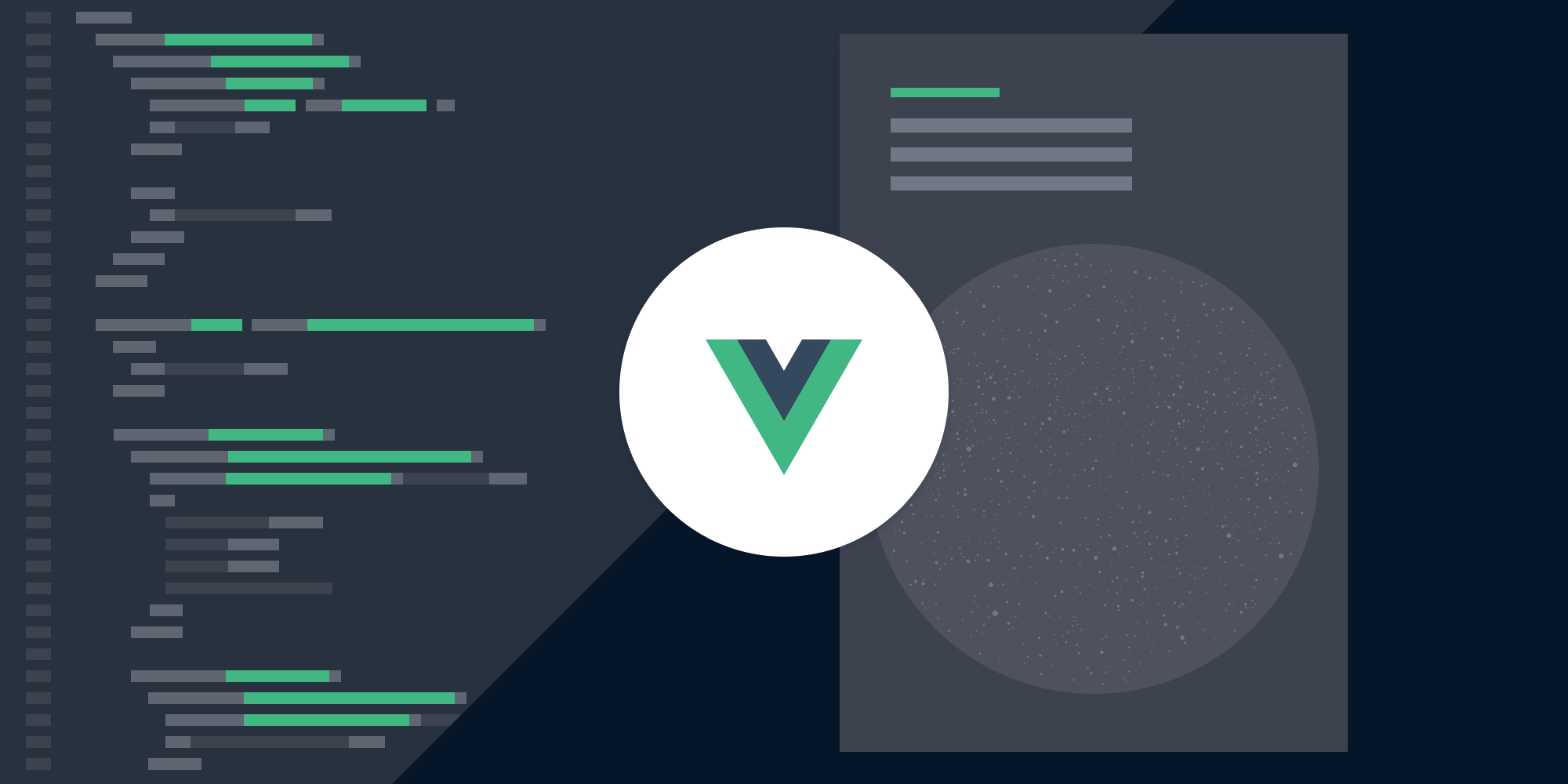
TL;DR
This tutorial compares three libraries for HTML-to-PDF conversion in Vue.js applications: html2pdf.js (simple integration with limited customization), jsPDF (programmatic creation with extensive customization), and pdfmake (complex layouts with detailed formatting). Choose html2pdf.js for straightforward conversion of existing HTML elements, jsPDF for dynamic PDF generation with tables and images, or pdfmake for highly structured documents with custom layouts. Each library offers different tradeoffs between ease of implementation and formatting control.
Generating a PDF from HTML is a common requirement in modern web applications. With Vue.js, you can efficiently create and customize PDF documents using libraries like html2pdf.js. In this post, we’ll show you how to leverage html2pdf.js to generate PDFs from Vue components, covering essential steps and options for a smooth implementation.
If you prefer a video tutorial, you can watch our step-by-step guide.
Prerequisites
This post assumes you’re familiar with Vue.js. If you aren’t, first read through the Vue.js guides.
Start by creating a boilerplate Vue.js project using npm create vue@latest
. This command will install and launch create-vue
, the official tool for setting up Vue projects. You’ll be prompted to select various optional features, such as TypeScript and testing support. Refer to the list below for guidance.
-
Project name: …
<your-project-name>
-
Add TypeScript? … No / Yes — Select
No
. -
Add JSX Support? … No / Yes — Select
Yes
. -
Add Vue Router for Single Page Application development? … No / Yes — Select
No
. -
Add Pinia for state management? … No / Yes — Select
No
. -
Add Vitest for Unit testing? … No / Yes — Select
No
. -
Add an End-to-End Testing Solution? … No / Cypress / Nightwatch / Playwright — Select
No
. -
Add ESLint for code quality? … No / Yes — Select
No
. -
Add Prettier for code formatting? … No / Yes — Select
Yes
. -
Add Vue DevTools 7 extension for debugging? (experimental) … No / Yes — Select
No
.
Once your project is set up, install html2pdf.js with:
npm install html2pdf.js
The creator of html2pdf.js
suggests using v0.9.3. We used the latest version and didn’t have a problem, but if you encounter problems in your project, downgrading might be the answer.
Generating a PDF from HTML with html2pdf.js
This post shows how to convert an img
element to PDF. Add the correct URL or file path to the string placeholder used in the src
tag of the img
element.
-
Setting up your Vue component
Update your App.vue
file to include an element for conversion and a button to trigger the PDF generation:
<template> <div id="app" ref="document"> <div id="element-to-convert"> <center> <img width="400" src="./path-or-url" /> </center> </div> </div> <button @click="exportToPDF">Export to PDF</button> </template> <style> #app { margin-top: 60px; } </style>
It will look like what’s shown below.
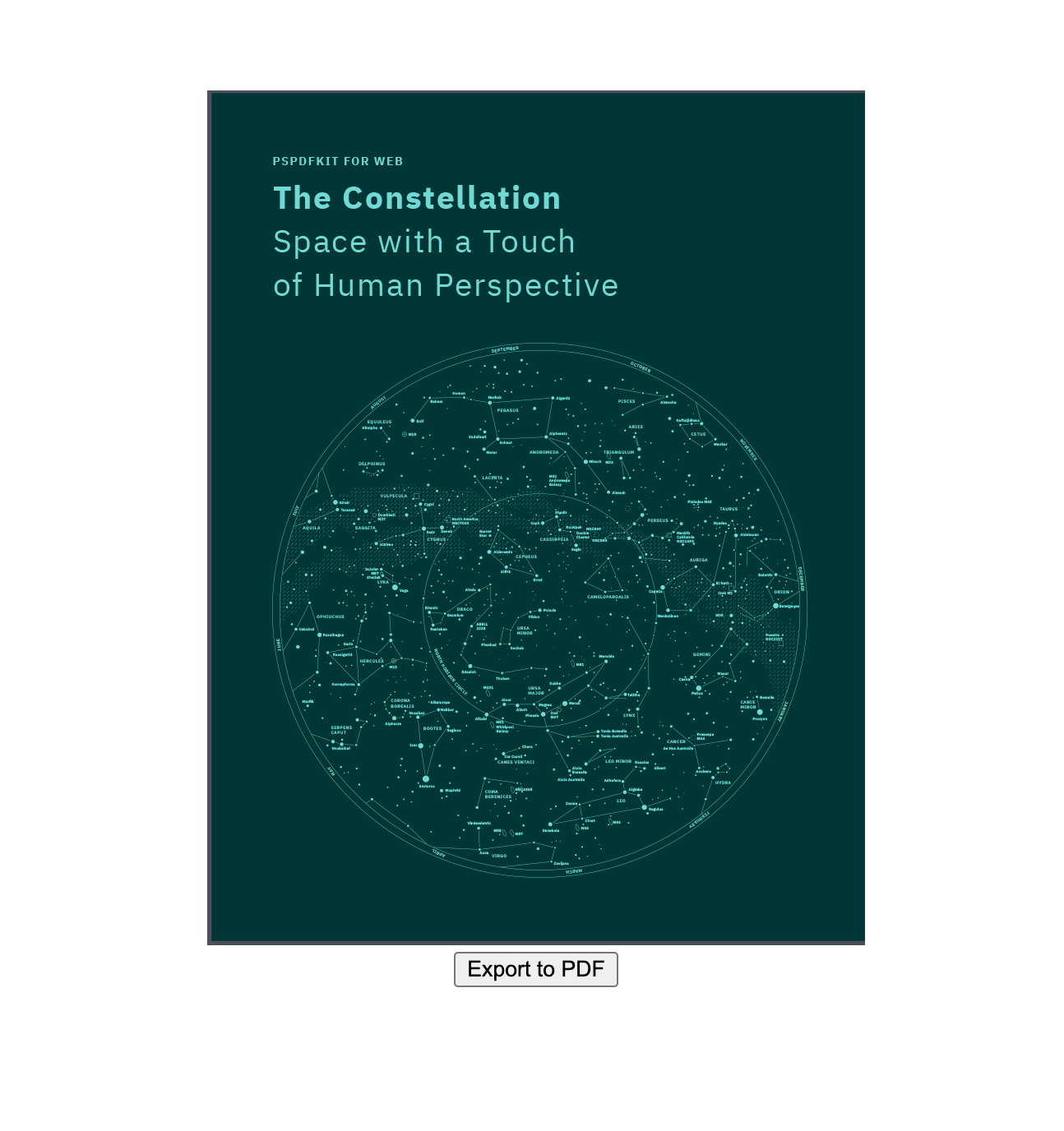
-
Adding PDF generation logic
In the App.vue
script section, import html2pdf
and define the exportToPDF
method:
<script> import html2pdf from "html2pdf.js"; export default { name: "App", methods: { exportToPDF() { html2pdf(document.getElementById("element-to-convert"), { margin: 1, filename: "generated-pdf.pdf", }); }, }, }; </script>
The exportToPDF
function takes a second parameter, an object, which allows you to configure some options that control how the PDF will look. The available options are margin
, filename
, pagebreak
, image
, enableLinks
, html2canvas
, and jsPDF
. You can read more about them here.
For this example, you’ll use a couple of them:
exportToPDF() { html2pdf(document.getElementById("element-to-convert"), { margin: 1, filename: "generated-pdf.pdf", }); },
The full solution
Here’s how the App.vuejs
file will look after your changes:
<template> <div id="app" ref="document"> <div id="element-to-convert"> <center> <img width="400" src="./path-or-url" /> </center> </div> <button @click="exportToPDF">Export to PDF</button> </div> </template> <script> import html2pdf from "html2pdf.js"; export default { name: "App", methods: { exportToPDF() { html2pdf(document.getElementById("element-to-convert"), { margin: 1, filename: "generated-pdf.pdf", }); }, }, }; </script> <style> #app { margin-top: 60px; text-align: center; } </style>
Run your Vue.js project with npm run serve
. The result is a website with an image and an Export to PDF button that converts the image to PDF when pressed.
Alternative libraries for PDF generation in Vue.js
While html2pdf.js is a popular choice for converting HTML to PDF in Vue.js projects, there are several other libraries that may better suit your needs, depending on your specific requirements. Below is a comparison of some Vue.js-compatible libraries that you can consider.
Comparison of Vue.js-compatible libraries for HTML-to-PDF conversion
Library | Key features | Ease of use | Customization options | Documentation |
---|---|---|---|---|
html2pdf.js | Simple integration, converts HTML directly to PDF | Easy | Limited | Good |
jsPDF | Programmatic PDF creation, supports tables and images | Moderate | High | Extensive |
pdfmake | Custom PDF layout, supports complex content structures | Moderate | High | Good |
Library overviews
-
html2pdf.js: This library is ideal for straightforward use cases where you need to convert existing HTML to PDF with minimal setup. It’s easy to integrate into Vue.js projects and offers a simple API for basic PDF generation needs.
-
jsPDF: If you need more control over the structure and content of your PDFs, jsPDF might be the better option. It allows for programmatic creation of PDFs, supporting tables, images, and other advanced features. This library is suitable for projects where you need to generate PDFs dynamically based on user input or other data.
-
pdfmake: pdfmake is a powerful library that supports complex content structures and custom PDF layouts. It’s well-suited for applications where you need to create highly customized PDFs with detailed formatting. While it requires a bit more effort to set up, it offers extensive customization options.
Integration of jsPDF in Vue.js
-
Install jsPDF
npm install jspdf
-
Import and use it in your Vue component
Create an instance of jsPDF
and use its methods to add content and customize your PDF document. For example, you can add text to the PDF and then save it:
import jsPDF from 'jspdf'; const doc = new jsPDF(); // Add text to the PDF. doc.text('Hello world!', 10, 10); // Save the PDF. doc.save('document.pdf');
Here’s a complete example of using jsPDF in a Vue component to generate and download a PDF:
<template> <div> <button @click="generatePDF">Generate PDF</button> </div> </template> <script> import jsPDF from 'jspdf'; export default { name: 'PDFGenerator', methods: { generatePDF() { // Create a new jsPDF instance. const doc = new jsPDF(); // Add text to the PDF. doc.text("Hello world!", 10, 10); // Save the PDF with a filename. doc.save("document.pdf"); }, }, }; </script>
Integration of pdfmake in Vue.js
To use pdfmake
for generating PDFs in your project, you’ll need to follow the steps below to integrate and use the library.
-
Install pdfmake in your project
npm install pdfmake
-
Import pdfmake and fonts
import pdfMake from 'pdfmake/build/pdfmake'; import pdfFonts from 'pdfmake/build/vfs_fonts'; // Set the fonts for pdfMake. pdfMake.vfs = pdfFonts.pdfMake.vfs;
-
Define the document content
Create a document definition object that specifies the content and layout of your PDF:
const docDefinition = { content: 'This is a sample PDF' };
-
Generate and download the PDF
Use the createPdf
method from pdfMake
to generate and download the PDF:
pdfMake.createPdf(docDefinition).download('sample.pdf');
Here’s a complete example of how to use pdfmake in a Vue component:
<template> <div> <button @click="generatePDF">Generate PDF</button> </div> </template> <script> import pdfMake from 'pdfmake/build/pdfmake'; import pdfFonts from 'pdfmake/build/vfs_fonts'; // Set the fonts for pdfMake. pdfMake.vfs = pdfFonts.pdfMake.vfs; export default { name: 'PDFGenerator', methods: { generatePDF() { const docDefinition = { content: 'This is a sample PDF', }; pdfMake.createPdf(docDefinition).download('sample.pdf'); }, }, }; </script>
Conclusion
html2pdf.js enables us to generate PDFs from HTML quickly and seamlessly. It’s a great choice for building simple applications that generate PDF documents. In this post, you saw how it’s possible to achieve this goal with a few lines of code.
If you have — or predict having — more complex workflows and needs, Nutrient can help. For example, if you’re interested in automating the PDF generation process, refer to our [PDF Generator API][], which enables you to generate PDF files from HTML templates. You can style the CSS, add unique images and fonts, and persist your headers and footers across multiple pages.
If you’re also interested in displaying PDFs in a powerful and customizable viewer that provides a number of advanced functionalities like annotating PDFs or digitally signing them, we recommend checking out Document Engine.
For more information on converting and generating PDFs, check out our related blog posts:
FAQ
How can I generate a PDF in a Vue.js application?
You can generate a PDF in a Vue.js application using libraries like html2pdf.js, jsPDF, or pdfmake. These libraries help convert HTML content to PDF format within a Vue component.What are the steps to install html2pdf in a Vue.js project?
Install html2pdf using npm with the commandnpm install html2pdf.js
. Then import and use it in your Vue components to capture and convert HTML content to PDF.
How does pdfmake differ from html2pdf in Vue.js applications?
pdfmake offers more control over PDF layout and content, allowing for programmatically generated PDFs with complex structures, whereas html2pdf is easier to use for converting existing HTML content to PDF.Can I generate PDFs from dynamic content in Vue.js?
Yes, you can generate PDFs from dynamic content by binding data to your Vue components and using libraries like html2pdf.js to capture the rendered HTML for conversion to PDF.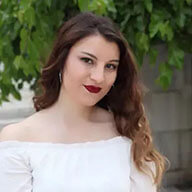
Hulya is a frontend web developer and technical writer at Nutrient who enjoys creating responsive, scalable, and maintainable web experiences. She’s passionate about open source, web accessibility, cybersecurity privacy, and blockchain.