How to Delete PDF Pages Using Java
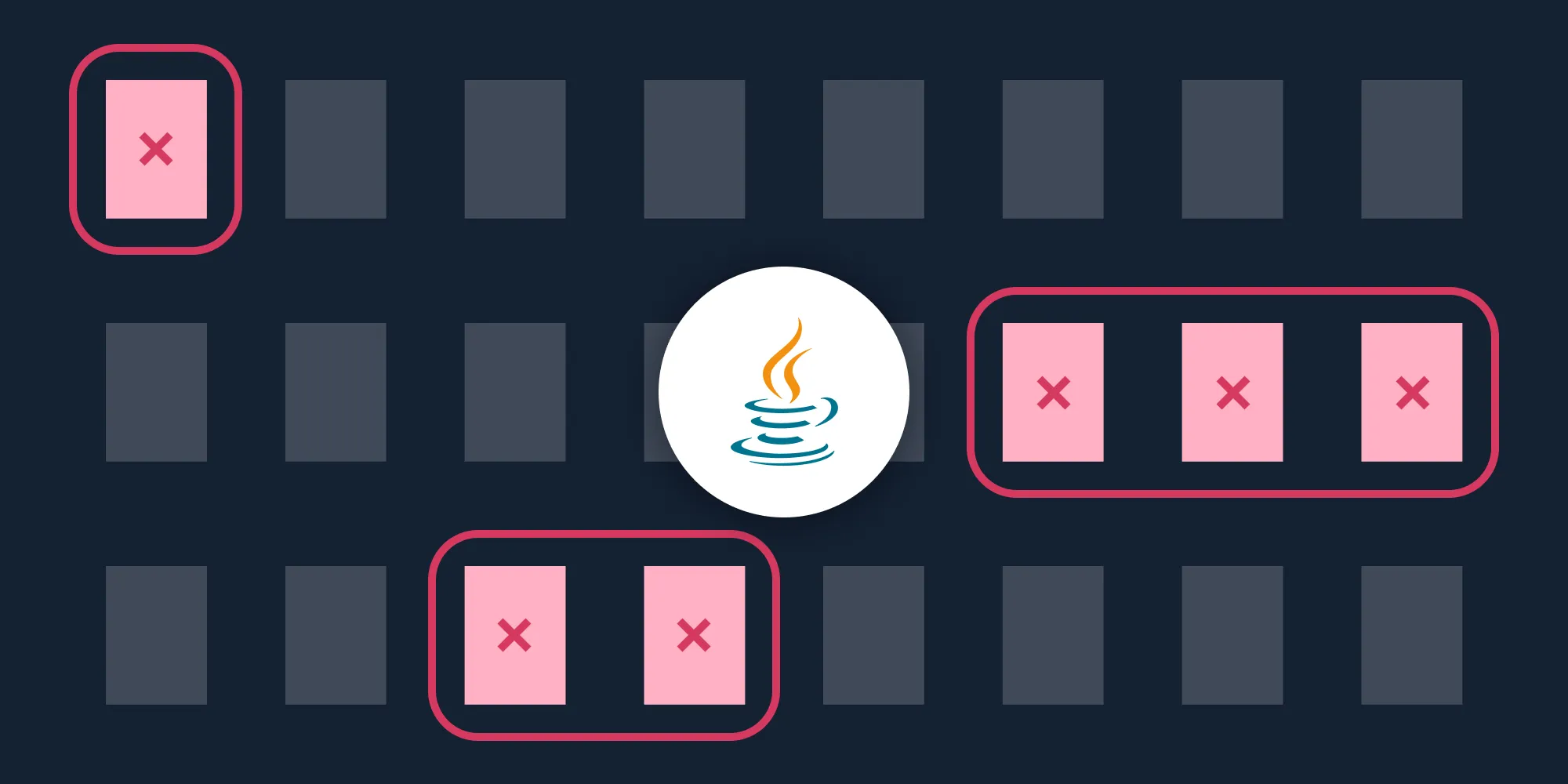
In this post, you’ll learn how to delete PDF pages using our Delete PDF Page Java API(opens in a new tab). With our API, you receive 100 credits with the free plan. Different operations on a document consume different amounts of credits, so the number of PDF documents you can generate may vary. You’ll just need to create a free account(opens in a new tab) to access your API key.
This post will be especially helpful for developers working with Java in document-heavy workflows who need to programmatically remove pages from a PDF to delete confidential data, save storage space, or remove information that’s unnecessary for end users.
PSPDFKit API
Deleting PDF pages is just one of the operations possible with our 30+ PDF API tools(opens in a new tab). You can combine our deletion tool with other tools to create complex document processing workflows, such as:
- Converting MS Office files and images into PDFs before removing pages
- Removing pages from two documents before merging them
- Deleting pages and then watermarking and flattening PDFs
Once you create your account, you’ll be able to access all our PDF API tools.
Step 1 — Creating a Free Account on PSPDFKit
Go to our website(opens in a new tab), where you’ll see the page below, prompting you to create your free account.
Once you’ve created your account, you’ll be welcomed by the page below, which shows an overview of your plan details.
As you can see in the bottom-left corner, you’ll start with 100 credits to process, and you’ll be able to access all our PDF API tools.
Step 2 — Obtaining the API Key
After you’ve verified your email, you can get your API key from the dashboard. In the menu on the left, click API Keys. You’ll see the following page, which is an overview of your keys:
Copy the Live API Key, because you’ll need this for the Delete PDF Page API.
Step 3 — Setting Up Folders and Files
For this tutorial, you’ll use IntellIJ IDEA as your primary code editor. Now, create a new project called delete_pdf
. You can choose any location, but make sure to select Java as the language, Gradle as the build system, and Groovy as the Gradle DSL.
Create a new directory in your project. Right-click on your project’s name and select New > Directory. From there, choose the src/main/java
option. Once done, create a class file inside the src/main/java
folder called processor.java
, and create two folders called input_documents
and processed_documents
in the root folder.
After that, paste your PDF file inside the input_documents
folder.
Your folder structure will look like this:
delete_pdf├── input_documents| └── document.pdf├── processed_documents├── src| └── main| └── java| └── processor.java
Step 4 — Installing Dependencies
Next, you’ll install two libraries:
OkHttp — This library makes API requests.
JSON — This library will parse the JSON payload.
Open the build.gradle
file and add the following dependencies to your project:
dependencies { implementation 'com.squareup.okhttp3:okhttp:4.9.2' implementation 'org.json:json:20210307'}
Once done, click the Add Configuration button in IntelliJ IDEA. This will open a dropdown menu.
Next, select Application from the menu.
Now, fill the form with the required details. Most of the fields will be prefilled, but you need to select java 18
in the module field and add -cp delete_pdf.main
as the main class and com.example.pspdfkit.Processor
in the field below it.
To apply settings, click the Apply button.
Step 5 — Writing the Code
Now, open the processor.java
file and paste the code below into it:
package com.example.pspdfkit;
import java.io.File;import java.io.IOException;import java.nio.file.FileSystems;import java.nio.file.Files;import java.nio.file.StandardCopyOption;
import org.json.JSONArray;import org.json.JSONObject;
import okhttp3.MediaType;import okhttp3.MultipartBody;import okhttp3.OkHttpClient;import okhttp3.Request;import okhttp3.RequestBody;import okhttp3.Response;
public final class Processor { public static void main(final String[] args) throws IOException { final RequestBody body = new MultipartBody.Builder() .setType(MultipartBody.FORM) .addFormDataPart( "document", "document.pdf", RequestBody.create( new File("input_documents/document.pdf"), MediaType.parse("application/pdf") ) ) .addFormDataPart( "instructions", new JSONObject() .put("parts", new JSONArray() .put(new JSONObject() .put("file", "document") .put("pages", new JSONObject() .put("end", 2) ) ) .put(new JSONObject() .put("file", "document") .put("pages", new JSONObject() .put("start", 4) ) ) ).toString() ) .build();
final Request request = new Request.Builder() .url("https://api.pspdfkit.com/build") .method("POST", body) .addHeader("Authorization", "Bearer YOUR API KEY HERE") .build();
final OkHttpClient client = new OkHttpClient() .newBuilder() .build();
final Response response = client.newCall(request).execute();
if (response.isSuccessful()) { Files.copy( response.body().byteStream(), FileSystems.getDefault().getPath("processed_documents/result.pdf"), StandardCopyOption.REPLACE_EXISTING ); } else { // Handle the error. throw new IOException(response.body().string()); } }}
ℹ️ Note: Make sure to replace
YOUR_API_KEY_HERE
with your API key.
Code Explanation
In the code above, you’re importing all the packages required to run the code and creating a class called processor
. In the main function, you’re first creating the request body for the API call that contains all the instructions for deleting the PDF pages.
You’re then calling the API using OkHttpClient
and checking the status of the response. If the response is successful
, you’re storing result.pdf
in the processed_documents
folder.
Output
To execute the code, click the Run button (which is a little green arrow). This is next to the field that says Processor, which is where you set the configuration.
On successful execution, you’ll see the new PDF with the removed pages in the processed_documents
folder. The folder structure should look like this:
delete_pdf├── input_documents| └── document.pdf├── processed_documents| └── result.pdf├── src| └── main| └── java| └── processor.java
Final Words
In this post, you learned how to easily and seamlessly delete pages from a PDF document for your Java application using our Delete PDF Page API.
You can integrate these functions into your existing applications to easily remove pages from PDFs. With the same API token, you can also perform other operations, such as merging documents into a single PDF, adding watermarks, and more. To get started with a free trial, sign up(opens in a new tab) here.