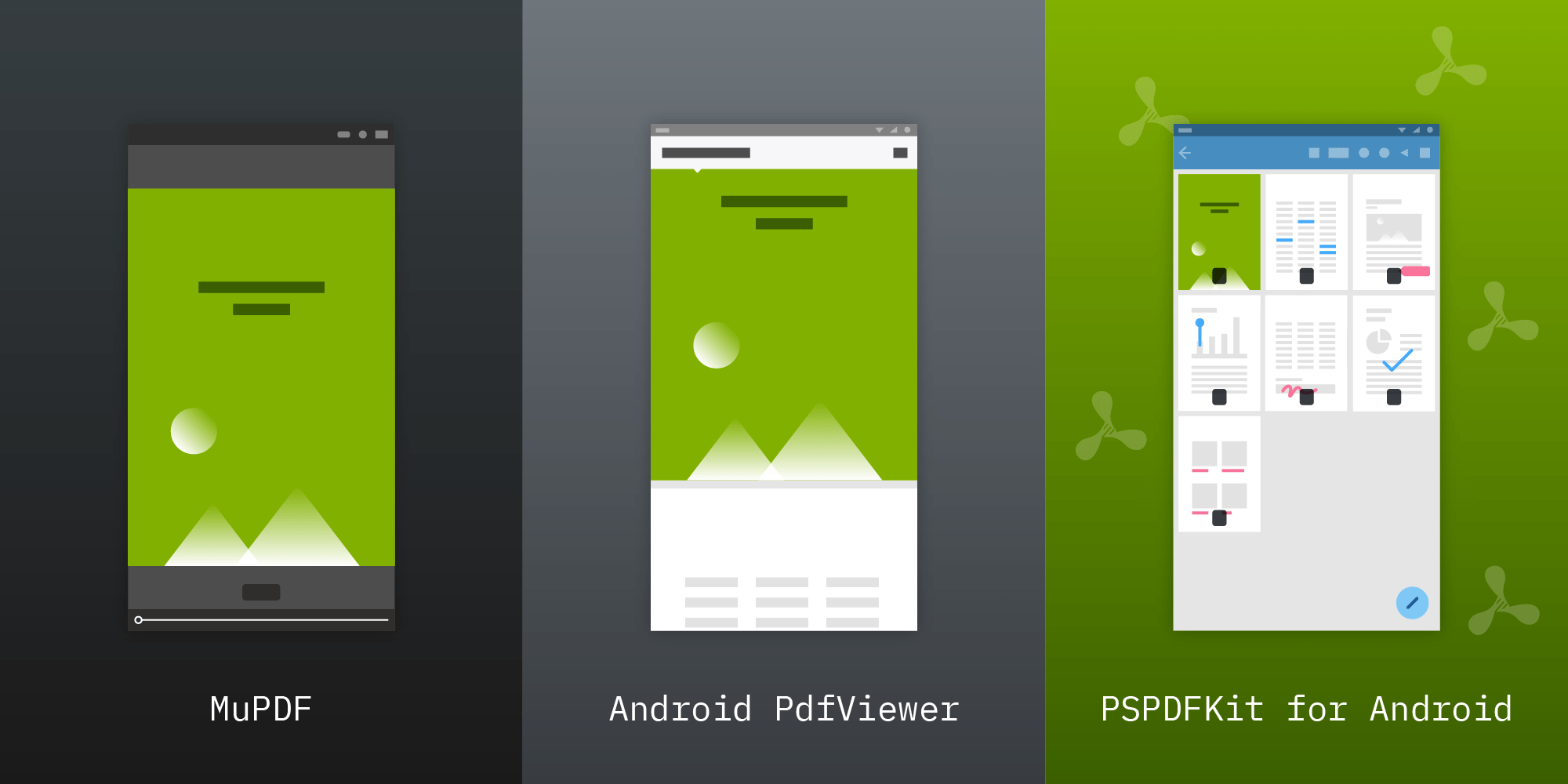
PDF documents have become the standard for securely archiving, transferring, and presenting documents online. For Android developers, integrating PDF viewing capabilities directly within their applications is a common requirement. In this article, we’ll explore several options for displaying PDFs in Android apps, starting with the basic capabilities of the Android SDK and moving on to third-party libraries like Android PdfViewer and Nutrient Android SDK. These solutions range from simple, open source options to more advanced, feature-rich libraries.
Reading PDF files on Android
Reading PDF files on Android devices is a straightforward process that can be accomplished using various apps. Android devices come with built-in support for PDF files, allowing users to view and interact with them using a range of apps. To read PDF files on Android, users can use apps like Google PDF Viewer, Files by Google, or Adobe Acrobat Reader. These apps allow users to view PDF files, zoom in and out, and navigate through the document using intuitive gestures.
Displaying PDFs using the Android SDK
The Android SDK has had basic support for PDF files since API level 21 (Android 5.0). This API resides in a package, android.graphics.pdf
, and it supports basic low-level operations, such as creating PDF files and rendering pages to bitmaps. The Android SDK doesn’t provide a UI for interacting with PDF documents, so you’ll need to write your own UI to handle user interaction if you wish to use it as a basis for a PDF viewer in your app.
The main API entry point is PdfRenderer
, which provides an easy way to render single pages into bitmaps:
// Create the page renderer for the PDF document. val fileDescriptor = ParcelFileDescriptor.open(documentFile, ParcelFileDescriptor.MODE_READ_ONLY) val pdfRenderer = PdfRenderer(fileDescriptor) // Open the page. val page = pdfRenderer.openPage(pageNumber) // Render the page to the bitmap. val bitmap = Bitmap.createBitmap(page.width, page.height, Bitmap.Config.ARGB_8888) page.render(bitmap, null, null, PdfRenderer.Page.RENDER_MODE_FOR_DISPLAY) // Use the rendered bitmap. ... // Close the page when you're done with it. page.close() ... // Close the `PdfRenderer` when you're done with it. pdfRenderer.close()
You can now set these rendered bitmaps into an ImageView
or build your own UI that handles multi-page documents, scrolling, and zooming.
Rendering PDFs with the Android PdfViewer library
Android PdfViewer is a library for displaying PDF documents. It has basic support for page layouts, zooming, and touch gestures. It’s available under the Apache License, Version 2.0 and, as such, is free to use, even in commercial apps.
Integration is simple. Start with adding a library dependency to your build.gradle
file:
compile 'com.github.barteksc:android-pdf-viewer:3.1.0-beta.1'
Then add the main PDFView
to the layout where you wish to view the PDFs:
... <com.github.barteksc.pdfviewer.PDFView android:id="@+id/pdfView" android:layout_width="match_parent" android:layout_height="match_parent"/> ...
Now you can load the document in your activity:
...
val pdfView = findViewById<PdfView>(R.id.pdfView)
pdfView.fromUri(documentUri).load()
...
Nutrient Android SDK
The solutions above are free or open source libraries with viewer capabilities. However, the PDF spec is fairly complex, and it defines many more features on top of the basic document viewing capabilities. We at Nutrient offer a comprehensive PDF solution for Android and other platforms, along with first-class support included with every plan. Nutrient comes with a fully featured document viewer with a modern customizable user interface and a range of additional features such as:
-
Annotation editing
-
Interactive forms with JavaScript support
-
Digital signatures
-
Indexed search
-
Document editing (both programmatically and through the UI)
-
Redaction
-
And much more
If you’re interested in our solution, visit the Android product page to learn more and download a free trial.
Getting started with Nutrient Android SDK
To integrate Nutrient into your Android app, follow the steps outlined below.
Step 1 — Creating a new project
-
Open Android Studio and select File > New > New Project….
-
Choose the appropriate template for your project. In this example, select Empty Activity.
-
Provide the app name (e.g. Nutrient Demo) and set the save location, the language, and the minimum SDK to 21 or higher.
Step 2 — Adding Nutrient to your project
-
In your
settings.gradle.kts
file, add the Nutrient Maven repository:
dependencyResolutionManagement { repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS) repositories { google() mavenCentral() maven { url = uri("https://my.nutrient.io/maven") } } }
-
In your
app/build.gradle.kts
file, add the Nutrient dependency:
dependencies {
implementation("io.nutrient:nutrient:10.1.1")
}
Step 3 — Configuring your build
Nutrient supports Android devices running API level 21 and above.
Make sure your app/build.gradle.kts
file has the following configuration:
android { compileSdk = 35 defaultConfig { applicationId = "com.example.app" minSdk = 21 targetSdk = 35 } }
Step 4 — Displaying a PDF document
To verify that Nutrient has been successfully integrated into your app, open a PDF file with the ready-to-use PdfActivity
.
-
Optional: If you have a trial or license key, add it to your
AndroidManifest.xml
:
<application> <meta-data android:name="nutrient_license_key" android:value="YOUR_LICENSE_KEY_GOES_HERE" /> ... </application>
-
Copy a PDF document to the assets directory of your Android project, e.g.
src/main/assets/my-document.pdf
. -
Add
PdfActivity
to your app’s AndroidManifest.xml:
<application> ... <activity android:name="com.pspdfkit.ui.PdfActivity" android:windowSoftInputMode="adjustNothing" /> </application>
-
Start
PdfActivity
with the document from your assets directory:
class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val uri = Uri.parse("file:///android_asset/my-document.pdf") val config = PdfActivityConfiguration.Builder(this).build() PdfActivity.showDocument(this, uri, config) } }
class MainActivity extends AppCompatActivity { @Override protected void onCreate(@Nullable final Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); final Uri uri = Uri.parse("file:///android_asset/my-document.pdf"); final PdfActivityConfiguration config = new PdfActivityConfiguration.Builder(context).build(); PdfActivity.showDocument(this, uri, config); } }
PdfActivity
will now present the document from your assets directory.
![]()
The
android_assets
folder is read-only and serves as a simple example. For practical use, consider copying the file to a local folder for full read and write privileges. Learn more from our guide on opening PDFs from URLs and Google’s Data and file storage overview.
Choosing a PDF reader
Choosing the right PDF reader for Android can be a daunting task, given the numerous options available. When selecting a PDF reader, users should consider their specific needs and preferences. For example, if you need to annotate or edit PDF files, you may want to choose a PDF reader that offers these features. On the other hand, if you simply need to view PDF files, a basic PDF reader may suffice.
Some key factors to consider when choosing a PDF reader include:
-
Compatibility — Ensure that the PDF reader is compatible with your Android device and operating system.
-
Features — Consider the features you need, such as annotation, highlighting, and bookmarking.
-
User interface — Choose a PDF reader with an intuitive and user-friendly interface.
-
Performance — Opt for a PDF reader that’s fast and responsive.
PDF reader features and alternatives
PDF readers for Android offer a range of features that make it easy to read and interact with PDF files. Some common features include:
-
Annotation — Allow users to add notes, highlights, and bookmarks to PDF files.
-
Highlighting — Enable users to highlight important text or passages in PDF files.
-
Bookmarking — Allow users to bookmark specific pages or sections in PDF files.
-
PDF converter — Enable users to convert PDF files to other file formats, such as Word or Excel.
-
PDF editor — Allow users to edit PDF files, including adding or removing text and images.
In addition to these features, some PDF readers offer alternative features that can enhance the reading experience. For example, some PDF readers offer night mode, which adjusts the screen brightness and color temperature to reduce eye strain. Others offer text-to-speech functionality, which allows users to listen to PDF files being read aloud.
Conclusion
In this post, we outlined some of the available free PDF viewer libraries for Android that can help you render PDFs. However, if you want to add more advanced features to your PDF viewer — such as PDF annotation support, interactive PDF forms, and digital signatures — consider looking into commercial alternatives.
At Nutrient, we offer a commercial, feature-rich, and completely customizable Android PDF library that’s easy to integrate and comes with well-documented APIs to handle complex use cases. Try our PDF library using our free trial, and check out our demos to see what’s possible.
FAQ
What’s the simplest way to display a PDF in an Android app?
You can use thePdfRenderer
class in the Android SDK for basic PDF rendering.
Can I use a free library to display PDFs in my Android app?
Yes, the Android PdfViewer library is a popular open source option for displaying PDFs.What additional features does Nutrient offer over free alternatives?
Nutrient provides advanced features like annotation editing, digital signatures, form filling, and document editing.Is Nutrient compatible with older Android versions?
Nutrient supports Android 5.0 (API level 21) and above.How do I start using Nutrient in my Android project?
Add the Nutrient Maven repository and dependency to yourbuild.gradle
file. Then use PdfActivity
to display PDFs.