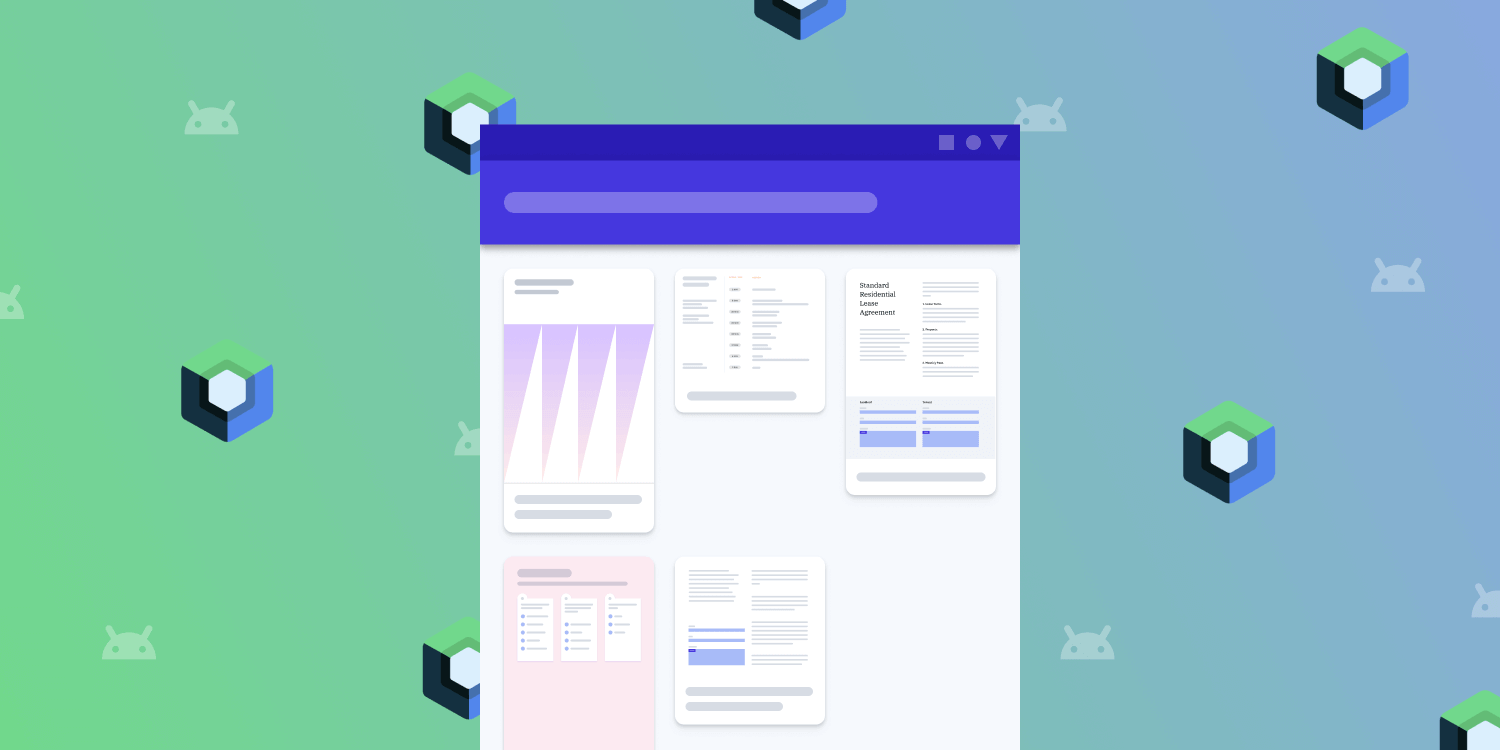
Jetpack Compose is Google’s declarative UI framework designed for modern Android development. It empowers engineers to build elegant and responsive UIs with less code and fewer bugs. Since its stable release, Jetpack Compose has become a popular choice for developing new Android applications and is increasingly used in production environments.
In this blog post, you’ll learn how to integrate Nutrient Android SDK with your Jetpack Compose application. We’ll look at how to create a @Composable
function that previews a document’s thumbnail and opens the document when tapped. In case you want to dive into the code, you can check it out in this repo. Even though it’s by no means what can be considered production-ready code, there are some good practices baked into it that can potentially be useful for real-world scenarios.
Introduction to Android UI development with Jetpack Compose
Android user interface (UI) development with Jetpack Compose introduces a modern and efficient way to build native user interfaces for Android applications. As a powerful UI toolkit, Jetpack Compose enables developers to create fast, beautiful, and reliable UIs using Kotlin. With its declarative approach, developers can design reusable and manageable UI components effortlessly.
Jetpack Compose uses a declarative programming model, allowing you to describe how your UI should appear, while Compose handles the implementation. This reduces boilerplate code, enhances predictability, and simplifies debugging.
A key feature of Jetpack Compose is that of its composable functions, which empower developers to create modular and reusable UI components. These functions streamline the development process and offer unparalleled flexibility for crafting intuitive and modern Android interfaces.
This post will explore the core concepts of Jetpack Compose and show how to build visually appealing and efficient Android UIs. Whether you’re a seasoned Android developer or starting your journey, Jetpack Compose offers a transformative approach to UI development.
Getting started
Before you can start building your app, you need to have Jetpack Compose and Nutrient Android SDK configured. If you already have these two in place, you can skip to the next section.
-
Open Android Studio and select File > New > New Project… to create a new project for your application.
-
Choose Empty Activity as the template.
-
Set your app name (e.g. Nutrient Demo), and specify the Save location, Language, and Minimum SDK 21.
-
Click Finish to save the project.
Adding Nutrient to your project
-
Update
settings.gradle
:
// settings.gradle dependencyResolutionManagement { repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS) repositories { google() mavenCentral() maven { url = uri("https://my.nutrient.io/maven") } } }
-
Update
app/build.gradle
:
// app/build.gradle android { compileSdk = 35 defaultConfig { applicationId = "com.example.app" minSdk = 21 targetSdk = 35 } } dependencies { implementation "io.nutrient:nutrient:10.1.1" }
![]()
If you have a more advanced integration scenario for Nutrient, you can check out our integration guide.
Displaying a PDF
The other thing you need before displaying a document is… well, the document itself! For the sample project, I added a few documents to Android’s src/main/assets/
folder, but your PDFs can come from pretty much anywhere. Nutrient provides built-in APIs for displaying PDFs inside Composable apps. You’ll use them in this example, but you aren’t limited to showing your PDFs this way. To learn more about how to open documents, refer to this guide on opening PDFs from custom data providers.
With the setting up of things out of the way, it’s now time write some declarative UI!
Preparing the stage
When working with Jetpack Compose, you can take full advantage of modern Android development practices to simplify your code. For this example app, you’ll use AppCompatActivity
to serve as the base activity and leverage the DocumentView
composable to display a PDF document from the assets folder. This composable makes it easy to render PDFs in a declarative way.
Here’s what your MainActivity
looks like:
// MainActivity.kt class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { Surface { val uri = Uri.parse("file:///android_asset/my-document.pdf") DocumentView( documentUri = uri, modifier = Modifier.fillMaxSize() ) } } } }
Showing PDF documents in Compose
Since version 8.0, Nutrient Android SDK has offered composable APIs that make integration with Jetpack Compose seamless. To display a PDF document, use the DocumentView
composable, which accepts a URI pointing to the document you want to display. This allows you to create a modern, clean, and responsive UI for PDF viewing.
Here’s a quick breakdown of how DocumentView
works:
-
URI-based loading — The
DocumentView
composable takes a URI as an input. In this case, the URI points to a PDF file stored in the app’sassets
folder (file:///android_asset/my-document.pdf
). -
Composable integration — Because
DocumentView
is a composable, you can use it like any other Jetpack Compose UI element. You can customize its size, layout, and behavior by combining it with other composables and using standard Compose modifiers. -
Simplifying PDF handling — By using
DocumentView
, you avoid the need for complex setup and boilerplate code that would otherwise be required to integrate a PDF viewer into your app. This composable abstracts away the underlying PDF rendering logic, making it easier to focus on building your app’s user interface.
With the DocumentView
composable, you can quickly add PDF viewing capabilities to your Compose app, allowing users to view documents with a modern, responsive UI. For more advanced usage, such as adding annotations or handling user interactions, refer to Nutrient’s comprehensive documentation.
Handling PDF loading and errors
When working with PDF files in Android UI development using Jetpack Compose, it’s essential to handle loading and errors effectively. Follow these best practices to ensure a seamless user experience:
-
Use a reliable library
Utilize a library like AndroidPdfViewer to simplify loading and displaying PDFs. These libraries are optimized for performance and offer features that enhance the user experience.
-
Implement error handling
Anticipate potential errors during PDF loading. Display an error message or placeholder image if a PDF fails to load, ensuring your app remains functional and user-friendly.
-
Provide loading indicators
Use loading indicators to inform users when a PDF is being loaded. This enhances the user experience by offering visual feedback.
-
Optimize loading times
Leverage caching and lazy loading techniques to improve performance. Caching frequently accessed PDFs reduces load times, while lazy loading ensures only the necessary parts of a file are loaded initially.
By following these best practices, you can deliver a reliable and responsive experience when working with PDFs in your Android app.
Top best practices for Jetpack Compose development
Jetpack Compose revolutionizes Android UI development with its declarative approach. To make the most of its capabilities, consider these best practices:
-
Adopt a declarative programming model
Define your UI components declaratively for improved code readability and maintainability.
-
Leverage composable functions
Build reusable, modular UI components with composable functions. This simplifies creating complex interfaces from manageable building blocks.
-
Effectively manage state
Use Jetpack Compose’s state management features to handle UI state and user interactions. Proper state management ensures responsiveness and consistency.
-
Utilize UI composition
Combine smaller composable functions to build intricate user interfaces. This promotes code reuse and simplifies the development process.
-
Optimize performance
Take advantage of Jetpack Compose’s built-in performance optimizations, such as managing recomposition effectively to prevent unnecessary updates.
-
Test and debug early
Use Compose’s robust tools for testing and debugging UI components. Identifying and resolving issues early leads to a stable, reliable app.
By adhering to these best practices, you can create fast, beautiful, and reliable Android applications. Jetpack Compose provides a powerful toolkit for modern UI development, and following these guidelines will help you unlock its full potential.
Conclusion
Combining the power of Jetpack Compose with Nutrient’s DocumentView
makes it incredibly easy to integrate PDF viewing into modern Android apps. With this setup, you can leverage the full capabilities of both frameworks to create a polished and responsive user experience.
FAQ
How do I add Nutrient to my Android project?
You can add Nutrient by including it as a dependency in yourbuild.gradle
file and configuring your repositories.
What is Jetpack Compose?
Jetpack Compose is Google’s declarative UI framework for Android that simplifies and accelerates UI development.Can I display a PDF using Jetpack Compose?
Yes, you can display PDFs in Jetpack Compose using Nutrient’sDocumentView
composable.
How do I load a PDF file from assets in Jetpack Compose?
You can use a URI with the pathfile:///android_asset/your-pdf.pdf
to load the PDF from the assets folder.