetSdkVersion } }
Step 4 — Configuring the iOS platform
-
Open
ios/Podfile
and set the platform version to iOS 15:
- platform :ios, '11.0' + platform :ios, '15.0'
-
Navigate to the
ios
folder and install the required dependencies:
cd ios pod install
-
Open the Workspace in Xcode:
open ReactNativePDFViewer.xcworkspace
-
In Xcode, ensure the deployment target is set to 15.0 or higher.
-
Change View controller-based status bar appearance to
YES
inInfo.plist
.
Step 5 — Displaying a PDF document
-
Drag a PDF document into your project to use as a sample.
-
If you haven’t already, create an assets directory for Android:
mkdir android/app/src/main/assets
-
Place your PDF document in the
assets
directory:
cp ~/Downloads/Document.pdf android/app/src/main/assets/Document.pdf
-
Open
App.js
and replace its contents with the following code snippet:
import React, { Component } from 'react'; import { Platform } from 'react-native'; import PSPDFKitView from 'react-native-pspdfkit'; import { NativeModules } from 'react-native'; const PSPDFKit = NativeModules.PSPDFKit; PSPDFKit.setLicenseKey(null); const DOCUMENT = Platform.OS === 'ios' ? 'Document.pdf' : 'file:///android_asset/Document.pdf'; export default class PSPDFKitDemo extends Component<{}> { render() { var pdfRef: React.RefObject<PSPDFKitView> = React.createRef(); return ( <PSPDFKitView document={DOCUMENT} configuration={{ showThumbnailBar: 'scrollable', pageTransition: 'scrollContinuous', scrollDirection: 'vertical', }} ref={pdfRef} fragmentTag="PDF1" style={{ flex: 1 }} /> ); } }
Step 6 — Launching the app
Run the app on your chosen platform:
npx react-native run-android npx react-native run-ios
Detailed comparison of libraries
When integrating PDF functionality in a React Native project, two popular libraries to consider are react-native-pdf
and the Nutrient React Native PDF SDK. Below is a detailed comparison to help you make an informed decision.
Feature | react-native-pdf | Nutrient React Native PDF SDK |
---|---|---|
Cost | Free (open source) | Paid (commercial) |
Features | Basic viewing, zooming | Advanced features (annotations, search, forms) |
Performance | Moderate | Optimized for high performance |
Ease of integration | Simple | Requires licensing setup |
Customization options | Limited | Extensive UI customization |
Community support | Active, open source | Professional support available |
Platform compatibility | Android, iOS | Android, iOS |
Recommendation:
-
Choose
react-native-pdf
for basic viewing needs with minimal setup and no cost. -
Opt for the Nutrient React Native PDF SDK if you require advanced features, customization, and professional support.
Choosing between these libraries depends on the project requirements, budget, and desired feature set.
Advanced features of Nutrient React Native PDF SDK vs. limitations of react-native-pdf
Sometimes, your business may require more complex features for handling PDFs in your React Native project, such as annotations, interactive forms, digital signatures, and long-term support. While the react-native-pdf
library provides basic PDF viewing capabilities, it lacks support for more advanced features. Below is a comparison of react-native-pdf
limitations and the capabilities of the Nutrient React Native PDF SDK.
1. Annotation support
-
Limitations of
react-native-pdf
—react-native-pdf
only renders annotations that were already in the source file. It doesn’t support annotation editing, so users won’t be able to create, update, or delete annotations in the PDF. -
Nutrient SDK features — Nutrient’s React Native SDK supports comprehensive annotation capabilities, allowing you to add, edit, and remove various types of annotations programmatically or via the user interface. It supports 17 annotation types, including highlights, text, ink, notes, and shapes. You can also import and export annotations in XFDF or JSON formats, with advanced features like cloudy annotations and custom appearances.
2. Interactive PDF forms
-
Limitations of
react-native-pdf
—react-native-pdf
only renders forms and their values from the source file and doesn’t support form editing. Users won’t be able to fill forms in a PDF document. -
Nutrient SDK features — Nutrient’s React Native SDK provides full support for interactive PDF forms, allowing users to fill, read, and edit PDF AcroForms programmatically or through the UI. You can capture form field data, export it, or embed it into the PDF.
3. Digital signatures
-
Limitations of
react-native-pdf
—react-native-pdf
currently has no support for digital signatures, which are essential for verifying the authenticity of filled-out PDFs. -
Nutrient SDK features — Nutrient React Native SDK includes support for adding electronic and digital signatures to PDF documents, ensuring a document’s authenticity.
4. Customizing the PDF viewer UI
-
Limitations of
react-native-pdf
— The customization options for the PDF viewer inreact-native-pdf
are limited compared to the Nutrient SDK. -
Nutrient SDK features — Nutrient offers extensive customization of the PDF viewer UI. You can modify the toolbar, adjust the background color, and configure the layout to match your app’s design. The viewer supports multiple page modes and transitions, ensuring the UI aligns with your app’s user experience.
5. Long-term support and updates
-
Limitations of
react-native-pdf
— The latest update forreact-native-pdf
was released in October 2020, and it has limited support for new features and updates. -
Nutrient SDK features — The Nutrient SDK is regularly updated to add new features, fix bugs, and maintain compatibility with the latest React Native versions, operating system updates, and dependencies. This ensures your app stays up to date with the latest PDF handling capabilities.
Optimizing performance of the PDF viewer in React Native
To optimize the performance of the PDF viewer in React Native applications using Nutrient SDK, follow the best practices outlined below.
-
Optimize PDF documents
Before rendering, optimize your PDF documents for mobile viewing by reducing image sizes to save memory, avoiding JPEG 2000 for image compression due to its complexity, and using tools like Adobe Acrobat to optimize PDFs for mobile devices.
-
Implement caching
Improve performance for recurring users by implementing a caching strategy, such as using service workers to precache resources and implementing client-side caching for faster interactions.
-
Use Reader View
For text-heavy documents, consider using the Reader View feature, which presents content in an easy-to-read, single-column view optimized for mobile devices. It ignores non-essential elements like images and headers, improving reading flow and enhancing performance.
-
Configure page transitions
Optimize page transitions for smoother scrolling by using continuous scrolling modes, which ensure seamless navigation between pages.
-
Lazy loading
Consider implementing lazy loading techniques to load only the visible pages and their immediate neighbors, reducing memory usage and improving initial load times.
-
Optimize UI rendering
Customize the UI to show only necessary elements by hiding unnecessary toolbar buttons to reduce UI complexity and using the PSPDFKitView
component to adjust the layout of the native UI component for optimal performance.
-
Use the latest version
Always use the latest version of Nutrient React Native SDK, as it likely includes performance improvements and bug fixes.
These optimizations should be applied thoughtfully based on your specific use case and the types of PDF documents you’re working with.
Conclusion
We hope this post will help you build a PDF viewer using react-native-pdf
and Nutrient React Native SDK in your React Native project. As you can see, react-native-pdf
is a great solution for many use cases. However, it has limitations when it comes to advanced PDF features like annotations, form filling, and digital signatures.
If your business relies on any of the features above, consider looking into alternatives. At Nutrient, we offer a commercial, feature-rich, and completely customizable React Native PDF viewer library that’s easy to integrate and comes with well-documented APIs to handle advanced use cases. Check out our demos to see it in action.
If you have any questions about our React Native PDF library, please don’t hesitate to reach out to us. We’re happy to help!
FAQ
What is the best library for building a React Native PDF viewer?
Two popular libraries for building a PDF viewer in React Native arereact-native-pdf
and Nutrient React Native SDK. react-native-pdf
is an open source library suitable for basic PDF viewing needs, while Nutrient offers a comprehensive commercial solution with advanced features like annotations, form filling, and digital signatures.
How can I display PDF files in a React Native app?
To display PDF files in a React Native app, you can use thereact-native-pdf
library for basic PDF rendering or Nutrient for a more feature-rich experience. Both libraries support loading and viewing PDF documents on iOS and Android platforms. The setup involves installing the required dependencies, adding the PDF file to your project, and using components from these libraries to display the file.
How do I add annotations to PDFs in React Native?
react-native-pdf
only renders existing annotations in a PDF but doesn’t support creating or editing them. For full annotation support, including adding, modifying, and deleting annotations, you can use Nutrient React Native SDK, which provides a robust API and tools for advanced PDF annotation capabilities.
Can I fill out forms in a PDF document using React Native?
Yes, but only with certain libraries. Whilereact-native-pdf
will display forms as they appear in the PDF, it doesn’t support interactive form filling. Nutrient React Native SDK offers complete form handling, allowing users to fill out interactive PDF forms directly within the app.
What are the advantages of using Nutrient React Native SDK?
Nutrient React Native SDK provides several advanced features not available inreact-native-pdf
, such as digital signatures, annotation editing, form filling, and long-term support with regular updates. It’s an ideal choice for apps that require robust PDF handling capabilities beyond basic viewing.