React pdf viewer
The fastest way to add a PDF viewer to your React app
Build powerful, customizable document experiences in your React app without the PDF.js headaches. The Nutrient React viewer SDK is a drop-in component that renders PDFs, Word files, Excel spreadsheets, and more — all with pixel-perfect fidelity and full developer control. Whether you’re building a document portal, digital signature flow, or AI-powered review tool, we give you the building blocks and get out of your way.
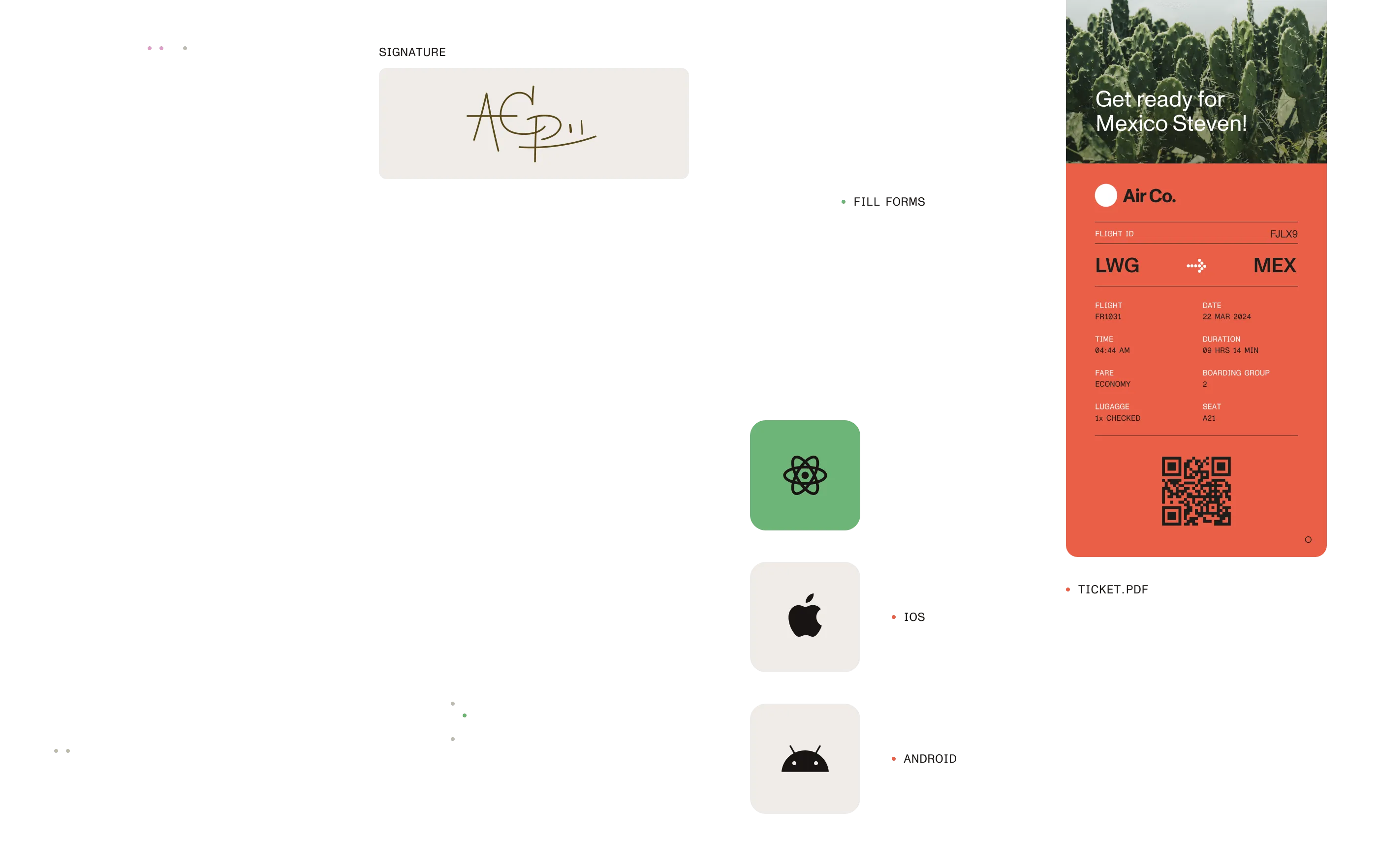
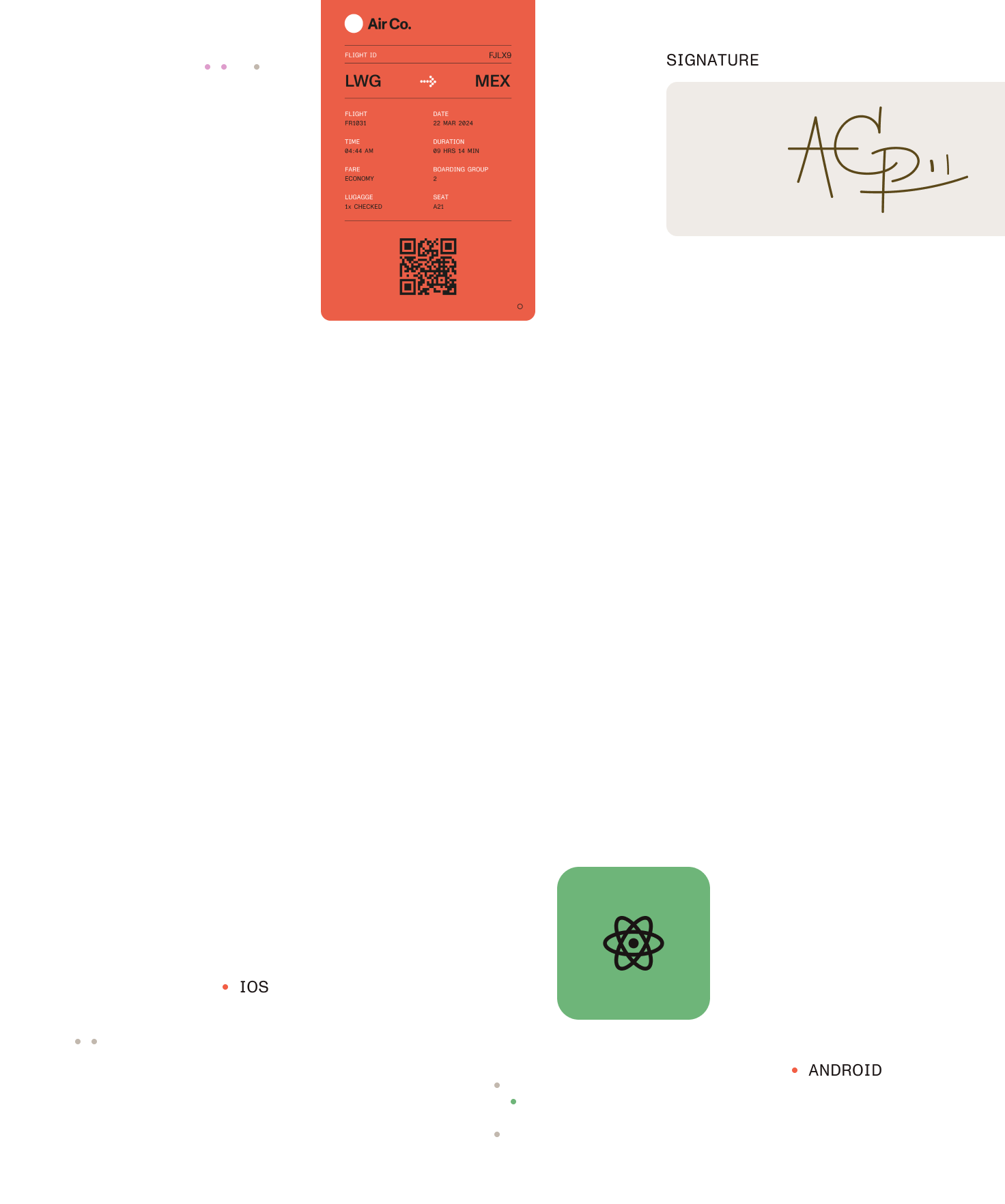
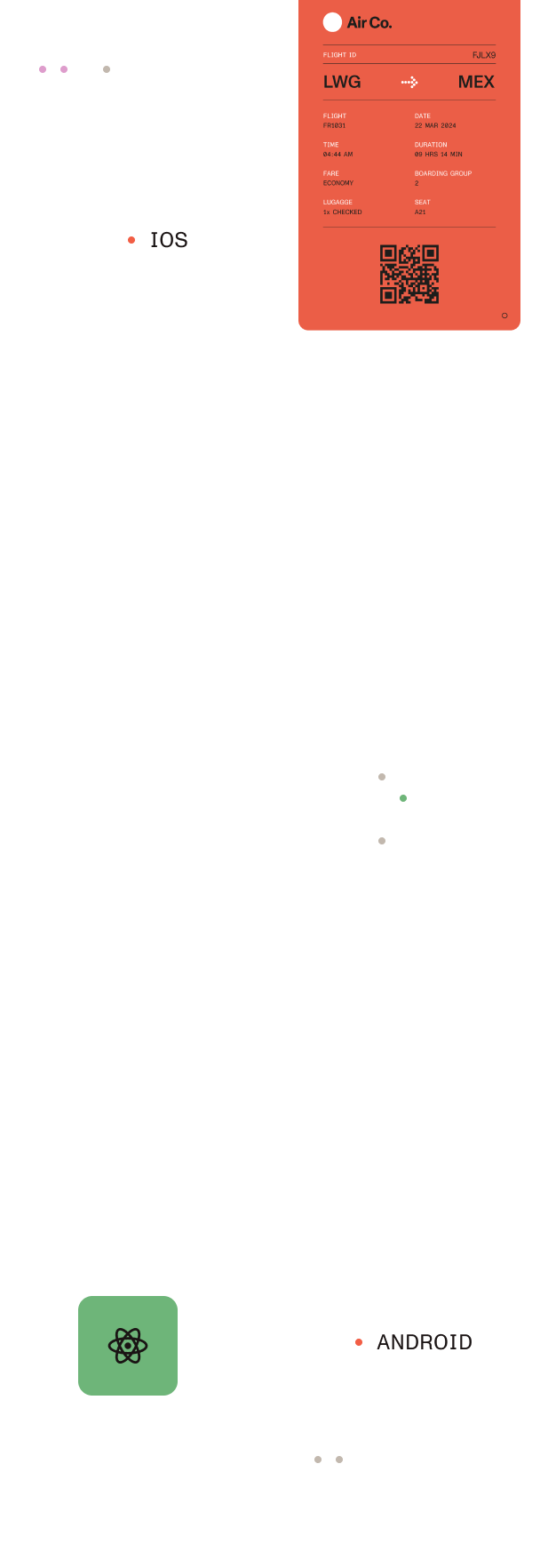
How we help
Accelerate your integration
Drop in a production-ready document viewer with just a few lines of code. Our SDK plugs seamlessly into any React project — no iframes, wrappers, or manual setup required.
Customize to fit your brand
Using a fully customizable user interface, adapt the viewer’s appearance to align seamlessly with your application’s design and branding guidelines.
Ensure cross-platform compatibility
Ensure a consistent, high-quality viewing experience across devices and browsers. Built-in responsive design makes your documents look great on desktop, tablet, or mobile.
Stream documents securely
Load files from URLs, blobs, or streamed sources — no need to expose public file links. Perfect for sensitive or compliance-bound industries like healthcare and finance.
Enhance document interactivity
Add rich PDF features like annotations, form filling, and digital signatures. Support complex workflows like redlining, approvals, or contract reviews — all inside your app.
Scale with confidence
Used by development teams building legal tech, financial portals, and document-heavy SaaS platforms. Whether you’re prototyping or scaling, the SDK supports production use from day one.
What you get
Key features
Client-side document rendering
Handle all document rendering directly in the browser — no server dependency required. Ideal for responsive, real-time interfaces where speed matters.
Accessibility ready
Ensure your document viewer works for everyone, with full compatibility for screen readers, keyboard navigation, and WCAG-compliant interfaces.
Advanced annotation and form handling
Support 17+ annotation types (highlight, text, ink, comments, etc.), advanced form creation, editing, and JavaScript-based validation.
Digital signatures
Integrate hand-drawn, typed, or certificate-based eSignatures with validation APIs. Build intuitive signing flows without external tools.
Zoom and navigation capabilities
Give users full control with zoom modes (marquee, fit-to-width), thumbnails, bookmarks, and a dynamic table of contents.
Secure sharing and control
Restrict printing, downloading, and document sharing with role-based permissions, toolbar controls, and password-protection features — all easily configured in React.
Benefits at a glance


Accelerate development
Skip weeks of low-level PDF.js setup and jump straight into building a polished viewer with customizable controls.
Improve user experience
Deliver fast, intuitive, responsive document viewing that feels like a natural part of your app — not a bolted-on afterthought.
Enable advanced workflows
Power features like document comparison, redlining, or AI assistance with our broader SDK ecosystem — all designed to work together.
Maintain full control
No black boxes. Nutrient lets you control every aspect of the viewer — from toolbar layout to event hooks and styling.
EXAMPLES
Easy to integrate
Basic example
1import NutrientViewer from "@nutrient-sdk/viewer";
2import { useEffect, useRef } from "react";
3
4function App() {
5 const containerRef = useRef(null);
6
7 useEffect(() => {
8 const container = containerRef.current;
9 NutrientViewer.load({
10 container,
11 // You can also specify a file in public directory, for example /document.pdf.
12 document: "https://www.nutrient.io/downloads/pspdfkit-web-demo.pdf",
13 });
14
15 return () => {
16 NutrientViewer?.unload(container);
17 };
18 }, []);
19
20 // You also need to set the container height and width.
21 return <div ref={containerRef} style={{ height: "100vh", width: "100vw" }} />;
22}
React PDF viewer
What are the advantages?
Integrating a PDF viewer into your React application can significantly improve document handling capabilities, offering users seamless access to view, annotate, and interact with PDF files directly within the app. This section will explore the essentials of implementing a React PDF viewer to guide you through this integration.
What is a React PDF viewer?
A React PDF viewer is a component or library that enables the rendering and interaction of PDF documents within React applications. It allows developers to embed PDF viewing functionality, providing users with features like page navigation, zooming, and text selection without leaving the application.
How to choose the right React PDF viewer
Selecting the appropriate React PDF viewer involves evaluating several key factors:
- Feature set — Ensure the viewer supports essential functionalities such as page navigation, zooming, text selection, and search capabilities.
- Customization options — Look for a viewer that allows customization of the user interface to align with your application’s design and user experience.
- Performance — Choose a viewer optimized for efficient rendering, especially for large or complex PDF documents.
- Integration ease — Opt for a solution with comprehensive documentation and support for seamless integration into your React application.
- Community and support — Consider the availability of community support and regular updates to ensure long-term reliability.
What are the best solutions to solve my React PDF viewing needs?
Various solutions are available for integrating PDF viewing capabilities into React applications:
- Basic embedding methods — Using HTML elements like <iframe> or <embed> for simple PDF viewing, though limited in functionality and customization.
- Commercial SDKs — Provide comprehensive features, dedicated support, and regular updates, ensuring reliability for enterprise-level applications.
What are the benefits of using Nutrient’s React PDF viewer?
Choosing Nutrient’s React PDF viewer offers several advantages:
- Comprehensive feature set — Includes functionalities like annotation, form filling, digital signatures, and content redaction — enhancing user interaction with documents.
- High-fidelity rendering — Utilizes a reliable rendering engine to ensure precise and accurate display of PDF documents.
- Client-side processing — Performs PDF processing on the client side, reducing server load and improving performance.
- Cross-platform compatibility — Supports various platforms and browsers, ensuring a consistent experience for all users.
How does Nutrient’s React PDF viewer compare to other solutions?
While other React PDF viewers may offer basic functionalities, Nutrient’s solution stands out with its extensive feature set, high performance, and focus on user experience. Its design prioritizes ease of use and seamless integration, making it a robust choice for applications aiming to enhance document interaction and management.
Integrating a React PDF viewer into your application is a strategic move to boost functionality and user satisfaction. By carefully evaluating your needs and exploring available options, you can select a solution that not only meets your current requirements but also supports your application’s future growth and evolution.
Connect to your tools, your way
Nutrient’s React PDF viewer seamlessly integrates with your existing tech stack, ensuring smooth interoperability with your current systems and workflows.
Frequently asked questions
How can I create a PDF viewer in React.js using react-pdf?
You can create a PDF viewer in React.js by installing the react-pdf library, importing its components, and using the document and page components to render PDF files.
What are the main components of the react-pdf library?
The main components are:
- Document — Used for loading the PDF file.
- Page — Used for rendering individual pages of the PDF.
These components leverage the PDF.js library to display PDF content.
How do I install and set up react-pdf in a React project?
Install react-pdf using npm with the following command:
npm install @react-pdf-viewer/react-pdf
Then, set up your project by:
- Importing the necessary components from the library.
- Configuring the PDF.js worker file to ensure proper rendering.
- Using the Document and Page components to display your PDF.
What are the limitations of the react-pdf library?
Some limitations of react-pdf include:
- It lacks a built-in user interface for navigation or other controls.
- Text selection can sometimes be inconsistent.
- Users need to manually build features like zoom, search, and navigation controls.
How can I enhance the functionality of a React.js PDF viewer?
You can enhance the functionality by:
- Building custom UI controls such as navigation, zoom, and search functionality.
- Integrating libraries like Nutrient to add advanced features like annotations, form filling, and enhanced user interface components.
Comparison of embedding methods
Method | Ease of use | customization | browser support | fallback option |
---|---|---|---|---|
<iframe> | Easy | Low | Excellent | |
<embed> | Easy | Low | Good | |
<object> | Moderate | Moderate | Excellent |
Get started today
Experience the capabilities of Nutrient’s React PDF viewer firsthand. We'll customize the solution to meet your specific requirements and assist you through the integration process.
.png)