How to print PDFs using PDF.js
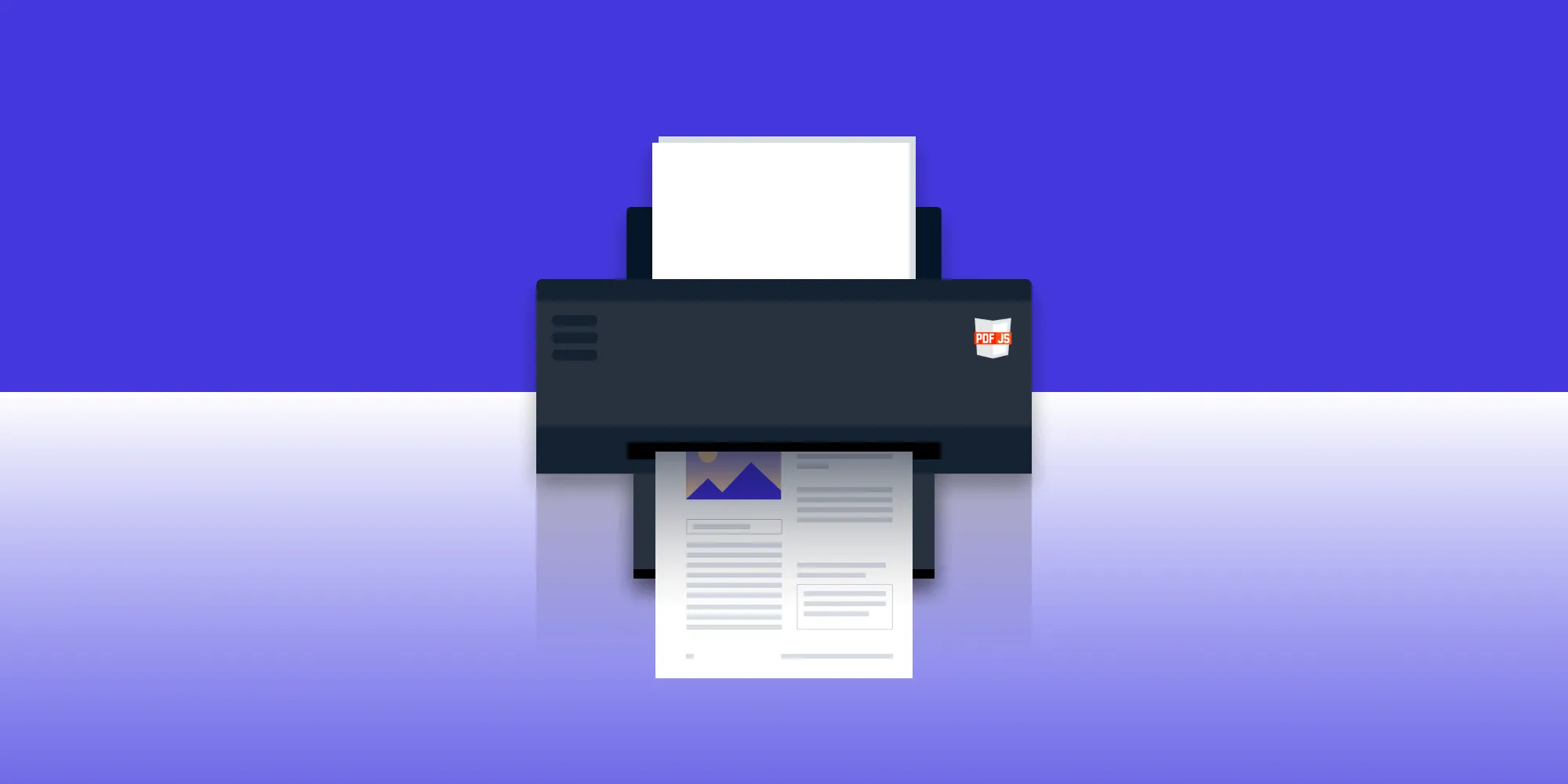
This tutorial presents three methods for printing PDFs with PDF.js: using window.print()
(fast and simple but may have layout issues), using the Print.js library (better control with minimal setup), and rendering in a hidden iframe (best quality using the browser’s native PDF capabilities). Implementation involves adding a print button to your toolbar and applying the appropriate JavaScript. Each approach has tradeoffs: window.print()
is fast but may not preserve document fidelity, Print.js offers more options but requires an additional library, and the iframe method provides the most reliable output but requires more complex code. Choose based on your specific printing quality requirements.
In a previous tutorial, you saw how to render and embed a PDF viewer in the browser with PDF.js. In this post, you’ll look at how to print PDFs using PDF.js.
If you read the aforementioned blog post, you’ll see that embedding a PDF.js viewer example provides a fully functional toolbar, and you can use the print button to print the PDF.
In this post, you’ll add a print button to your custom toolbar using the pdfjs-display-example(opens in a new tab).
Adding a print button to the PDF.js toolbar
The source code for adding a print button is the same code used in the How to build a JavaScript PDF viewer with PDF.js blog.
Step 1 — Cloning the project
Start by cloning the project from GitHub and changing your directory to the pdfjs-display-example
folder:
git clone https://github.com/PSPDFKit-labs/pdfjs-web-example-javascript.git
cd pdfjs-display-example
Step 2 — Adding a print button to the toolbar
Add a print button to the toolbar using the print
icon from Font Awesome(opens in a new tab):
<li class="navigation__item"> <button class="print-button"> <i class="fa-solid fa-print"></i> </button></li>
Add this list item inside the ul
element with the navigation
class.
Step 3 — Attaching a click event listener
Open the index.js
file and attach a click event listener to the button:
const printButton = document.querySelector('.print-button');
// Print PDF.printButton.addEventListener('click', () => { window.print();});
Here, access the button’s class with querySelector()
(opens in a new tab), and add an event listener to it. When the button is clicked, the window.print()
method will be called.
Step 4 — Hiding the toolbar during printing
Now, when you click the print button, the PDF will be printed. To disable printing the toolbar, use the @media print
CSS rule:
/* Hide Print button */@media print { .navigation { display: none; }}
Advantages and limitations of window.print()
Advantages:
- The PDF is generated directly from HTML, CSS, and JavaScript in the browser.
- No dependency on external tools.
- Faster execution.
- Supports the latest CSS properties.
Limitations:
- Server-side file storage is not supported.
- Printing from a
<canvas>
element might not preserve the PDF’s layout, text, or image quality as well as a native PDF viewer.
Using Print.js for better control
An alternative to window.print()
is using Print.js(opens in a new tab), which is a library specifically created for printing PDFs.
Step 1 — Adding the CDN links
To use this library, download the latest version from the GitHub releases page(opens in a new tab) and add it to your project.
Or, download using npm(opens in a new tab), or use a CDN(opens in a new tab). This tutorial uses the CDN option.
Add the CDN links to the index.html
file. Place the print.css
link before the closing </head>
tag:
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/print-js/1.6.0/print.css" integrity="sha512-tKGnmy6w6vpt8VyMNuWbQtk6D6vwU8VCxUi0kEMXmtgwW+6F70iONzukEUC3gvb+KTJTLzDKAGGWc1R7rmIgxQ==" crossorigin="anonymous" referrerpolicy="no-referrer"/>
Include the print.js
script before the closing </body>
tag:
<script src="https://cdnjs.cloudflare.com/ajax/libs/print-js/1.6.0/print.js" integrity="sha512-/fgTphwXa3lqAhN+I8gG8AvuaTErm1YxpUjbdCvwfTMyv8UZnFyId7ft5736xQ6CyQN4Nzr21lBuWWA9RTCXCw==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
Step 2 — Updating the print button
You can reuse the same print button from the previous section. Update the click handler function to use the printJS()
method:
printButton.addEventListener('click', () => { printJS('canvas', 'html');});
Print.js supports printing HTML content. To print the PDF, pass the DOM selector of the element — in this case, it’s canvas
— to the printJS()
method. The second argument is the type of the content. In this case, it’s html
.
You can print any element as long as it has a unique selector. Now, when you click the print button, the PDF will be printed in a way similar to the window.print()
method.
Rendering the PDF in a hidden iframe
Another effective method for printing PDFs is by rendering a PDF in an invisible iframe and then using the iframe’s print()
method. This approach ensures that the PDF is printed exactly as it appears in the viewer, utilizing the browser’s native PDF rendering capabilities:
const printButton = document.querySelector('.print-button');
printButton.addEventListener('click', () => { const iframe = document.createElement('iframe'); iframe.style.position = 'fixed'; iframe.style.right = '100%'; iframe.style.bottom = '100%'; iframe.src = 'document.pdf'; // URL of the PDF file iframe.onload = () => { iframe.contentWindow.print(); }; document.body.appendChild(iframe);});
Why use this method?
This method ensures that the PDF is rendered by the browser’s native PDF viewer, which typically handles printing better than directly rendering the PDF on a <canvas>
element and printing from there. The browser’s PDF viewer often provides more consistent results and better support for advanced PDF features like vector graphics and embedded fonts.
Conclusion
In this tutorial, we explored several methods for printing PDFs using PDF.js, including the use of window.print
, Print.js, and rendering the PDF in a hidden iframe. Each method has its advantages and limitations, so you can choose the one that best suits your needs.
If you’re looking to add more robust PDF capabilities, consider using Nutrient, a commercial JavaScript PDF library that integrates easily into your web application. It offers more than 30 features, including viewing, annotating, editing, and signing documents directly in your browser. With a polished and flexible UI, Nutrient can be extended or simplified based on your unique use case.
You can explore Nutrient’s web framework deployment options like React.js, Angular, and Vue.js. Start your free trial or launch our demo to see our viewer in action.
FAQ
How Do I Add a Print Button to a `PDF.js` Viewer?
Clone the project, add a print button in HTML, attach a window.print()
event listener in index.js
, and use CSS to hide the toolbar during printing.
What Is the Advantage of Using `window.print()` for Printing PDFs?
window.print()
generates the PDF directly from the browser, is tool-independent, and supports modern CSS properties.
Can I Use `Print.js` to Print PDFs from `PDF.js`?
Yes, you can use Print.js
by including its library and updating the print button’s click handler to use printJS()
with the PDF element’s selector.
What Are the Limitations of `window.print()` for Printing PDFs?
window.print()
doesn’t support server-side file storage.