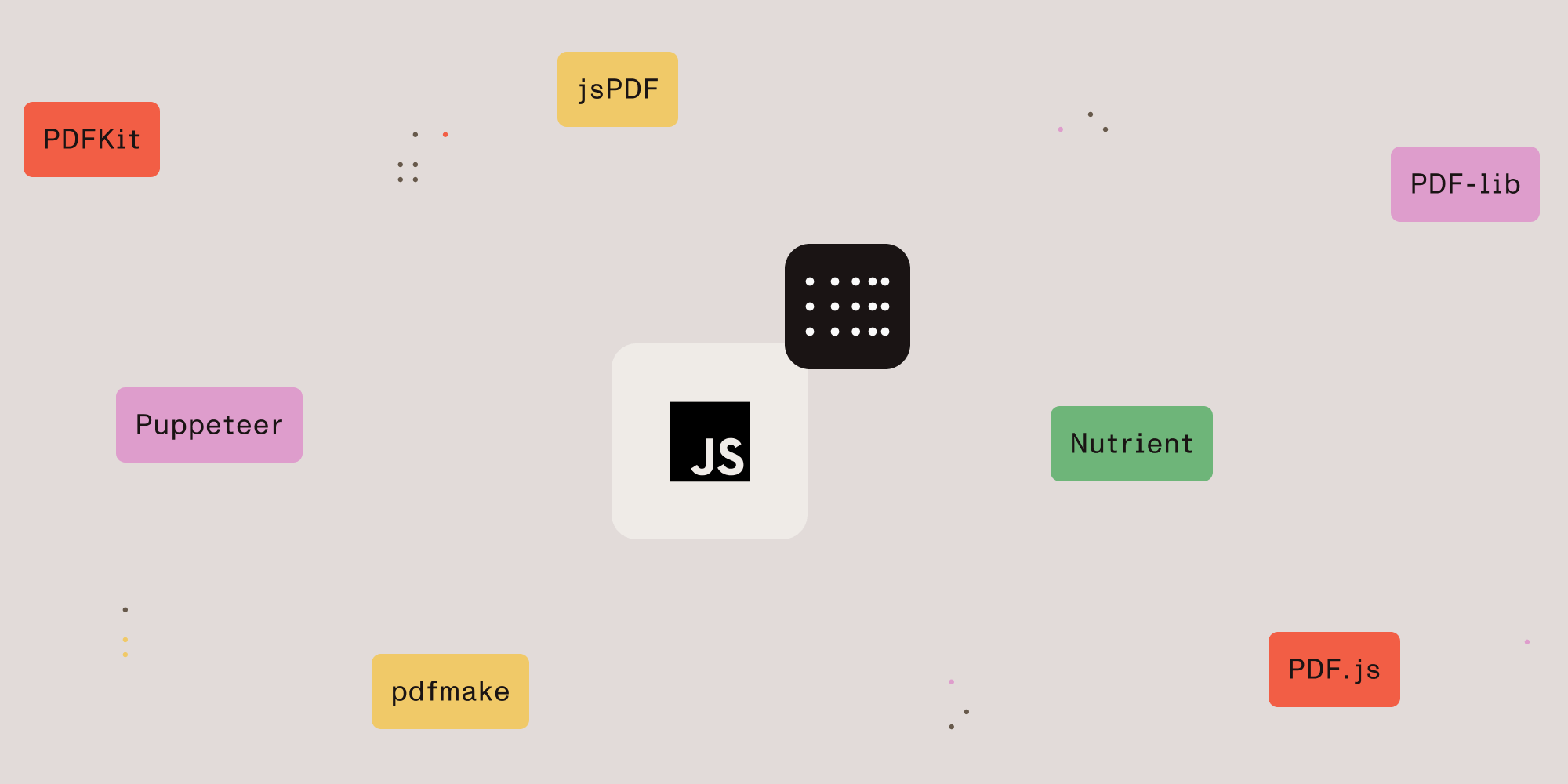
Working with PDFs in web development can be tricky, but luckily, there are several JavaScript libraries available that make generating and manipulating PDFs much easier.
In this post, we’ll explore the top JavaScript PDF libraries and provide a comparison based on their features, ease of use, and performance.
1. PDFKit
PDFKit is a popular and robust library for generating PDF documents in Node.js. It allows you to create multipage documents and add text, images, and other elements. It also supports embedding fonts. While it’s primarily server-side, it can also be used in the browser through Browserify.
Key features
-
Generates PDFs from scratch
-
Supports adding images, shapes, and custom fonts
-
Streams output to a file, HTTP response, or byte buffer
-
Works with both the server (Node.js) and browser
Use case
Ideal for generating PDFs from custom content — such as invoices, reports, and tickets — especially in Node.js applications.
Getting started with PDFKit
-
Initialize a new project and create an entry file (e.g.
app.js
):
mkdir my-pdfkit-app cd my-pdfkit-app npm init -y touch app.js
-
Install PDFKit via
npm
:
npm install pdfkit
-
In your
app.js
file, add the following code to create a basic PDF document:
const PDFDocument = require('pdfkit'); const fs = require('fs'); const doc = new PDFDocument(); doc.pipe(fs.createWriteStream('output.pdf')); doc.text('Hello, PDFKit!'); doc.end();
-
Run the script using Node.js to generate your PDF:
node app.js
Check your directory for the output.pdf
file!
When to use PDFKit
Use PDFKit when you need to generate PDFs from scratch on the server, especially when building custom PDFs like invoices, reports, or tickets. It’s ideal for applications that need server-side PDF generation and manipulation.
To learn more about PDFKit, check out the following blog posts:
- Python HTML to PDF: Convert HTML to PDF using wkhtmltopdf
- Generate PDF invoices with PDFKit in Node.js
2. jsPDF
jsPDF is a client-side PDF generation library that works directly in the browser. It’s a lightweight option, great for generating simple PDFs from HTML content, and it offers functionality like text, images, and annotations.
Key features
-
Simple API for generating PDFs in the browser
-
Supports text, images, and shapes
-
Can generate PDFs from HTML content
-
Provides plugin support for enhanced features (like HTML2PDF)
Use case
Best suited for client-side applications where you need to generate PDFs dynamically, such as form submissions, reports, or downloadable content.
Getting started with jsPDF
-
Install jsPDF via npm:
npm install jspdf
-
Add a basic HTML file to use
jsPDF
in the browser:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>jsPDF Example</title> </head> <body> <button id="generate-pdf">Generate PDF</button> <script src="node_modules/jspdf/dist/jspdf.umd.min.js"></script> <script> const { jsPDF } = window.jspdf; document .getElementById('generate-pdf') .addEventListener('click', function () { const doc = new jsPDF(); doc.text('Hello, jsPDF!', 10, 10); doc.save('output.pdf'); }); </script> </body> </html>
-
Open the HTML file in a browser, and when you click the Generate PDF button, it’ll create and download a PDF.
When to use jsPDF
jsPDF is best suited for client-side applications where you need to generate PDFs dynamically from HTML content, such as form submissions or downloadable reports.
To learn more about jsPDF, check out the following blog posts:
- How to convert HTML to PDF in React
- Generate PDFs in Salesforce with Lightning web components
- Generate a PDF from HTML with Vue.js
- How to use jsPDF and Angular to generate PDFs
- How to export to PDF using React
3. PDF-lib
PDF-lib is a powerful library that allows you to create, modify, and fill PDF documents in both the browser and Node.js. It’s well-suited for manipulating existing PDFs, such as filling forms or merging documents.
Key features
-
Create and modify PDFs
-
Supports filling out forms and embedding images
-
Works on both the client side and server side
-
No external dependencies, fully self-contained
Use case
Great for applications where you need to manipulate existing PDF files, such as filling form fields or adding annotations.
Getting started with PDF-lib
-
Initialize a new project and create an entry file:
mkdir my-pdf-lib-app cd my-pdf-lib-app npm init -y touch app.js
-
Install the library via
npm
:
npm install pdf-lib
-
In
app.js
, add code to generate and manipulate a PDF:
const { PDFDocument, rgb } = require('pdf-lib'); const fs = require('fs'); async function createPDF() { const pdfDoc = await PDFDocument.create(); const page = pdfDoc.addPage([600, 400]); page.drawText('Hello, PDF-lib!', { x: 200, y: 300, size: 30, color: rgb(0, 0, 1), }); const pdfBytes = await pdfDoc.save(); fs.writeFileSync('output.pdf', pdfBytes); } createPDF();
-
Run your script to generate the PDF:
node app.js
You’ll now have output.pdf
in your project directory.
When to use PDF-lib
PDF-lib is perfect for applications that need to manipulate existing PDF files, such as when filling out form fields, adding annotations, or merging multiple PDFs. It works well for both client-side and server-side PDF handling.
To learn more about PDF-lib, check out the following blog posts:
- How to build a JavaScript PDF editor with pdf-lib
- How to add annotations to PDF using Vue.js
- How to build a Node.js PDF editor with pdf-lib
- How to use JavaScript to capture signatures in PDFs
- How to convert images to PDF in Node.js
- How to fill a PDF form in React
- How to fill PDF forms in Node.js
- How to programmatically create and fill PDF forms in Angular
4. pdfmake
pdfmake is a high-level PDF generation library that uses a declarative approach for defining the content of a PDF. It’s easy to use and supports features like tables, lists, and images. It works for client-side and server-side PDF handling.
Key features
-
Declarative content definition
-
Supports text styling, tables, and images
-
Works in the browser and Node.js
-
Well-documented, with a large community
Use case
Perfect for generating PDFs with structured content, such as reports or catalogs, where layout and design are crucial.
Getting started with pdfmake
-
Install pdfmake via
npm
:
npm install pdfmake
-
Use
pdfmake
in the browser with the following HTML file:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>pdfmake Example</title> </head> <body> <button id="generate-pdf">Generate PDF</button> <script src="node_modules/pdfmake/build/pdfmake.js"></script> <script src="node_modules/pdfmake/build/vfs_fonts.js"></script> <script> document .getElementById('generate-pdf') .addEventListener('click', function () { const docDefinition = { content: 'Hello, pdfmake!' }; pdfMake.createPdf(docDefinition).download('output.pdf'); }); </script> </body> </html>
-
Open this HTML file in your browser, and click the Generate PDF button to generate and download a PDF.
When to use pdfmake
pdfmake is best suited for applications that require structured content in PDFs, such as reports with tables and images.
Check out the following blog post to learn more about how to use pdfmake:
5. PDF.js
PDF.js is primarily a PDF viewer, but it also offers tools for parsing and rendering PDF documents. It’s maintained by Mozilla and can be used to display PDF files in the browser. While not a generation tool, it’s invaluable for PDF display and manipulation on the client side.
Key features
-
Client-side PDF rendering
-
Supports parsing and rendering PDF documents
-
Works with HTML5 canvas for rendering
-
Maintained by Mozilla
Use case
Ideal for web applications that need to display or manipulate existing PDFs, such as e-readers or embedded document viewers.
Getting started with PDF.js
-
Download a recent copy of PDF.js from mozilla.github.io. Extract all the files in the downloaded copy of PDF.js.
-
Move
pdf.mjs
andpdf.worker.mjs
from thebuild/
folder of the download to a new empty folder. -
Use the following HTML file to display a PDF in the browser:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>PDF.js Example</title> </head> <body> <canvas id="pdf-canvas"></canvas> <script src="/pdf.mjs" type="module"></script> <script type="module"> import { GlobalWorkerOptions } from 'https://cdn.jsdelivr.net/npm/[email protected]/build/pdf.min.mjs'; GlobalWorkerOptions.workerSrc = 'https://cdn.jsdelivr.net/npm/[email protected]/build/pdf.worker.min.mjs'; const url = 'output.pdf'; // Replace with your PDF URL const canvas = document.getElementById('pdf-canvas'); const ctx = canvas.getContext('2d'); pdfjsLib .getDocument(url) .promise.then((pdf) => { pdf.getPage(1).then((page) => { const viewport = page.getViewport({ scale: 1.5 }); canvas.height = viewport.height; canvas.width = viewport.width; page.render({ canvasContext: ctx, viewport: viewport, }); }); }) .catch((error) => { console.error('Error loading PDF:', error); }); </script> </body> </html>
-
Open the HTML file in your browser to display the PDF from the URL.
When to use PDF.js
PDF.js is ideal for rendering and viewing PDFs directly in a web application. To learn more, check out the following blog posts:
- Complete guide to PDF.js: The leading JavaScript library for PDF rendering
- How to build a JavaScript PDF viewer with PDF.js
- How to build a React PDF viewer with PDF.js
- How to build a TypeScript PDF viewer with PDF.js
- How to build a Vue.js PDF viewer with PDF.js
- How to build an Angular PDF viewer with PDF.js
- How to build an Electron PDF viewer with PDF.js
6. Puppeteer
Puppeteer is a Node.js library that provides a high-level API to control Chrome or Chromium, allowing it to generate PDFs from web pages. It’s often used for server-side rendering of PDFs from dynamic web content.
Key features
-
Can generate PDFs from webpages
-
Supports complex layouts and styles
-
Ideal for headless browser automation
Use case
Useful for server-side PDF generation from websites or web applications with complex layouts, such as generating reports from dashboards or invoices from forms.
Getting started with Puppeteer
-
Create a new directory and initialize your Node.js project:
mkdir puppeteer-pdf-app cd puppeteer-pdf-app npm init -y touch generatePDF.js
-
Puppeteer can be installed via npm. This will also install a compatible version of Chromium that Puppeteer controls:
npm install puppeteer
-
Open the
generatePDF.js
file and add the following code to generate a PDF of a web page:
const puppeteer = require('puppeteer'); async function generatePDF() { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto('https://example.com', { waitUntil: 'networkidle2', }); await page.pdf({ path: 'example.pdf', format: 'A4' }); await browser.close(); } generatePDF();
-
Run the script using Node.js:
node generatePDF.js
The script will navigate to the specified URL and generate a PDF of the webpage as example.pdf
in the project folder.
When to use Puppeteer
Puppeteer is a powerful tool for generating PDFs from dynamic web content, making it ideal for use cases like website snapshots, dynamic page rendering, or automated testing where you need a pixel-perfect PDF representation of a web page.
7. Nutrient Web SDK
Nutrient Web SDK is an enterprise-grade JavaScript library for viewing, annotating, and editing PDFs directly in the browser. It offers advanced features like real-time collaboration, form filling, and digital signing.
Key features
-
Advanced PDF editing and annotation
-
Real-time collaboration features
-
Form filling and digital signing
-
High-quality PDF rendering
Use case
Best suited for applications that require a fully featured PDF editor or viewer, with the ability to add annotations, fill forms, and collaborate on documents.
Getting started with Nutrient
-
Install the
pspdfkit
package fromnpm
. If you prefer, you can also download Nutrient Web SDK manually:
npm install pspdfkit
-
For Nutrient Web SDK to work, it’s necessary to copy the directory containing all the required library files (artifacts) to the assets folder. Use the following command to do this:
cp -R ./node_modules/pspdfkit/dist/ ./assets/
-
Make sure your
assets
directory contains thepspdfkit.js
file and apspdfkit-lib
directory with the library assets. -
Add the document you want to display to your project’s directory. You can use our demo file as an example.
-
Add an empty
<div>
element with a defined height to your HTML file where Nutrient will be mounted:
<div id="pspdfkit" style="height: 100vh;"></div>
-
Include
pspdfkit.js
in your HTML page:
<script src="assets/pspdfkit.js"></script>
-
Finally, initialize Nutrient Web SDK in JavaScript by calling
PSPDFKit.load()
:
<script> PSPDFKit.load({ container: "#pspdfkit", document: "document.pdf" // Add the path to your file here. }) .then(function(instance) { console.log("PSPDFKit loaded", instance); }) .catch(function(error) { console.error(error.message); }); </script>
You can see the full index.html
file below:
<!DOCTYPE html> <html> <head> <title>My App</title> <!-- Provide proper viewport information so that the layout works on mobile devices. --> <meta name="viewport" content="width=device-width, initial-scale=1.0, minimum-scale=1.0, maximum-scale=1.0, user-scalable=no" /> </head> <body> <!-- Element where Nutrient will be mounted. --> <div id="pspdfkit" style="height: 100vh;"></div> <script src="assets/pspdfkit.js"></script> <script> PSPDFKit.load({ container: '#pspdfkit', document: 'document.pdf', // Add the path to your document here. }) .then(function (instance) { console.log('PSPDFKit loaded', instance); }) .catch(function (error) { console.error(error.message); }); </script> </body> </html> 9. To serve the website, install the serve package: ```bash npm install --global serve
-
Serve the contents of the current directory:
serve -l 8080 .
Navigate to http://localhost:8080 to view the website.
To learn more about Nutrient Web SDK, check out the following blog posts:
- How to build a TypeScript PDF viewer with Nutrient
- How to build a Nuxt.js PDF viewer with Nutrient
- How to display a PDF in React
- How to build a Tauri PDF viewer with Nutrient
- How to display PDFs using Angular
- How to build a PowerPoint (PPT/PPTX) viewer in JavaScript
- How to open Excel (XLS and XLSX) files in the browser with JavaScript
- How to build a React.js file viewer: PDF, image, MS Office
- How to open Word (DOC and DOCX) files in the browser with JavaScript
Comparison table
Library | PDF creation | PDF modification | Client-side | Server-side | Complexity | File size |
---|---|---|---|---|---|---|
PDFKit | Yes | No | No | Yes | Medium | Medium |
jsPDF | Yes | No | Yes | No | Low | Small |
PDF-lib | Yes | Yes | Yes | Yes | Medium | Small |
pdfmake | Yes | No | Yes | Yes | Low | Medium |
PDF.js | No | Yes | Yes | No | Medium | Medium |
Puppeteer | Yes | No | No | Yes | Medium | Large |
Nutrient | Yes | Yes | Yes | Yes | Medium | Large |
Conclusion
Choosing the right JavaScript PDF library depends on your project’s needs. For server-side PDF generation, PDFKit is a great option. For client-side work, jsPDF and pdfmake offer lightweight and flexible solutions, while PDF-lib excels at modifying existing PDFs and filling out forms.
If you need advanced rendering and viewing capabilities in the browser and server, Nutrient stands out with its robust document handling features.
Explore these libraries to find the best fit for your requirements, and if you want to learn more about Nutrient, feel free to reach out to our Sales team and try our demo.
FAQ
Here are a few frequently asked questions about JavaScript PDF libraries for PDF generation and manipulation.