PDF.js vs. Nutrient Web SDK: A comprehensive PDF viewer comparison
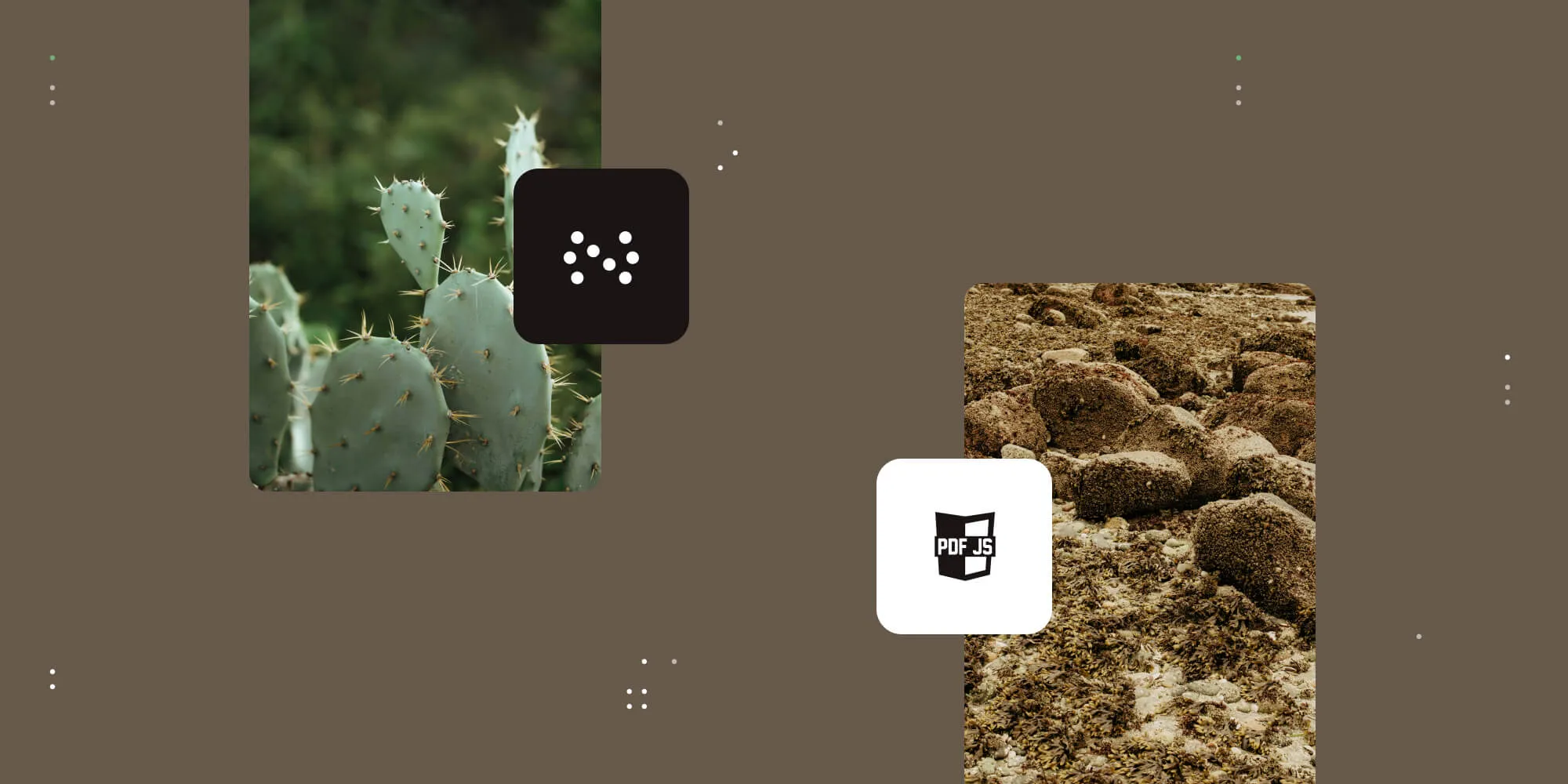
PDF.js is a free, client-side JavaScript library optimized for lightweight PDF rendering using HTML5 <canvas>
, but it lacks native support for annotations, form filling, and advanced document manipulation.
Nutrient Web SDK is a commercial, enterprise-level PDF solution with hybrid rendering (client-server), real-time collaboration, annotations, digital signatures, and superior performance for large-scale applications.
Use PDF.js for basic document rendering where minimal interactivity is required. Choose Nutrient Web SDK for feature-rich, scalable, and secure PDF processing in production environments.
Understanding the need for web-based PDF viewing
Web applications today rely heavily on PDFs for document handling. Whether you’re generating reports, displaying invoices, managing legal documents, or collaborating on shared content, having a reliable PDF viewer is essential. However, the complexity of PDF handling varies depending on the use case:
- Basic viewing — Simply rendering static PDFs in a browser.
- Interactive documents — Filling forms, adding annotations, and signing digital documents.
- Collaboration and workflow — Real-time document editing and secure sharing.
- Security and compliance — Ensuring encrypted access to sensitive documents.
- Automation and processing — Extracting data, redacting content, or generating structured reports.
PDF.js: A lightweight PDF viewer
PDF.js is primarily a PDF viewer, but it also provides tools for parsing and rendering PDF documents. It’s maintained by Mozilla and is widely used to display PDF files in web applications. While PDF.js isn’t a PDF generation tool, it’s invaluable for PDF display and basic manipulation on the client side.
Explore the PDF.js demoKey features of PDF.js
- Client-side PDF rendering — Uses the HTML5
<canvas>
element to display PDFs directly in the browser. - Parsing and rendering capabilities — Supports loading and interpreting PDF documents.
- Works with HTML5 canvas — Uses native browser technology for rendering.
- Maintained by Mozilla — Regular updates and an active open source community.
Use case
PDF.js is ideal for web applications that need to display or manipulate existing PDFs, such as e-readers, embedded document viewers, and online document portals.
Viewing a PDF with PDF.js
<!DOCTYPE html><html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>PDF.js Example</title> </head> <body> <canvas id="pdf-canvas"></canvas>
<script src="/pdf.mjs" type="module"></script> <script type="module"> import { GlobalWorkerOptions } from 'https://cdn.jsdelivr.net/npm/[email protected]/build/pdf.min.mjs';
GlobalWorkerOptions.workerSrc =
const url = 'output.pdf'; // Replace with your PDF URL.
const canvas = document.getElementById('pdf-canvas'); const ctx = canvas.getContext('2d');
pdfjsLib .getDocument(url) .promise.then((pdf) => { pdf.getPage(1).then((page) => { const viewport = page.getViewport({ scale: 1.5 }); canvas.height = viewport.height; canvas.width = viewport.width;
page.render({ canvasContext: ctx, viewport: viewport, }); }); }) .catch((error) => { console.error('Error loading PDF:', error); }); </script> </body></html>
Check out our complete guide to PDF.js for more details on how to use PDF.js in your web application.
Nutrient Web SDK: An enterprise-grade PDF solution
Nutrient Web SDK is an enterprise-grade JavaScript library for viewing, annotating, and editing PDFs directly in the browser. Unlike PDF.js, it offers advanced features such as real-time collaboration, form filling, and digital signing.
Key capabilities of Nutrient Web SDK
Nutrient Web SDK is designed to support a wide range of document processing needs beyond simple viewing. It delivers agile, innovative, and easy-to-use solutions for the entire document ecosystem, including:
- Collaboration — Enable real-time document collaboration with shared annotations and editing tools.
- Document conversion — Convert PDFs to and from multiple formats, including Word, Excel, and images.
- Editing — Modify content, rearrange pages, and apply document-wide changes.
- Forms — Create, fill, and extract data from interactive forms.
- Document generation — Generate PDFs dynamically from structured and unstructured data.
- Redaction — Permanently remove sensitive data while maintaining document integrity.
- Security and compliance — Provides encryption, access controls, and digital rights management.
- Hybrid rendering (client-server) — Provides smooth performance, even with large documents.
Use case
Nutrient Web SDK is best suited for applications that require a fully featured PDF editor or viewer with the ability to add annotations, fill forms, and collaborate on documents in real time.
Example of our JavaScript PDF viewer
To demo our JavaScript PDF viewer, upload a PDF, JPG, PNG, or TIFF file by clicking Open Document under the Standalone option (if you don’t see this option, select Choose Example from the dropdown). Once your document is displayed in the viewer, try drawing freehand, adding a note, or applying a crop or an eSignature.
Viewing a PDF with Nutrient Web SDK
Nutrient Web SDK provides a powerful PDF viewer with support for various document formats, including PDF, PDF/A, Office documents (DOCX, XLSX, PPTX), and images (PNG, JPEG, TIFF). The following example demonstrates how to integrate the SDK for seamless document viewing in the browser:
<!DOCTYPE html><html> <head> <title>My App</title> <meta name="viewport" content="width=device-width, initial-scale=1.0, minimum-scale=1.0, maximum-scale=1.0, user-scalable=no" /> </head> <body> <div id="pspdfkit" style="height: 100vh;"></div>
<script src="assets/pspdfkit.js"></script> <script> PSPDFKit.load({ container: '#pspdfkit', document: 'document.pdf', // Add the path to your file. }) .then(function (instance) { console.log('Nutrient loaded', instance); }) .catch(function (error) { console.error(error.message); }); </script> </body></html>
If you prefer a video tutorial, you can watch our step-by-step guide.
Nutrient Document Authoring
Beyond document viewing, Nutrient Web SDK includes Document Authoring, enabling developers to open, edit, and format DOCX documents directly in the browser. This feature integrates seamlessly with the viewer, providing powerful tools for document creation and editing.
Key features
DOCX editing — Open, modify, and export DOCX files with support for rich text, tables, images, and more.
Multi-format export — Save documents as DOCX or PDF with high fidelity for print and digital use.
DocJSON support — Enables flexible document handling for custom workflows and automation.
Page-based editing — Provides an intuitive in-browser experience similar to traditional word processors.
Offline support — Allows users to edit documents entirely on the client-side without a server connection.
Advantages
High-fidelity exports — Ensures perfect formatting when saving or printing documents.
Full DOCX support — Advanced formatting and layout tools for professional document editing.
Offline editing — Users can edit and save documents without an internet connection.
Easy integration — Built-in TypeScript support for seamless implementation in existing applications.
Compliance and data governance — Offers full control over data storage and security policies.
Getting started with Nutrient Document Authoring
The following example demonstrates how to initialize the Document Authoring SDK and create a simple document editor:
import { createDocAuthSystem } from '@pspdfkit/document-authoring';
(async () => { const docAuthSystem = await createDocAuthSystem(); const editor = await docAuthSystem.createEditor( document.getElementById('editor'), { document: await docAuthSystem.createDocumentFromPlaintext( 'Hello world!', ), }, );})();
This setup allows users to create and edit documents within a web application, providing a seamless document management experience.
Explore the Nutrient Document Authoring demo to see it in action!Adding annotations with Nutrient Web SDK
Nutrient Web SDK makes it easy to programmatically add highlight annotations to PDFs. Using the PSPDFKit.Annotations.HighlightAnnotation
constructor, developers can define specific areas of a PDF to highlight dynamically:
const highlightRects = PSPDFKit.Immutable.List([ new PSPDFKit.Geometry.Rect({ left: 10, top: 10, width: 200, height: 10, }), new PSPDFKit.Geometry.Rect({ left: 10, top: 25, width: 200, height: 10, }),]);const highlightAnnotation = new PSPDFKit.Annotations.HighlightAnnotation( { pageIndex: 0, rects: highlightRects, boundingBox: PSPDFKit.Geometry.Rect.union(highlightRects), },);instance.create(highlightAnnotation);
Learn more about how to add highlight annotations to PDFs in JavaScript and explore 8 common PDF annotation types.
Adding watermarks with Nutrient Web SDK
Nutrient Web SDK enables you to add dynamic watermarks to PDFs displayed in the browser, helping to deter unauthorized screenshots by overlaying user-specific details like a username. This can be implemented using the renderPageCallback
option in the PSPDFKit.load()
method, allowing you to draw watermarks on each page with the Canvas API. Here’s an example:
PSPDFKit.load({ document: document, renderPageCallback: function (ctx, pageIndex, pageSize) { ctx.beginPath(); ctx.moveTo(0, 0); ctx.lineTo(pageSize.width, pageSize.height); ctx.stroke();
ctx.font = '30px Comic Sans MS'; ctx.fillStyle = 'red'; ctx.textAlign = 'center';
ctx.fillText( `Generated for John Doe. Page ${pageIndex + 1}`, pageSize.width / 2, pageSize.height / 2, ); },});
This watermark is visible only when viewing the PDF in the browser and doesn’t modify the original document.
With Nutrient Web SDK, developers can fully customize watermark text, position, and styling to align with their branding requirements.
Inserting digital signatures in a PDF with Nutrient Web SDK
Nutrient Web SDK allows you to digitally sign PDF documents using a private key and certificate. This ensures document authenticity and prevents tampering. The following example demonstrates how to integrate digital signatures using Nutrient and the signDocument
method. It fetches the private key and certificate, creates a PKCS#7
signature, and signs the document securely:
<!DOCTYPE html><html lang="en-US"> <head> <meta charset="UTF-8" /> <title>Demo</title> <style> .pdfdiv { width: 100vw; height: 100vh; } </style> </head>
<body> <div class="pdfdiv"></div> <script src="pspdfkit/dist/pspdfkit.js"></script> <script> var instance = PSPDFKit.load({ document: 'sample.pdf', container: '.pdfdiv', }) .then((instance) => { console.log('Successfully mounted PSPDFKit', instance); instance .signDocument(null, generatePKCS7) .then(() => { console.log('document signed.'); }) .catch((error) => { console.error( 'The document could not be signed.', error, ); }); }) .catch((error) => { console.error(error.message); });
function generatePKCS7({ fileContents }) { const certificatePromise = fetch( 'cert.pem', ).then((response) => response.text()); const privateKeyPromise = fetch( 'private-key.pem', ).then((response) => response.text()); return new Promise((resolve, reject) => { Promise.all([certificatePromise, privateKeyPromise]) .then(([certificatePem, privateKeyPem]) => { const certificate = forge.pki.certificateFromPem( certificatePem, ); const privateKey = forge.pki.privateKeyFromPem( privateKeyPem, );
const p7 = forge.pkcs7.createSignedData(); p7.content = new forge.util.ByteBuffer( fileContents, ); p7.addCertificate(certificate); p7.addSigner({ key: privateKey, certificate: certificate, digestAlgorithm: forge.pki.oids.sha256, authenticatedAttributes: [ { type: forge.pki.oids.contentType, value: forge.pki.oids.data, }, { type: forge.pki.oids.messageDigest, }, { type: forge.pki.oids.signingTime, value: new Date(), }, ], });
p7.sign({ detached: true }); const result = stringToArrayBuffer( forge.asn1.toDer(p7.toAsn1()).getBytes(), ); resolve(result); }) .catch(reject); }); }
// https://developers.google.com/web/updates/2012/06/How-to-convert-ArrayBuffer-to-and-from-String function stringToArrayBuffer(binaryString) { const buffer = new ArrayBuffer(binaryString.length); let bufferView = new Uint8Array(buffer); for (let i = 0, len = binaryString.length; i < len; i++) { bufferView[i] = binaryString.charCodeAt(i); } return buffer; } </script> </body></html>
This implementation ensures documents are signed with a PKCS#7 certificate, making them verifiable and secure for legal and enterprise use cases.
Check out our blog post on how to insert a digital signature in a PDF using JavaScript for more details.
Limitations of PDF.js
While PDF.js serves well as a lightweight PDF viewer, it lacks many features essential for interactive document handling:
- Annotation limitations — No built-in support for advanced annotations, page manipulation, redaction, or eSignatures. Lacks pinch-to-zoom support for mobile devices.
- Performance bottlenecks — Entirely client-side rendering can slow down large or complex PDFs.
- Third-party dependence — Requires external applications to handle MS Office documents, TXT files, and images.
- Selection inconsistencies — Text selection is unreliable due to missing or incorrect bounding boxes, causing spacing issues and missing words.
- Compliance challenges — Struggles with compliance for accessibility standards like 508/ADA due to inconsistent text extraction.
- Security concerns — No enterprise-grade security features such as access controls or encryption.
- Quality concerns — May produce blurry output and inaccurate printing, with issues in color fidelity when rendering on
<canvas>
. - Minimal customization — UI customization is limited and often requires additional development work.
- Update disruptions — Frequent open source updates can break custom implementations, requiring constant monitoring and support.
PDF.js vs. Nutrient Web SDK: Feature comparison
Feature | Nutrient Web SDK ✅ | PDF.js ⚠️ |
---|---|---|
Render/view PDFs | ||
Search text | ||
Select/copy text | ||
Advanced annotations and editing | ||
Draw lines and mark up | ||
Content editing (modify text and pages) | ||
eSignatures and digital signatures | ||
Instant synchronization and comments | ||
Redaction (remove sensitive data) | ||
Measurement tools | ||
Document conversion (PDF to Word, Excel, images, etc.) | ||
PDF generation | ||
Office file support (Word, Excel, PowerPoint) | ||
Image documents (PNG, JPEG, TIFF, etc.) | ||
PDF/A conversion (archiving standard) | ||
Page thumbnails | ||
Rotate pages | ||
Open/print/download PDFs | ||
High-quality rendering | <canvas> | |
Performance optimization | ||
Security and encryption | ||
Compliance (508/ADA accessibility standards) | ||
UI customization | ||
Stability and updates |
Pricing and licensing
When selecting a PDF solution, licensing and cost are critical factors. PDF.js and Nutrient Web SDK follow different pricing models, each catering to different use cases and business requirements.
PDF.js licensing and cost
PDF.js licensing:
✅ Open source library available under the Apache License 2.0(opens in a new tab) and free to use.
⚠️ No dedicated customer support — reliant on community contributions.
⚠️ Limited built-in functionality — annotations, form handling, and security features require additional development.
📌 Bottom line: PDF.js is a good option for lightweight applications but may require extra development effort for advanced document handling.
Nutrient Web SDK licensing and cost
Nutrient Web SDK licensing:
✅ Commercial license with structured pricing based on users and components.
✅ Includes enterprise-level features like real-time collaboration, annotations, and digital signatures.
✅ Dedicated technical support and frequent updates.
📌 Bottom line: Nutrient Web SDK provides a scalable and professional PDF solution, making it ideal for businesses that require advanced functionality, security, and long-term support.
Choosing the right tool for your needs
Looking for a PDF solution that does more than just rendering? Nutrient Web SDK is built for serious applications — annotations, editing, digital signatures, and enterprise security, all in one package.
📌 Try Nutrient Web SDK today and experience the difference. 👉 Request a free trial or talk to our experts.